
ATT example code
Dependencies: WNCInterface mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MQTTClient.h" 00003 #include "MQTTFormat.h" 00004 #include "MQTTWNCInterface.h" 00005 #include "rtos.h" 00006 #include "k64f.h" 00007 #include "HTS221.h" 00008 #include "MODSERIAL.h" 00009 00010 I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used 00011 MODSERIAL pc(USBTX, USBRX); 00012 MODSERIAL pump(PTC15, PTC14);//TX, RX to connect pump 00013 00014 #include "hardware.h" 00015 /* 00016 #define ORG_ID "44a8w4" 00017 #define DEVICE_TYPE "IoT-Moog-1" 00018 #define DEVICE_NAME "IoT-Moog-Device1" 00019 #define USERNAME "use-token-auth" 00020 #define PASSWORD "ih*SKz2AyUSt+I*L0N" 00021 */ 00022 00023 #define ORG_ID "hpy42p" 00024 #define DEVICE_TYPE "PumpTest" 00025 #define DEVICE_NAME "SomethingOtherThanPumpTest" 00026 #define USERNAME "use-token-auth" 00027 #define PASSWORD "SLD21utaPZP7TE8Vgk" 00028 00029 #define URL ORG_ID ".messaging.internetofthings.ibmcloud.com" 00030 #define CLIENTSTR "d:" ORG_ID ":" DEVICE_TYPE ":%s" 00031 00032 #define PORT 1883 // MQTT broker port number 00033 #define PUBLISH_TOPIC "iot-2/evt/status/fmt/json" // MQTT topic 00034 #define SUBSCRIBTOPIC "iot-2/cmd/+/fmt/+" 00035 /// Largest msg possible is 111 bytes - header, 99 data bytes, CheckSum, LF/CR 00036 #define PDMS_MAX_COMMAND_SIZE 111 00037 Thread serial_thread; 00038 volatile int key_reader =0; 00039 volatile int old_Key =0; 00040 char command_str[PDMS_MAX_COMMAND_SIZE]; 00041 unsigned char AIL_Enable=0; 00042 Queue<uint32_t, 6> messageQ; 00043 00044 // LED color control function 00045 void controlLED(color_t led_color) { 00046 switch(led_color) { 00047 case red : 00048 greenLED = blueLED = 1; 00049 redLED = 0.7; 00050 break; 00051 case green : 00052 redLED = blueLED = 1; 00053 greenLED = 0.7; 00054 break; 00055 case blue : 00056 redLED = greenLED = 1; 00057 blueLED = 0.7; 00058 break; 00059 case off : 00060 redLED = greenLED = blueLED = 1; 00061 break; 00062 } 00063 } 00064 00065 // Switch 2 interrupt handler 00066 void sw2_ISR(void) { 00067 messageQ.put((uint32_t*)22); 00068 } 00069 00070 // Switch3 interrupt handler 00071 void sw3_ISR(void) { 00072 messageQ.put((uint32_t*)33); 00073 } 00074 00075 // MQTT message arrived callback function 00076 void messageArrived(MQTT::MessageData& md) { 00077 MQTT::Message &message = md.message; 00078 PRINTF("Receiving MQTT message: %.*s\r\n", message.payloadlen, (char*)message.payload); 00079 00080 if (message.payloadlen == 3) { 00081 if (strncmp((char*)message.payload, "red", 3) == 0) 00082 controlLED(red); 00083 00084 else if(strncmp((char*)message.payload, "grn", 3) == 0) 00085 controlLED(green); 00086 00087 else if(strncmp((char*)message.payload, "blu", 3) == 0) 00088 controlLED(blue); 00089 00090 else if(strncmp((char*)message.payload, "off", 3) == 0) 00091 controlLED(off); 00092 } 00093 } 00094 00095 void alla_serial() 00096 { 00097 printf("Enter