
BLE mouse with uBit ( still some issues to resolve )
Dependencies: BLE_API microbit_ble_mouse mbed nRF51822
Fork of microbit_mouse_BLE by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ble/BLE.h" 00019 #include "HIDServiceBase.h" 00020 //#include "KeyboardService.h" 00021 #include "MouseService.h" 00022 #include "examples_common.h" 00023 #include "MMA8652.h" // accelerometer 00024 //Serial pc(USBTX, USBRX); 00025 /** 00026 * This program implements a complete //////////////////////////HID-over-Gatt Profile: 00027 * - //////HID is provided by MouseService 00028 * - Battery Service 00029 * - Device Information Service 00030 */ 00031 00032 //The micro:bit has a matrixed display, this is a simple way to use some LEDs on it 00033 // turn off LED saves 1 mA current 00034 00035 DigitalOut col9(P0_12, 0); 00036 //DigitalOut waiting_led(P0_13); 00037 //DigitalOut connected_led(P0_15); 00038 00039 MMA8652 accelerometer(P0_20, P0_19); // i2c pin assign 00040 //I2C i2c(P0_19, P0_20); 00041 //const int accel_addr = 0x3A; 00042 00043 ButtonState click; 00044 MouseButton LR; 00045 00046 int Xpos = 0;int Ypos = 0; 00047 int OldXpos = 0; int OldYpos = 0; 00048 int dX = 0; int dY = 0; 00049 00050 float accelerometer_data[3]; 00051 float x,y,z; 00052 Ticker movement,timeout ; 00053 00054 00055 InterruptIn button1(BUTTON_A); 00056 InterruptIn button2(BUTTON_B); 00057 00058 BLE ble; 00059 //KeyboardService *kbdServicePtr; 00060 //MouseService musServicePtr(ble); 00061 MouseService *musServicePtr; 00062 static const char DEVICE_NAME[] = "micro:bit_BLE_Mouse"; 00063 static const char SHORT_DEVICE_NAME[] = "uBit-mUs"; 00064 00065 static void onDisconnect(const Gap::DisconnectionCallbackParams_t *params) 00066 { 00067 //////////HID_DEBUG("disconnected\r\n"); 00068 // connected_led = 0; 00069 ble.gap().startAdvertising(); // restart advertising 00070 } 00071 00072 static void onConnect(const Gap::ConnectionCallbackParams_t *params) 00073 { 00074 ////HID_DEBUG("connected\r\n"); 00075 // waiting_led = false; 00076 } 00077 00078 static void waiting() 00079 { 00080 // if (!musServicePtr->isConnected()) 00081 {}// waiting_led = !waiting_led; 00082 // else 00083 {}// connected_led = !connected_led; 00084 } 00085 00086 00087 void stop_mouse_move(void) 00088 { 00089 // Set mouse state to immobile 00090 // musServicePtr->.setButton(MOUSE_BUTTON_LEFT, MOUSE_UP); 00091 if (!musServicePtr) 00092 return; 00093 if (!musServicePtr->isConnected()) 00094 { 00095 //////HID_DEBUG("we haven't connected yet...\n"); 00096 } else { 00097 musServicePtr->setSpeed(0, 0, 0); 00098 } 00099 } 00100 00101 void start_mouse_move(void) 00102 { 00103 // Move left with a left button down. If the focus is on a drawing 00104 // software, for instance, this should draw a line. 00105 // mouse.setButton(MOUSE_BUTTON_LEFT, MOUSE_DOWN); 00106 musServicePtr->setSpeed(0, 0, 0); 00107 // timeout.attach(stop_mouse_move, 0.2); 00108 } 00109 00110 00111 void register_Lclick() 00112 { 00113 // send click left 00114 if (!musServicePtr) 00115 return; 00116 if (!musServicePtr->isConnected()) 00117 { 00118 //////HID_DEBUG("we haven't connected yet...