
sensor de color prueba 1
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "scolor_TCS3200.h" 00003 00004 /* ***************************************************************************** 00005 Programa que solicita telemetria al sistema embebido, por medio del comando 0xFe 00006 para preguntar por el color que detecta el sensor TCS3200 00007 00008 el sistema embebido recibe el inicio de trama FE y el número de comado: 01 00009 00010 | INITELE | CMD | 00011 | 0xfe | 0x01 | 00012 00013 00014 para enviar los comandos usar el programa Coolterm http://freeware.the-meiers.org/ 00015 00016 00017 @fabeltranm 2019 00018 fbeltranm@ecci.edu.co 00019 00020 ******************************************************************************** 00021 datasheet https://www.mouser.com/catalog/specsheets/TCS3200-E11.pdf 00022 00023 00024 S0 Frequency scaling 00025 S1 Frequency scaling 00026 S2 Photo diode selection 00027 S3 Photo diode selection 00028 OutFreq Frequency 00029 00030 ----------------------------------- 00031 | ____________ ____________ | 00032 ----> | | | | | | ___ ___ 00033 Light | | Photodiode | | Current |--|---OUTPUT_FREQ | |___| |___ 00034 ----> | | Array |---| to | | 00035 | | | | Frequency | | 00036 | |____________| |____________| | 00037 | ^ ^ ^ ^ | 00038 -------|--|-------------|--|------- 00039 | | | | 00040 S2 S3 S0 S1 00041 00042 SO | S1 | OUTPUT FREQUENCY SCALING | | S2 | S3 | PHOTODIODE TYPE | 00043 0 | 0 | power down | | 0 | 0 | Red | 00044 0 | 1 | 2% | | 0 | 1 | Blue | 00045 1 | 0 | 20% | | 1 | 0 | Clear (no filter) | 00046 1 | 1 | 100% | | 1 | 1 | Green | 00047 00048 ******************************************************************************/ 00049 #define INITCMD 0xFF // comando de sonido ff 01 3 00050 #define INITELE 0xFE // comando de sensor de colores fe 01 00051 #define CMD 0x01 // 00052 00053 #define DO 104 00054 #define RE 153 00055 #define MI 166 00056 #define FA 184 00057 #define SO 205 00058 00059 00060 00061 Serial command(USBTX, USBRX); 00062 // S0, S1, S2, S3, OUT 00063 scolor_TCS3200 scolor(PA_8, PB_10, PB_4, PB_5, PB_3); 00064 PwmOut mybuzzer(PB_9); 00065 00066 // definición de las funciones 00067 00068 void setup_uart(); // funcion conexion pc 00069 void leer_datos(); // funcion de lectura de comandos HEX 00070 void leer_color();// recepcion de la lectura de frecuencia del sensor 00071 00072 void buzzer_on(uint8_t tono, uint8_t tiempo); 00073 00074 uint8_t cmd; 00075 uint8_t tono; // variable almacena la frecuencia del buzzer 00076 uint8_t tiempo; // varable almacena los tiempos del buzzer leer_datos() 00077 00078 int main() { 00079 00080 setup_uart(); 00081 while(1){ 00082 leer_datos(); 00083 if (cmd==CMD){} 00084 leer_color(); 00085 buzzer_on(tono, tiempo); 00086 } 00087 } 00088 00089 00090 00091 void setup_uart(){ 00092 00093 command.baud(115200); 00094 printf ("hola mundo sonido vicion"); 00095 00096 00097 } 00098 00099 00100 void leer_datos(){ 00101 00102 while(command.getc()!= INITELE); 00103 cmd=command.getc(); 00104 00105 while(command.getc()!= INITCMD); 00106 tono=command.getc(); 00107 tiempo=command.getc(); 00108 00109 00110 } 00111 00112 00113 void leer_color(){ 00114 00115 00116 00117 long red = scolor.ReadRed(); 00118 long green = scolor.ReadGreen(); 00119 long blue = scolor.ReadBlue(); 00120 long clear = scolor.ReadClear(); 00121 00122 //printf("RED: %5d GREEN: %5d BLUE: %5d CLEAR: %5d \n ", red, green, blue, clear); 00123 00124 if (red < blue && green > blue && red < 35) 00125 { 00126 printf (" Rojo"); 00127 } 00128 else if (blue < red && blue < green && green < red) 00129 { 00130 printf (" Azul"); 00131 } 00132 else if (red > green && blue > green ) 00133 { 00134 printf(" Verde"); 00135 } 00136 else{ 00137 printf(" "); 00138 } 00139 } 00140 00141 00142 void buzzer_on(uint8_t tono, uint8_t tiempo){ 00143 00144 /* complementar el código necesario 00145 */ 00146 00147 mybuzzer.write(0); 00148 switch(tono){ 00149 case 1: mybuzzer.period_us(DO);break; 00150 case 2: mybuzzer.period_us(RE);break; 00151 case 3: mybuzzer.period_us(MI);break; 00152 case 4: mybuzzer.period_us(FA);break; 00153 default:mybuzzer.period_us(SO); break; 00154 } 00155 mybuzzer.write(0.5); 00156 00157 wait(tiempo); 00158 mybuzzer.write(0); 00159 00160 00161 } 00162 00163 00164
Generated on Fri Jul 15 2022 04:24:59 by
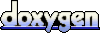