
code
Dependencies: MPU6050_SIM5320_TEST SDFileSystem WakeUp
Fork of Nucleo_L476RG_SDCard_WorkingSample by
SIM5320.cpp
00001 #include "SIM5320.h" 00002 #include <string> 00003 00004 SIM5320::SIM5320() : SIM5320Serial(PA_9, PA_10), debugSerial(USBTX, USBRX) 00005 { 00006 SIM5320Serial.baud(115200); 00007 debugSerial.baud(115200); 00008 SIM5320Serial.attach(callback(this,&SIM5320::rxInterrupt),Serial::RxIrq); 00009 flushBuffer(); 00010 rx_in=0; 00011 } 00012 00013 SIM5320::SIM5320(PinName tx, PinName rx, bool debug) : SIM5320Serial(tx, rx), debugSerial(USBTX, USBRX) 00014 { 00015 SIM5320Serial.baud(115200); 00016 debugSerial.baud(115200); 00017 SIM5320Serial.attach(callback(this,&SIM5320::rxInterrupt),Serial::RxIrq); 00018 flushBuffer(); 00019 rx_in=0; 00020 } 00021 00022 string SIM5320::sendCommand(const char *command, uint8_t timeout) 00023 { 00024 //printDebug(command); 00025 SIM5320Serial.printf("%s\r\n", command); 00026 wait(timeout); 00027 string ret = readBuffer(); 00028 const char* debugResponse = rx_buffer; 00029 printDebug(debugResponse); 00030 flushBuffer(); 00031 return ret; 00032 00033 00034 } 00035 00036 uint8_t SIM5320::sendCommandAndCheck(const char *command, const char *response, uint8_t timeout) 00037 { 00038 string answer = sendCommand(command, timeout); 00039 00040 string resp(response); 00041 if (answer.find(resp) != string::npos) { 00042 return 1; 00043 } 00044 return 0; 00045 } 00046 void SIM5320::printDebug(const char *line) 00047 { 00048 debugSerial.printf("%s\n",line); 00049 } 00050 bool SIM5320::connect(const char *apn, const char *userName, const char *passPhrase) 00051 { 00052 sendCommand("AT",2); 00053 sendCommand("AT+CSQ",2); 00054 sendCommand("AT+CREG?",2); 00055 sendCommand("AT+CPSI?",2); 00056 sendCommand("AT+CGREG?",2); 00057 sendCommand(concatChars("AT+CGSOCKCONT=1,\"IP\",\"",apn,"\""),3); 00058 sendCommand("AT+CSOCKSETPN=1",2); 00059 sendCommand("AT+CIPMODE=0",2); 00060 sendCommand("AT+NETOPEN",10); 00061 return sendCommandAndCheck("AT+IPADDR","IPADDR",3); 00062 } 00063 bool SIM5320::disconnect() 00064 { 00065 sendCommand("AT+NETCLOSE",2); 00066 return sendCommandAndCheck("AT+IPADDR","IP ERROR",2); 00067 } 00068 bool SIM5320::enableGPS(bool isEnabled) 00069 { 00070 char x='0'; 00071 if(isEnabled) { 00072 x='1'; 00073 } else { 00074 x='0'; 00075 } 00076 return sendCommandAndCheck("AT+CGPS=1","OK",3); 00077 } 00078 void SIM5320::rxInterrupt() 00079 { 00080 while(SIM5320Serial.readable()) { 00081 char c = SIM5320Serial.getc(); 00082 rx_buffer[rx_in] = c; 00083 rx_in++; 00084 if(rx_in==BUFFER_SIZE) 00085 rx_in=0; 00086 } 00087 return; 00088 } 00089 00090 string SIM5320::readBuffer() 00091 { 00092 00093 return string(rx_buffer); 00094 } 00095 00096 void SIM5320::flushBuffer() 00097 { 00098 for(int i=0; i<BUFFER_SIZE; i++) 00099 rx_buffer[i]=0; 00100 rx_in=0; 00101 } 00102 00103 char* SIM5320::concatChars(const char* in1, const char* in2, const char* in3) 00104 { 00105 char* out1 = (char *) malloc(1+strlen(in1)+strlen(in2)); 00106 char* out2 = (char *) malloc(1+strlen(out1)+strlen(in3)); 00107 strcpy(out1, in1); 00108 strcat(out1, in2); 00109 00110 strcpy(out2, out1); 00111 strcat(out2, in3); 00112 00113 return out2; 00114 00115 }
Generated on Sun Jul 24 2022 09:08:56 by
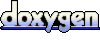