This is older repository, see HTTPClientForFIAP. 新しい Version のリポジトリーが HTTPClientForFIAP にあります。
Fork of HTTPClientForSOAP by
HTTPMap.cpp
00001 /* HTTPMap.cpp */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "HTTPMap.h" 00021 00022 #include <cstring> 00023 00024 #include <cctype> 00025 00026 #define OK 0 00027 00028 using std::strncpy; 00029 00030 HTTPMap::HTTPMap() : m_pos(0), m_count(0) 00031 { 00032 00033 } 00034 00035 void HTTPMap::put(const char* key, const char* value) 00036 { 00037 if(m_count >= HTTPMAP_TABLE_SIZE) 00038 { 00039 return; 00040 } 00041 m_keys[m_count] = key; 00042 m_values[m_count] = value; 00043 m_count++; 00044 } 00045 00046 void HTTPMap::clear() 00047 { 00048 m_count = 0; 00049 m_pos = 0; 00050 } 00051 00052 00053 /*virtual*/ int HTTPMap::read(char* buf, size_t len, size_t* pReadLen) 00054 { 00055 if(m_pos >= m_count) 00056 { 00057 *pReadLen = 0; 00058 m_pos = 0; 00059 return OK; 00060 } 00061 00062 //URL encode 00063 char* out = buf; 00064 const char* in = m_keys[m_pos]; 00065 if( (m_pos != 0) && (out - buf < len - 1) ) 00066 { 00067 *out='&'; 00068 out++; 00069 } 00070 00071 while( (*in != '\0') && (out - buf < len - 3) ) 00072 { 00073 if (std::isalnum(*in) || *in == '-' || *in == '_' || *in == '.' || *in == '~') 00074 { 00075 *out = *in; 00076 out++; 00077 } 00078 else if( *in == ' ' ) 00079 { 00080 *out='+'; 00081 out++; 00082 } 00083 else 00084 { 00085 char hex[] = "0123456789abcdef"; 00086 *out='%'; 00087 out++; 00088 *out=hex[(*in>>4)&0xf]; 00089 out++; 00090 *out=hex[(*in)&0xf]; 00091 out++; 00092 } 00093 in++; 00094 } 00095 00096 if( out - buf < len - 1 ) 00097 { 00098 *out='='; 00099 out++; 00100 } 00101 00102 in = m_values[m_pos]; 00103 while( (*in != '\0') && (out - buf < len - 3) ) 00104 { 00105 if (std::isalnum(*in) || *in == '-' || *in == '_' || *in == '.' || *in == '~') 00106 { 00107 *out = *in; 00108 out++; 00109 } 00110 else if( *in == ' ' ) 00111 { 00112 *out='+'; 00113 out++; 00114 } 00115 else 00116 { 00117 char hex[] = "0123456789abcdef"; 00118 *out='%'; 00119 out++; 00120 *out=hex[(*in>>4)&0xf]; 00121 out++; 00122 *out=hex[(*in)&0xf]; 00123 out++; 00124 } 00125 in++; 00126 } 00127 00128 *pReadLen = out - buf; 00129 00130 m_pos++; 00131 return OK; 00132 } 00133 00134 /*virtual*/ int HTTPMap::getDataType(char* type, size_t maxTypeLen) //Internet media type for Content-Type header 00135 { 00136 strncpy(type, "application/x-www-form-urlencoded", maxTypeLen-1); 00137 type[maxTypeLen-1] = '\0'; 00138 return OK; 00139 } 00140 00141 /*virtual*/ bool HTTPMap::getIsChunked() //For Transfer-Encoding header 00142 { 00143 return false; ////Data is computed one key/value pair at a time 00144 } 00145 00146 /*virtual*/ size_t HTTPMap::getDataLen() //For Content-Length header 00147 { 00148 size_t count = 0; 00149 for(size_t i = 0; i< m_count; i++) 00150 { 00151 //URL encode 00152 const char* in = m_keys[i]; 00153 if( i != 0 ) 00154 { 00155 count++; 00156 } 00157 00158 while( (*in != '\0') ) 00159 { 00160 if (std::isalnum(*in) || *in == '-' || *in == '_' || *in == '.' || *in == '~') 00161 { 00162 count++; 00163 } 00164 else if( *in == ' ' ) 00165 { 00166 count++; 00167 } 00168 else 00169 { 00170 count+=3; 00171 } 00172 in++; 00173 } 00174 00175 count ++; 00176 00177 in = m_values[i]; 00178 while( (*in != '\0') ) 00179 { 00180 if (std::isalnum(*in) || *in == '-' || *in == '_' || *in == '.' || *in == '~') 00181 { 00182 count++; 00183 } 00184 else if( *in == ' ' ) 00185 { 00186 count++; 00187 } 00188 else 00189 { 00190 count+=3; 00191 } 00192 in++; 00193 } 00194 } 00195 return count; 00196 }
Generated on Sat Jul 16 2022 18:54:06 by
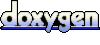