
Nucleo F411RE, SR04 and servomotor with Cayenne IoT
Dependencies: Cayenne-MQTT-mbed mbed Servo X_NUCLEO_IDW01M1v2 NetworkSocketAPI HCSR04
main.cpp
00001 /** 00002 * Example app for using the Cayenne MQTT C++ library to send and receive example data. This example uses 00003 * the X-NUCLEO-IDW01M1 WiFi expansion board via the X_NUCLEO_IDW01M1v2 library. 00004 */ 00005 00006 #include "MQTTTimer.h" 00007 #include "CayenneMQTTClient.h" 00008 #include "MQTTNetworkIDW01M1.h" 00009 #include "SpwfInterface.h" 00010 //#include "hcsr04.h" 00011 //#include "Servo.h" 00012 00013 // WiFi network info. 00014 char* ssid = "iPhone"; 00015 char* wifiPassword = "abcd1234"; 00016 00017 // Cayenne authentication info. This should be obtained from the Cayenne Dashboard. 00018 char* username = "4f3fbcb0-3796-11e9-ad96-c15442ccb423"; 00019 char* password = "9e099f3d9aaedd7b76ca94044c6bb488c3999e3c"; 00020 char* clientID = "4288d2f0-a5a9-11e9-9636-f9904f7b864b"; 00021 00022 SpwfSAInterface interface(D8, D2); // TX, RX 00023 MQTTNetwork<SpwfSAInterface> network(interface); 00024 CayenneMQTT::MQTTClient<MQTTNetwork<SpwfSAInterface>, MQTTTimer> mqttClient(network, username, password, clientID); 00025 00026 DigitalIn button1(USER_BUTTON); 00027 DigitalOut led1(LED1); 00028 DigitalOut ledGreen(D11); 00029 DigitalOut ledRed(D12); 00030 //HCSR04 sensor(D7, D6); 00031 //Servo myservo(D10); 00032 00033 int iotvalue; 00034 00035 //Function prototype new 00036 MQTTTimer publishData(MQTTTimer, int/*, int*/); 00037 MQTTTimer myFunction(MQTTTimer); 00038 00039 MQTTTimer myFunction (MQTTTimer timer){ 00040 /** 00041 * Write your codes here. 00042 **/ 00043 led1 = 1; 00044 wait(0.2); 00045 led1 = 0; 00046 wait(0.2); 00047 00048 ledGreen = iotvalue; 00049 00050 /*ULTRASONIC + SERVO///////////////////////////// 00051 int openclose; 00052 long distance = sensor.distance(); 00053 printf("distance %d \n",distance); 00054 wait(1.0); // 1 sec 00055 if (distance > 50) { 00056 ledGreen = 0; 00057 ledRed = 1; 00058 myservo = 1; //tutup 00059 wait(0.2); 00060 openclose = 0; 00061 } 00062 if (distance <50) { 00063 ledGreen = 1; 00064 ledRed = 0; 00065 myservo = 0; //buka 00066 wait(0.2); 00067 openclose = 1; 00068 } 00069 */ 00070 timer = publishData(timer, ledGreen/*, openclose*/); 00071 return timer; 00072 } 00073 00074 MQTTTimer publishData(MQTTTimer timer, int data1/*, int data2*/){ 00075 // Publish some example data every few seconds. This should be changed to send your actual data to Cayenne. 00076 if (timer.expired()) { 00077 int error = 0; 00078 if ((error = mqttClient.publishData(DATA_TOPIC, 1, TYPE_VOLTAGE, UNIT_DIGITAL, data1)) != CAYENNE_SUCCESS) { 00079 printf("Publish data failed, error: %d\n", error); 00080 } 00081 // if ((error = mqttClient.publishData(DATA_TOPIC, 2, TYPE_VOLTAGE, UNIT_DIGITAL, data2)) != CAYENNE_SUCCESS) { 00082 // printf("Publish data failed, error: %d\n", error); 00083 // } 00084 // Restart the countdown timer for publishing data every 5 seconds. Change the timeout parameter to publish at a different interval. 00085 timer.countdown_ms(1500); 00086 } 00087 return timer; 00088 } 00089 00090 /** 00091 * Print the message info. 00092 * @param[in] message The message received from the Cayenne server. 00093 */ 00094 void outputMessage(CayenneMQTT::MessageData& message) 00095 { 00096 switch (message.topic) { 00097 case COMMAND_TOPIC: 00098 printf("topic=Command"); 00099 break; 00100 case CONFIG_TOPIC: 00101 printf("topic=Config"); 00102 break; 00103 default: 00104 printf("topic=%d", message.topic); 00105 break; 00106 } 00107 printf(" channel=%d", message.channel); 00108 if (message.clientID) { 00109 printf(" clientID=%s", message.clientID); 00110 } 00111 if (message.type) { 00112 printf(" type=%s", message.type); 00113 } 00114 for (size_t i = 0; i < message.valueCount; ++i) { 00115 if (message.getValue(i)) { 00116 printf(" value=%s", message.getValue(i)); 00117 } 00118 if (message.getUnit(i)) { 00119 printf(" unit=%s", message.getUnit(i)); 00120 } 00121 } 00122 if (message.id) { 00123 printf(" id=%s", message.id); 00124 } 00125 printf("\n"); 00126 } 00127 00128 /** 00129 * Handle messages received from the Cayenne server. 00130 * @param[in] message The message received from the Cayenne server. 