A library to read and write all 25* serial SPI flash devices from various manufacturers.
Embed:
(wiki syntax)
Show/hide line numbers
flash25spi.h
00001 /* This library is based on the Ser25lcxxx library by Hendrik Lipka 00002 * It was adapted to flash memory chips on 19.2.2011 by Klaus Steinhammer 00003 * the BSD license also applies to this code - have fun. 00004 */ 00005 00006 /* 00007 * Ser25lcxxx library 00008 * Copyright (c) 2010 Hendrik Lipka 00009 * 00010 * Permission is hereby granted, free of charge, to any person obtaining a copy 00011 * of this software and associated documentation files (the "Software"), to deal 00012 * in the Software without restriction, including without limitation the rights 00013 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00014 * copies of the Software, and to permit persons to whom the Software is 00015 * furnished to do so, subject to the following conditions: 00016 * 00017 * The above copyright notice and this permission notice shall be included in 00018 * all copies or substantial portions of the Software. 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00021 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00022 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00023 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00024 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00025 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00026 * THE SOFTWARE. 00027 */ 00028 00029 #ifndef __FLASH25SPI_H__ 00030 #define __FLASH25SPI_H__ 00031 00032 #include "mbed.h" 00033 00034 /** 00035 A class to read and write all 25* serial SPI flash devices from various manufacturers. 00036 It tries to autodetect the connected device. If your flash is not in the device list, but 00037 it compatible, feel free to modify the library and add it to the list. 00038 */ 00039 00040 class flash25spi 00041 { 00042 public: 00043 /** 00044 create the handler class. it tries to autodetect your device. If your device is not recognized, add the devices parameters 00045 to the device data structure at the library sources. 00046 @param spi the SPI port where the flash is connected. Must be set to format(8,3), and with a speed matching the one of your device 00047 @param enable the pin name for the port where /CS is connected 00048 */ 00049 flash25spi(SPI *spi, PinName enable); 00050 00051 /** 00052 destroys the handler, and frees the /CS pin 00053 */ 00054 ~flash25spi(); 00055 00056 /** 00057 read a part of the flashs memory. The buffer will be allocated here, and must be freed by the user 00058 @param startAdr the adress where to start reading. Doesn't need to match a page boundary 00059 @param len the number of bytes to read (must not exceed the end of memory) 00060 @return NULL in case of an error or if the adresses are out of range, the pointer to the data otherwise 00061 */ 00062 char* read(unsigned int startAdr, unsigned int len); 00063 00064 /** 00065 writes the give buffer into the memory. This function handles dividing the write into 00066 pages, and waites until the phyiscal write has finished 00067 @param startAdr the adress where to start writing. Doesn't need to match a page boundary 00068 @param len the number of bytes to read (must not exceed the end of memory) 00069 @return false if the adresses are out of range 00070 */ 00071 bool write(unsigned int startAdr, unsigned int len, const char* data); 00072 00073 /** 00074 fills the sector containing the given address with 0xFF - this erases several pages. 00075 Refer to the flashes datasheet about the memory organisation. 00076 @param addr an address within the sector to be cleared 00077 */ 00078 void clearSector(unsigned int addr); 00079 00080 /** 00081 fills the block containing the given address with 0xFF - this erases several pages and several sectors. 00082 Refer to the flashes datasheet about the memory organisation. 00083 @param addr an address within the sector to be cleared 00084 */ 00085 void clearBlock(unsigned int addr); 00086 00087 00088 /** 00089 fills the whole flash with 0xFF 00090 */ 00091 void clearMem(); 00092 00093 private: 00094 bool writePage(unsigned int startAdr, unsigned int len, const char* data); 00095 int readStatus(); 00096 void waitForWrite(); 00097 void enableWrite(); 00098 00099 00100 SPI* _spi; 00101 DigitalOut* _enable; 00102 unsigned int _size,_pageSize,_sectorSize,_blockSize; 00103 00104 }; 00105 00106 00107 00108 #endif
Generated on Wed Jul 13 2022 09:50:18 by
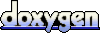