Thread alla_serial"); 00098 while(1) 00099 { 00100 memset(command_str, 0x00,PDMS_MAX_COMMAND_SIZE); 00101 pump.gets(command_str, PDMS_MAX_COMMAND_SIZE); 00102 printf("%s", command_str); //print data received from Pump 00103 if(strstr(command_str, "Standby")) 00104 { 00105 key_reader=8; 00106 } 00107 else if(strstr(command_str, "Paused")) 00108 { 00109 if(AIL_Enable == 0) 00110 key_reader=1; 00111 else 00112 AIL_Enable--; 00113 } 00114 else if(strstr(command_str, "AIR IN LINE")) 00115 { 00116 key_reader=2; 00117 AIL_Enable=2; 00118 } 00119 else if(strstr(command_str, "Run Interval")) 00120 { 00121 key_reader=3; 00122 } 00123 else if(strstr(command_str, "Run Continous")) 00124 { 00125 key_reader=4; 00126 } 00127 else if(strstr(command_str, "DOOR OPEN")) 00128 { 00129 key_reader=5; 00130 AIL_Enable=2; 00131 } 00132 else if(strstr(command_str, "Priming")) 00133 { 00134 key_reader=6; 00135 } 00136 else if(strstr(command_str, "PUMP WAITING")) 00137 { 00138 key_reader=7; 00139 } 00140 else if(strstr(command_str, "Cleared")) 00141 { 00142 key_reader=9; 00143 } 00144 else 00145 { 00146 printf("\n %s", command_str); 00147 } 00148 } 00149 } 00150 int main() { 00151 ////////////////////////////////////////////////////// 00152 pump.baud(115200); 00153 // pump.format(8, SerialBase::Even, 1); 00154 serial_thread.start(&alla_serial); 00155 ////////////////////////////////////////////////////// 00156 00157 int rc, pSW2=0, txSel=0, good = 0; 00158 Timer tmr; 00159 char* topic = PUBLISH_TOPIC; 00160 char clientID[100], buf[100]; 00161 string st, uniqueID; 00162 00163 HTS221 hts221; 00164 00165 pc.baud(115200); 00166 rc = hts221.init(); 00167 if ( rc ) { 00168 PRINTF(BLU "HTS221 Detected (0x%02X)\n\r",rc); 00169 PRINTF(" Temp is: %0.2f F \r\n Huumid is: %02d %%\r\n\r\n", 00170 CTOF(hts221.readTemperature()), hts221.readHumidity()/10); 00171 } 00172 else { 00173 PRINTF(RED "HTS221 NOT DETECTED!\n\r"); 00174 } 00175 00176 controlLED(green); 00177 00178 // set SW2 and SW3 to generate interrupt on falling edge 00179 switch2.fall(&sw2_ISR); 00180 switch3.fall(&sw3_ISR); 00181 00182 // initialize ethernet interface 00183 MQTTwnc ipstack = MQTTwnc(); 00184 00185 // get and display client network info 00186 WNCInterface& eth = ipstack.getEth(); 00187 00188 // construct the MQTT client 00189 MQTT::Client<MQTTwnc, Countdown> client = MQTT::Client<MQTTwnc, Countdown>(ipstack); 00190 00191 controlLED(blue); 00192 00193 char* hostname = URL; 00194 int port = PORT; 00195 // uniqueID = "IoT-Moog-Device1"; //Guru Device 00196 uniqueID = "SomethingOtherThanPumpTest"; // Suraj Device 00197 sprintf(clientID, CLIENTSTR, uniqueID.c_str()); 00198 00199 PRINTF("Local network info...