\n"); 00119 } else { 00120 00121 LR = MOUSE_BUTTON_LEFT; 00122 click = BUTTON_DOWN; 00123 musServicePtr->setButton(LR,click); 00124 } 00125 } 00126 00127 void register_Rclick() 00128 { 00129 // send click rightLR = MOUSE_BUTTON_LEFT; 00130 if (!musServicePtr) 00131 return; 00132 if (!musServicePtr->isConnected()) 00133 { 00134 //////HID_DEBUG("we haven't connected yet...\n"); 00135 } else { 00136 00137 LR = MOUSE_BUTTON_RIGHT; 00138 click = BUTTON_DOWN; 00139 musServicePtr->setButton(LR,click); 00140 } 00141 } 00142 00143 void register_Lclick_release() 00144 { 00145 // send click left_release 00146 if (!musServicePtr) 00147 return; 00148 if (!musServicePtr->isConnected()) 00149 { 00150 //////HID_DEBUG("we haven't connected yet...\n"); 00151 } else { 00152 00153 LR = MOUSE_BUTTON_LEFT; 00154 click = BUTTON_UP; 00155 musServicePtr->setButton(LR,click); 00156 } 00157 } 00158 00159 void register_Rclick_release() 00160 { 00161 // send click right_release 00162 if (!musServicePtr) 00163 return; 00164 if (!musServicePtr->isConnected()) 00165 { 00166 //////HID_DEBUG("we haven't connected yet...\n"); 00167 } else { 00168 LR = MOUSE_BUTTON_RIGHT; 00169 click = BUTTON_UP; 00170 musServicePtr->setButton(LR,click); 00171 } 00172 } 00173 00174 void stop_mouse_movement() 00175 { 00176 musServicePtr->setSpeed(0, 0, 0); 00177 } 00178 00179 void register_mouse_movement() 00180 { 00181 if (!musServicePtr) 00182 return; 00183 if (!musServicePtr->isConnected()) 00184 { 00185 //////HID_DEBUG("we haven't connected yet...\n"); 00186 } else { 00187 00188 accelerometer.ReadXYZ(accelerometer_data); 00189 x = accelerometer_data[0]; 00190 y = accelerometer_data[1]; 00191 z = accelerometer_data[2]; 00192 // do some math to adjust movement 00193 00194 Xpos = x*50.0; 00195 Ypos = y*50.0; // float to int conv 00196 dX = abs(Xpos - OldXpos); dY = abs(Ypos - OldYpos); 00197 OldXpos = Xpos; OldYpos = Ypos; 00198 musServicePtr->setSpeed(dX, dY, 0); 00199 dX=0;dY=0; 00200 timeout.attach(stop_mouse_movement, 0.001); 00201 } 00202 } 00203 00204 int main() 00205 { 00206 Ticker heartbeat; 00207 00208 button1.rise(register_Lclick); 00209 button2.rise(register_Rclick); 00210 00211 button1.fall(register_Lclick_release); 00212 button2.fall(register_Rclick_release); 00213 00214 heartbeat.attach(waiting, 1); 00215 00216 ble.init(); 00217 ble.gap().onDisconnection(onDisconnect); 00218 ble.gap().onConnection(onConnect); 00219 initializeSecurity(ble); 00220 initializeHOGP(ble); 00221 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MOUSE); 00222 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00223 (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00224 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00225 (uint8_t *)SHORT_DEVICE_NAME, sizeof(SHORT_DEVICE_NAME)); 00226 ble.gap().setDeviceName((const uint8_t *)DEVICE_NAME); 00227 ble.gap().startAdvertising(); 00228 00229 wait(10); 00230 // movement.attach(®ister_mouse_movement, 0.05); 00231 00232 while (true) 00233 { 00234 register_mouse_movement(); 00235 ble.waitForEvent(); 00236 00237 /// make the following a separate periodic ticker 00238 //accelerometer.ReadXYZ(accelerometer_data); 00239 // x = accelerometer_data[0]; 00240 // y = accelerometer_data[1]; 00241 // z = accelerometer_data[2]; 00242 00243 } 00244 } 00245
Generated on Wed Jul 13 2022 19:03:32 by
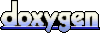