00131 */ 00132 void messageArrived(CayenneMQTT::MessageData& message) 00133 { 00134 int error = 0; 00135 00136 // Add code to process the message. Here we just ouput the message data. 00137 outputMessage(message); 00138 00139 if (message.topic == COMMAND_TOPIC) { 00140 switch(message.channel) { 00141 case 0: 00142 // Set the onboard LED state 00143 iotvalue = atoi(message.getValue()); 00144 printf("From Cayenne = %d\n",iotvalue); 00145 // Publish the updated LED state 00146 if ((error = mqttClient.publishData(DATA_TOPIC, message.channel, NULL, NULL, message.getValue())) != CAYENNE_SUCCESS) { 00147 printf("Publish LED state failure, error: %d\n", error); 00148 } 00149 break; 00150 } 00151 00152 // If this is a command message we publish a response. Here we are just sending a default 'OK' response. 00153 // An error response should be sent if there are issues processing the message. 00154 if ((error = mqttClient.publishResponse(message.id, NULL, message.clientID)) != CAYENNE_SUCCESS) { 00155 printf("Response failure, error: %d\n", error); 00156 } 00157 } 00158 } 00159 00160 /** 00161 * Connect to the Cayenne server. 00162 * @return Returns CAYENNE_SUCCESS if the connection succeeds, or an error code otherwise. 00163 */ 00164 int connectClient(void) 00165 { 00166 int error = 0; 00167 // Connect to the server. 00168 printf("Connecting to %s:%d\n", CAYENNE_DOMAIN, CAYENNE_PORT); 00169 while ((error = network.connect(CAYENNE_DOMAIN, CAYENNE_PORT)) != 0) { 00170 printf("TCP connect failed, error: %d\n", error); 00171 wait(2); 00172 } 00173 00174 if ((error = mqttClient.connect()) != MQTT::SUCCESS) { 00175 printf("MQTT connect failed, error: %d\n", error); 00176 return error; 00177 } 00178 printf("Connected\n"); 00179 00180 // Subscribe to required topics. 00181 if ((error = mqttClient.subscribe(COMMAND_TOPIC, CAYENNE_ALL_CHANNELS)) != CAYENNE_SUCCESS) { 00182 printf("Subscription to Command topic failed, error: %d\n", error); 00183 } 00184 if ((error = mqttClient.subscribe(CONFIG_TOPIC, CAYENNE_ALL_CHANNELS)) != CAYENNE_SUCCESS) { 00185 printf("Subscription to Config topic failed, error:%d\n", error); 00186 } 00187 00188 // Send device info. Here we just send some example values for the system info. These should be changed to use actual system data, or removed if not needed. 00189 mqttClient.publishData(SYS_VERSION_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, CAYENNE_VERSION); 00190 mqttClient.publishData(SYS_MODEL_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "mbedDevice"); 00191 //mqttClient.publishData(SYS_CPU_MODEL_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "CPU Model"); 00192 //mqttClient.publishData(SYS_CPU_SPEED_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "1000000000"); 00193 00194 return CAYENNE_SUCCESS; 00195 } 00196 00197 /** 00198 * Main loop where MQTT code is run. 00199 */ 00200 void loop(void) 00201 { 00202 // Start the countdown timer for publishing data every 5 seconds. Change the timeout parameter to publish at a different interval. 00203 MQTTTimer timer(1500); 00204 00205 while (true) { 00206 00207 // Yield to allow MQTT message processing. 00208 mqttClient.yield(1000); 00209 00210 // Check that we are still connected, if not, reconnect. 00211 if (!network.connected() || !mqttClient.connected()) { 00212 network.disconnect(); 00213 mqttClient.disconnect(); 00214 printf("Reconnecting\n"); 00215 while (connectClient() != CAYENNE_SUCCESS) { 00216 wait(2); 00217 printf("Reconnect failed, retrying\n"); 00218 } 00219 } 00220 timer = myFunction (timer); 00221 } 00222 } 00223 00224 /** 00225 * Main function. 00226 */ 00227 int main() 00228 { 00229 // Initialize the network interface. 00230 printf("Initializing interface\n"); 00231 interface.connect(ssid, wifiPassword, NSAPI_SECURITY_WPA2); 00232 00233 // Set the default function that receives Cayenne messages. 00234 mqttClient.setDefaultMessageHandler(messageArrived); 00235 00236 // Connect to Cayenne. 00237 if (connectClient() == CAYENNE_SUCCESS) { 00238 // Run main loop. 00239 loop(); 00240 } 00241 else { 00242 printf("Connection failed, exiting\n"); 00243 } 00244 00245 if (mqttClient.connected()) 00246 mqttClient.disconnect(); 00247 if (network.connected()) 00248 network.disconnect(); 00249 00250 return 0; 00251 }
Generated on Sun Jul 17 2022 04:47:42 by
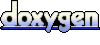