
Basic RX/TX example, this is the firmware that LoDev S76S comes with initially.
Dependencies: mbed SX1276RonothLib
main.cpp
00001 #include "mbed.h" 00002 #include "config.h" 00003 #include "sx1276-mbed-hal.h" 00004 #include "main.h" 00005 00006 // Override stdout to use our serial port 00007 FileHandle* mbed::mbed_override_console(int fd) { 00008 static UARTSerial pc(PA_9, PA_10, 115200); 00009 return &pc; 00010 } 00011 00012 00013 static RadioEvents_t RadioEvents; 00014 00015 SX1276Generic *Radio; 00016 DigitalOut blueLed = DigitalOut(LED); 00017 00018 // NOTE: By default the sample application is in recieve mode. 00019 // Connect PIN PC_2 to 3.3v to transmit 00020 DigitalIn txModeIndicator = DigitalIn(TX_IND, PullDown); 00021 00022 int timeoutCount, errCount, recvCount = 0; 00023 00024 int main() 00025 { 00026 00027 blueLed = 1; 00028 00029 Radio = new SX1276Generic(NULL, RFM95_SX1276, 00030 LORA_SPI_MOSI, LORA_SPI_MISO, LORA_SPI_SCLK, LORA_CS, LORA_RESET, 00031 LORA_DIO0, LORA_DIO1, LORA_DIO2, LORA_DIO3, LORA_DIO4, LORA_DIO5); 00032 00033 printf("LoDev RX/TX Demo Application\n" ); 00034 printf("Freqency: %.1f\n", (double)RF_FREQUENCY/1000000.0); 00035 printf("TXPower: %d dBm\n", TX_OUTPUT_POWER); 00036 printf("Bandwidth: %d Hz\n", LORA_BANDWIDTH); 00037 printf("Spreading factor: SF%d\n", LORA_SPREADING_FACTOR); 00038 00039 // Initialize Radio driver 00040 RadioEvents.TxDone = OnTxDone; 00041 RadioEvents.RxDone = OnRxDone; 00042 RadioEvents.RxError = OnRxError; 00043 RadioEvents.TxTimeout = OnTxTimeout; 00044 RadioEvents.RxTimeout = OnRxTimeout; 00045 if (Radio->Init( &RadioEvents ) == false) { 00046 while(1) { 00047 printf("Radio could not be detected!\n"); 00048 wait( 1 ); 00049 } 00050 } 00051 00052 Radio->DetectBoardType(); 00053 Radio->SetChannel(RF_FREQUENCY ); 00054 00055 Radio->SetTxConfig( MODEM_LORA, TX_OUTPUT_POWER, 0, LORA_BANDWIDTH, 00056 LORA_SPREADING_FACTOR, LORA_CODINGRATE, 00057 LORA_PREAMBLE_LENGTH, LORA_FIX_LENGTH_PAYLOAD_ON, 00058 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00059 LORA_IQ_INVERSION_ON, 2000 ); 00060 00061 Radio->SetRxConfig( MODEM_LORA, LORA_BANDWIDTH, LORA_SPREADING_FACTOR, 00062 LORA_CODINGRATE, 0, LORA_PREAMBLE_LENGTH, 00063 LORA_SYMBOL_TIMEOUT, LORA_FIX_LENGTH_PAYLOAD_ON, 0, 00064 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00065 LORA_IQ_INVERSION_ON, true ); 00066 00067 printf("Starting loop\n"); 00068 00069 uint16_t frameCount = 0; 00070 char buffer[18]; 00071 00072 bool txMode = false; 00073 if(txModeIndicator == 1) { 00074 // Transmit 00075 txMode = true; 00076 } else { 00077 Radio->Rx(0); 00078 } 00079 00080 while( 1 ) 00081 { 00082 blueLed = 1; 00083 if(txMode) { 00084 wait_ms(5000); 00085 sprintf(buffer, "%u Hello World", frameCount); 00086 printf("Sending packet: %s\n", buffer); 00087 Radio->Send( buffer, strlen(buffer) ); 00088 frameCount++; 00089 } else { 00090 wait_ms(100); 00091 } 00092 00093 } 00094 } 00095 00096 void OnTxDone(void *radio, void *userThisPtr, void *userData) 00097 { 00098 Radio->Sleep( ); 00099 blueLed = 0; 00100 printf("> OnTxDone\n"); 00101 } 00102 00103 void OnRxDone(void *radio, void *userThisPtr, void *userData, uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr) 00104 { 00105 Radio->Sleep( ); 00106 blueLed = 0; 00107 recvCount++; 00108 printf("Status: Recv=%d, Error=%d, Timeout=%d\n", recvCount, errCount, timeoutCount); 00109 printf("> OnRxDone: RssiValue=%d dBm, SnrValue=%d\n", rssi, snr); 00110 printf("Data[%d]: %.*s\n", size, size, payload); 00111 Radio->Rx(0); 00112 } 00113 00114 void OnTxTimeout(void *radio, void *userThisPtr, void *userData) 00115 { 00116 Radio->Sleep( ); 00117 blueLed = 0; 00118 printf("> OnTxTimeout\n"); 00119 } 00120 00121 void OnRxTimeout(void *radio, void *userThisPtr, void *userData) 00122 { 00123 Radio->Sleep( ); 00124 blueLed = 0; 00125 printf("> OnRxTimeout\n"); 00126 timeoutCount++; 00127 blueLed = 0; 00128 Radio->Rx(0); 00129 } 00130 00131 void OnRxError(void *radio, void *userThisPtr, void *userData) 00132 { 00133 Radio->Sleep( ); 00134 printf("> OnRxError\n"); 00135 errCount++; 00136 blueLed = 0; 00137 Radio->Rx(0); 00138 }
Generated on Sat Jul 16 2022 09:01:07 by
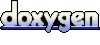