Library for Adafruit OLED FeatherWing
Dependents: FTHR_OLED FTHR_SensorHub MAXREFDES101_SOURCE DisplayBMI160_Oled ... more
Fork of Adafruit_GFX by
Adafruit_SSD1306.h
00001 /********************************************************************* 00002 This is a library for our Monochrome OLEDs based on SSD1306 drivers 00003 00004 Pick one up today in the adafruit shop! 00005 ------> http://www.adafruit.com/category/63_98 00006 00007 These displays use SPI to communicate, 4 or 5 pins are required to 00008 interface 00009 00010 Adafruit invests time and resources providing this open source code, 00011 please support Adafruit and open-source hardware by purchasing 00012 products from Adafruit! 00013 00014 Written by Limor Fried/Ladyada for Adafruit Industries. 00015 BSD license, check license.txt for more information 00016 All text above, and the splash screen must be included in any redistribution 00017 *********************************************************************/ 00018 00019 /* 00020 * Modified by Neal Horman 7/14/2012 for use in mbed 00021 * Modified by Greg Steiert 2/7/2017 for FeatherOLED 00022 */ 00023 00024 #ifndef _ADAFRUIT_SSD1306_H_ 00025 #define _ADAFRUIT_SSD1306_H_ 00026 00027 #include "mbed.h" 00028 #include "Adafruit_GFX.h" 00029 00030 #include <vector> 00031 #include <algorithm> 00032 00033 #define SSD1306_EXTERNALVCC 0x1 00034 #define SSD1306_SWITCHCAPVCC 0x2 00035 00036 /** The pure base class for the SSD1306 display driver. 00037 * 00038 * You should derive from this for a new transport interface type, 00039 * such as the SPI and I2C drivers. 00040 */ 00041 class Adafruit_SSD1306 : public Adafruit_GFX 00042 { 00043 public: 00044 Adafruit_SSD1306(uint8_t rawHeight = 32, uint8_t rawWidth = 128) 00045 : Adafruit_GFX(rawWidth,rawHeight) 00046 { 00047 buffer.resize(rawHeight * rawWidth / 8); 00048 }; 00049 00050 void begin(uint8_t switchvcc = SSD1306_SWITCHCAPVCC); 00051 00052 // These must be implemented in the derived transport driver 00053 virtual void command(uint8_t c) = 0; 00054 virtual void data(uint8_t c) = 0; 00055 virtual void drawPixel(int16_t x, int16_t y, uint16_t color); 00056 00057 /// Clear the display buffer 00058 void clearDisplay(void); 00059 virtual void invertDisplay(bool i); 00060 00061 /// Cause the display to be updated with the buffer content. 00062 void display(); 00063 /// Fill the buffer with the AdaFruit splash screen. 00064 virtual void splash(); 00065 00066 protected: 00067 virtual void sendDisplayBuffer() = 0; 00068 00069 // the memory buffer for the LCD 00070 std::vector<uint8_t> buffer; 00071 }; 00072 00073 00074 /** This is the I2C SSD1306 display driver transport class 00075 * 00076 */ 00077 class Adafruit_SSD1306_I2c : public Adafruit_SSD1306 00078 { 00079 public: 00080 #define SSD_I2C_ADDRESS 0x78 00081 /** Create a SSD1306 I2C transport display driver instance with the specified RST pin name, the I2C address, as well as the display dimensions 00082 * 00083 * Required parameters 00084 * @param i2c - A reference to an initialized I2C object 00085 * @param RST - The Reset pin name 00086 * 00087 * Optional parameters 00088 * @param i2cAddress - The i2c address of the display 00089 * @param rawHeight - The vertical number of pixels for the display, defaults to 32 00090 * @param rawWidth - The horizonal number of pixels for the display, defaults to 128 00091 */ 00092 Adafruit_SSD1306_I2c(I2C &i2c, uint8_t i2cAddress = SSD_I2C_ADDRESS, uint8_t rawHeight = 32, uint8_t rawWidth = 128) 00093 : Adafruit_SSD1306(rawHeight, rawWidth) 00094 , mi2c(i2c) 00095 , mi2cAddress(i2cAddress) 00096 { 00097 begin(); 00098 splash(); 00099 display(); 00100 }; 00101 00102 virtual void command(uint8_t c) 00103 { 00104 char buff[2]; 00105 buff[0] = 0; // Command Mode 00106 buff[1] = c; 00107 mi2c.write(mi2cAddress, buff, sizeof(buff)); 00108 } 00109 00110 virtual void data(uint8_t c) 00111 { 00112 char buff[2]; 00113 buff[0] = 0x40; // Data Mode 00114 buff[1] = c; 00115 mi2c.write(mi2cAddress, buff, sizeof(buff)); 00116 }; 00117 00118 protected: 00119 virtual void sendDisplayBuffer() 00120 { 00121 char buff[17]; 00122 buff[0] = 0x40; // Data Mode 00123 00124 // send display buffer in 16 byte chunks 00125 for(uint16_t i=0, q=buffer.size(); i<q; i+=16 ) 00126 { uint8_t x ; 00127 00128 // TODO - this will segfault if buffer.size() % 16 != 0 00129 for(x=1; x<sizeof(buff); x++) 00130 buff[x] = buffer[i+x-1]; 00131 mi2c.write(mi2cAddress, buff, sizeof(buff)); 00132 } 00133 }; 00134 00135 I2C &mi2c; 00136 uint8_t mi2cAddress; 00137 }; 00138 00139 #endif
Generated on Thu Jul 14 2022 02:07:26 by
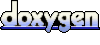