for debug
Dependencies: HTTPClient PowerControl SNICInterface mbed-rtos mbed-src
Fork of HTTPClient_WiFi_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 00003 #include "SNIC_WifiInterface.h" 00004 #include "HTTPClient.h" 00005 #if defined(TARGET_LPC1768) 00006 #include "PowerControl/EthernetPowerControl.h" 00007 #endif 00008 00009 #define DEMO_AP_SSID "Eric 5S" 00010 #define DEMO_AP_SECURITY_TYPE e_SEC_WPA2_AES 00011 #define DEMO_AP_SECUTIRY_KEY "liaoduck" 00012 00013 #if defined(TARGET_LPC1768) 00014 C_SNIC_WifiInterface wifi( p9, p10, NC, NC, p30 ); 00015 #elif defined(TARGET_NUCLEO_F401RE) || defined(TARGET_NUCLEO_L152RE) 00016 C_SNIC_WifiInterface wifi( D8, D2, NC, NC, D3); 00017 #elif defined(TARGET_K64F) 00018 C_SNIC_WifiInterface wifi( D1, D0, NC, NC, D2); 00019 #endif 00020 00021 Serial pc(USBTX, USBRX); 00022 00023 HTTPClient http; 00024 char str[512]; 00025 00026 int main() 00027 { 00028 int ret = -1; 00029 #if defined(TARGET_LPC1768) 00030 PHY_PowerDown(); 00031 #endif 00032 00033 pc.printf("WiFi init...\n"); 00034 00035 wifi.init(); 00036 00037 wait(0.5); 00038 int s = wifi.disconnect(); 00039 if( s != 0 ) { 00040 return -1; 00041 } 00042 00043 wait(0.3); 00044 // Connect AP 00045 while(ret != 0) { 00046 pc.printf("WiFi connectng...\n"); 00047 ret = wifi.connect( DEMO_AP_SSID 00048 , strlen(DEMO_AP_SSID) 00049 , DEMO_AP_SECURITY_TYPE 00050 , DEMO_AP_SECUTIRY_KEY 00051 , strlen(DEMO_AP_SECUTIRY_KEY) ); 00052 wait(1); 00053 //pc.printf("ret is %d\n", ret); 00054 }; 00055 wifi.setIPConfig( true ); //Use DHCP 00056 wait(0.5); 00057 pc.printf("IP Address is %s\n", wifi.getIPAddress()); 00058 00059 00060 #if 0 00061 // disabled this test, since the library doesn't support HTTPS connection 00062 //GET data 00063 pc.printf("\nTrying to fetch page...\n"); 00064 ret = http.get("http://developer.mbed.org/media/uploads/donatien/hello.txt", str, 128); 00065 if (!ret) { 00066 pc.printf("Page fetched successfully - read %d characters\n", strlen(str)); 00067 pc.printf("Result: %s\n", str); 00068 } else { 00069 pc.printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00070 } 00071 #endif 00072 00073 //POST data 00074 HTTPMap map; 00075 HTTPText inText(str, 512); 00076 map.put("Hello", "World"); 00077 map.put("test", "1234"); 00078 pc.printf("\nTrying to post data...\n"); 00079 ret = http.post("http://httpbin.org/post", map, &inText); 00080 if (!ret) { 00081 pc.printf("Executed POST successfully - read %d characters\n", strlen(str)); 00082 pc.printf("Result: %s\n", str); 00083 } else { 00084 pc.printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00085 } 00086 00087 //PUT data 00088 strcpy(str, "This is a PUT test!"); 00089 HTTPText outText(str); 00090 //HTTPText inText(str, 512); 00091 pc.printf("\nTrying to put resource...\n"); 00092 ret = http.put("http://httpbin.org/put", outText, &inText); 00093 if (!ret) { 00094 pc.printf("Executed PUT successfully - read %d characters\n", strlen(str)); 00095 pc.printf("Result: %s\n", str); 00096 } else { 00097 pc.printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00098 } 00099 00100 //DELETE data 00101 //HTTPText inText(str, 512); 00102 pc.printf("\nTrying to delete resource...\n"); 00103 ret = http.del("http://httpbin.org/delete", &inText); 00104 if (!ret) { 00105 pc.printf("Executed DELETE successfully - read %d characters\n", strlen(str)); 00106 pc.printf("Result: %s\n", str); 00107 } else { 00108 pc.printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00109 } 00110 00111 wifi.disconnect(); 00112 00113 while(1) { 00114 } 00115 }
Generated on Tue Jul 12 2022 19:11:41 by
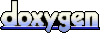