\r\n"); 00200 PRINTF("IP address is %s\r\n", eth.getIPAddress()); 00201 PRINTF("MAC address is %s\r\n", eth.getMACAddress()); 00202 PRINTF("Gateway address is %s\r\n", eth.getGateway()); 00203 PRINTF("Your <uniqueID> is: %s\r\n", uniqueID.c_str()); 00204 PRINTF("---------------------------------------------------------------\r\n"); 00205 00206 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00207 00208 int tries; 00209 00210 while( !good ) { 00211 00212 tries=0; 00213 // connect to TCP socket and check return code 00214 tmr.start(); 00215 rc = 1; 00216 while( rc && tries < 3) { 00217 PRINTF("\r\n\r\n(%d) Attempting TCP connect to %s:%d: ", tries++, hostname, port); 00218 rc = ipstack.connect(hostname, port); 00219 if( rc ) { 00220 PRINTF("Failed (%d)!\r\n",rc); 00221 while( tmr.read_ms() < 5000 ) ; 00222 tmr.reset(); 00223 } 00224 else { 00225 PRINTF("Success!\r\n"); 00226 rc = 0; 00227 } 00228 } 00229 if( tries < 3 ) 00230 tries = 0; 00231 else 00232 continue; 00233 00234 data.willFlag = 0; 00235 data.MQTTVersion = 3; 00236 00237 data.clientID.cstring = clientID; 00238 data.username.cstring = USERNAME; 00239 data.password.cstring = PASSWORD; 00240 data.keepAliveInterval = 10; 00241 data.cleansession = 1; 00242 00243 rc = 1; 00244 tmr.reset(); 00245 while( !client.isConnected() && rc && tries < 3) { 00246 PRINTF("(%d) Attempting MQTT connect to '%s': ", tries++, clientID); 00247 rc = client.connect(data); 00248 if( rc ) { 00249 PRINTF("Failed (%d)!\r\n",rc); 00250 while( tmr.read_ms() < 5000 ); 00251 tmr.reset(); 00252 } 00253 else 00254 PRINTF("Success!\r\n"); 00255 } 00256 00257 if( tries < 3 ) 00258 tries = 0; 00259 else 00260 continue; 00261 00262 // subscribe to MQTT topic 00263 tmr.reset(); 00264 rc = 1; 00265 while( rc && client.isConnected() && tries < 3) { 00266 PRINTF("(%d) Attempting to subscribing to MQTT topic '%s': ", tries, SUBSCRIBTOPIC); 00267 rc = client.subscribe(SUBSCRIBTOPIC, MQTT::QOS0, messageArrived); 00268 if( rc ) { 00269 PRINTF("Failed (%d)!\r\n", rc); 00270 while( tmr.read_ms() < 5000 ); 00271 tries++; 00272 tmr.reset(); 00273 } 00274 else { 00275 good=1; 00276 PRINTF("Subscribe successful!\r\n"); 00277 } 00278 } 00279 while (!good); 00280 } 00281 00282 MQTT::Message message; 00283 message.qos = MQTT::QOS0; 00284 message.retained = false; 00285 message.dup = false; 00286 message.payload = (void*)buf; 00287 00288 while(true) 00289 { 00290 osEvent switchEvent = messageQ.get(100); 00291 if(key_reader != old_Key) 00292 { 00293 old_Key = key_reader; 00294 memset(buf,0x00,sizeof(buf)); 00295 //sprintf(buf, "{\"d\" : {\"Key\" : %d }}", key_reader); 00296 sprintf(buf, "{\"Key\":\"%d\"}", key_reader); 00297 PRINTF("Publishing MQTT message '%s' ", (char*)message.payload); 00298 message.payloadlen = strlen(buf); 00299 PRINTF("\n payloadlen:(%d)\r\n",message.payloadlen); 00300 rc = client.publish(topic, message); 00301 if( rc ) 00302 { 00303 PRINTF("Publish request failed! (%d)\r\n",rc); 00304 FATAL_WNC_ERROR(resume); 00305 } 00306 } 00307 else 00308 { 00309 client.yield(1000); 00310 } 00311 } 00312 }
Generated on Sat Jul 16 2022 01:21:13 by
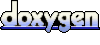