Library for big numbers from http://www.ttmath.org/
Dependents: PIDHeater82 Conceptcontroller_v_1_0 AlarmClockApp COG4050_adxl355_tilt ... more
ttmaththreads.h
00001 /* 00002 * This file is a part of TTMath Bignum Library 00003 * and is distributed under the (new) BSD licence. 00004 * Author: Tomasz Sowa <t.sowa@ttmath.org> 00005 */ 00006 00007 /* 00008 * Copyright (c) 2006-2009, Tomasz Sowa 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without 00012 * modification, are permitted provided that the following conditions are met: 00013 * 00014 * * Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 00017 * * Redistributions in binary form must reproduce the above copyright 00018 * notice, this list of conditions and the following disclaimer in the 00019 * documentation and/or other materials provided with the distribution. 00020 * 00021 * * Neither the name Tomasz Sowa nor the names of contributors to this 00022 * project may be used to endorse or promote products derived 00023 * from this software without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00028 * ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE 00029 * LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00030 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00031 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00032 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00033 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00034 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF 00035 * THE POSSIBILITY OF SUCH DAMAGE. 00036 */ 00037 00038 00039 00040 #ifndef headerfilettmaththreads 00041 #define headerfilettmaththreads 00042 00043 #include "ttmathtypes.h" 00044 00045 #ifdef TTMATH_WIN32_THREADS 00046 #include <windows.h> 00047 #include <cstdio> 00048 #endif 00049 00050 #ifdef TTMATH_POSIX_THREADS 00051 #include <pthread.h> 00052 #endif 00053 00054 00055 00056 /*! 00057 \file ttmaththreads.h 00058 \brief Some objects used in multithreads environment 00059 */ 00060 00061 00062 /* 00063 this is a simple skeleton of a program in multithreads environment: 00064 00065 #define TTMATH_MULTITHREADS 00066 #include<ttmath/ttmath.h> 00067 00068 TTMATH_MULTITHREADS_HELPER 00069 00070 int main() 00071 { 00072 [...] 00073 } 00074 00075 make sure that macro TTMATH_MULTITHREADS is defined and (somewhere in *.cpp file) 00076 use TTMATH_MULTITHREADS_HELPER macro (outside of any classes/functions/namespaces scope) 00077 */ 00078 00079 00080 namespace ttmath 00081 { 00082 00083 00084 #ifdef TTMATH_WIN32_THREADS 00085 00086 /* 00087 we use win32 threads 00088 */ 00089 00090 00091 /*! 00092 in multithreads environment you should use TTMATH_MULTITHREADS_HELPER macro 00093 somewhere in *.cpp file 00094 00095 (at the moment in win32 this macro does nothing) 00096 */ 00097 #define TTMATH_MULTITHREADS_HELPER 00098 00099 00100 /*! 00101 objects of this class are used to synchronize 00102 */ 00103 class ThreadLock 00104 { 00105 HANDLE mutex_handle; 00106 00107 00108 void CreateName(char * buffer) const 00109 { 00110 #ifdef _MSC_VER 00111 #pragma warning (disable : 4996) 00112 // warning C4996: 'sprintf': This function or variable may be unsafe. Consider using sprintf_s instead. 00113 #endif 00114 00115 sprintf(buffer, "TTMATH_LOCK_%ul", (unsigned long)GetCurrentProcessId()); 00116 00117 #ifdef _MSC_VER 00118 #pragma warning (default : 4996) 00119 #endif 00120 } 00121 00122 00123 public: 00124 00125 bool Lock() 00126 { 00127 char buffer[50]; 00128 00129 CreateName(buffer); 00130 mutex_handle = CreateMutexA(0, false, buffer); 00131 00132 if( mutex_handle == 0 ) 00133 return false; 00134 00135 WaitForSingleObject(mutex_handle, INFINITE); 00136 00137 return true; 00138 } 00139 00140 00141 ThreadLock () 00142 { 00143 mutex_handle = 0; 00144 } 00145 00146 00147 ~ThreadLock () 00148 { 00149 if( mutex_handle != 0 ) 00150 { 00151 ReleaseMutex(mutex_handle); 00152 CloseHandle(mutex_handle); 00153 } 00154 } 00155 }; 00156 00157 #endif // #ifdef TTMATH_WIN32_THREADS 00158 00159 00160 00161 00162 00163 #ifdef TTMATH_POSIX_THREADS 00164 00165 /* 00166 we use posix threads 00167 */ 00168 00169 00170 /*! 00171 in multithreads environment you should use TTMATH_MULTITHREADS_HELPER macro 00172 somewhere in *.cpp file 00173 (this macro defines a pthread_mutex_t object used by TTMath library) 00174 */ 00175 #define TTMATH_MULTITHREADS_HELPER \ 00176 namespace ttmath \ 00177 { \ 00178 pthread_mutex_t ttmath_mutex = PTHREAD_MUTEX_INITIALIZER; \ 00179 } 00180 00181 00182 /*! 00183 ttmath_mutex will be defined by TTMATH_MULTITHREADS_HELPER macro 00184 */ 00185 extern pthread_mutex_t ttmath_mutex ; 00186 00187 00188 /*! 00189 objects of this class are used to synchronize 00190 */ 00191 class ThreadLock 00192 { 00193 public: 00194 00195 bool Lock() 00196 { 00197 if( pthread_mutex_lock(&ttmath_mutex ) != 0 ) 00198 return false; 00199 00200 return true; 00201 } 00202 00203 00204 ~ThreadLock() 00205 { 00206 pthread_mutex_unlock(&ttmath_mutex ); 00207 } 00208 }; 00209 00210 #endif // #ifdef TTMATH_POSIX_THREADS 00211 00212 00213 00214 00215 #if !defined(TTMATH_POSIX_THREADS) && !defined(TTMATH_WIN32_THREADS) 00216 00217 /*! 00218 we don't use win32 and pthreads 00219 */ 00220 00221 /*! 00222 */ 00223 #define TTMATH_MULTITHREADS_HELPER 00224 00225 00226 /*! 00227 objects of this class are used to synchronize 00228 actually we don't synchronize, the method Lock() returns always 'false' 00229 */ 00230 class ThreadLock 00231 { 00232 public: 00233 00234 bool Lock() 00235 { 00236 return false; 00237 } 00238 }; 00239 00240 00241 #endif // #if !defined(TTMATH_POSIX_THREADS) && !defined(TTMATH_WIN32_THREADS) 00242 00243 00244 00245 00246 00247 } // namespace 00248 00249 #endif 00250 00251
Generated on Tue Jul 12 2022 14:03:18 by
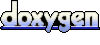