USB Composite support
Dependents: mbed_cdc_hid_composite
Fork of USBDevice by
USBDevice.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBDEVICE_H 00020 #define USBDEVICE_H 00021 00022 #include "mbed.h" 00023 #include "USBDevice_Types.h" 00024 #include "USBHAL.h" 00025 #include "USBInterface.h" 00026 00027 00028 class USBDevice: public USBHAL 00029 { 00030 public: 00031 USBDevice(uint16_t vendor_id = 0x1f00, uint16_t product_id = 0x2012, uint16_t product_release = 0x0001); 00032 00033 /* 00034 * Check if the device is configured 00035 * 00036 * @returns true if configured, false otherwise 00037 */ 00038 bool configured(void); 00039 00040 /* 00041 * Connect a device 00042 * 00043 * @param blocking: block if not configured 00044 */ 00045 void connect(bool blocking = true); 00046 00047 /* 00048 * Disconnect a device 00049 */ 00050 void disconnect(void); 00051 00052 /* 00053 * Add an endpoint 00054 * 00055 * @param endpoint endpoint which will be added 00056 * @param maxPacket Maximum size of a packet which can be sent for this endpoint 00057 * @returns true if successful, false otherwise 00058 */ 00059 bool addEndpoint(uint8_t endpoint, uint32_t maxPacket); 00060 00061 /* 00062 * Start a reading on a certain endpoint. 00063 * You can access the result of the reading by USBDevice_read 00064 * 00065 * @param endpoint endpoint which will be read 00066 * @param maxSize the maximum length that can be read 00067 * @return true if successful 00068 */ 00069 bool readStart(uint8_t endpoint, uint32_t maxSize); 00070 00071 /* 00072 * Read a certain endpoint. Before calling this function, USBUSBDevice_readStart 00073 * must be called. 00074 * 00075 * Warning: blocking 00076 * 00077 * @param endpoint endpoint which will be read 00078 * @param buffer buffer will be filled with the data received 00079 * @param size the number of bytes read will be stored in *size 00080 * @param maxSize the maximum length that can be read 00081 * @returns true if successful 00082 */ 00083 bool readEP(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00084 00085 /* 00086 * Read a certain endpoint. 00087 * 00088 * Warning: non blocking 00089 * 00090 * @param endpoint endpoint which will be read 00091 * @param buffer buffer will be filled with the data received (if data are available) 00092 * @param size the number of bytes read will be stored in *size 00093 * @param maxSize the maximum length that can be read 00094 * @returns true if successful 00095 */ 00096 bool readEP_NB(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00097 00098 /* 00099 * Write a certain endpoint. 00100 * 00101 * Warning: blocking 00102 * 00103 * @param endpoint endpoint to write 00104 * @param buffer data contained in buffer will be write 00105 * @param size the number of bytes to write 00106 * @param maxSize the maximum length that can be written on this endpoint 00107 */ 00108 bool write(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00109 00110 00111 /* 00112 * Write a certain endpoint. 00113 * 00114 * Warning: non blocking 00115 * 00116 * @param endpoint endpoint to write 00117 * @param buffer data contained in buffer will be write 00118 * @param size the number of bytes to write 00119 * @param maxSize the maximum length that can be written on this endpoint 00120 */ 00121 bool writeNB(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00122 00123 00124 /* 00125 * Called by USBDevice layer on bus reset. Warning: Called in ISR context 00126 * 00127 * May be used to reset state 00128 */ 00129 virtual void USBCallback_busReset(void) {}; 00130 00131 00132 /* 00133 * Called by USBDevice on Endpoint0 request. Warning: Called in ISR context 00134 * This is used to handle extensions to standard requests 00135 * and class specific requests 00136 * 00137 * @returns true if class handles this request 00138 */ 00139 virtual bool USBCallback_request() { 00140 if (_intf) 00141 return _intf->USBCallback_request(); 00142 else 00143 return false; 00144 }; 00145 00146 /* 00147 * Called by USBDevice on Endpoint0 request completion 00148 * if the 'notify' flag has been set to true. Warning: Called in ISR context 00149 * 00150 * In this case it is used to indicate that a HID report has 00151 * been received from the host on endpoint 0 00152 * 00153 * @param buf buffer received on endpoint 0 00154 * @param length length of this buffer 00155 */ 00156 virtual void USBCallback_requestCompleted(uint8_t * buf, uint32_t length) { 00157 if (_intf) 00158 _intf->USBCallback_requestCompleted(buf, length); 00159 }; 00160 00161 /* 00162 * Called by USBDevice layer. Set configuration of the device. 00163 * For instance, you can add all endpoints that you need on this function. 00164 * 00165 * @param configuration Number of the configuration 00166 */ 00167 virtual bool USBCallback_setConfiguration(uint8_t configuration) { 00168 if (_intf) 00169 return _intf->USBCallback_setConfiguration(configuration); 00170 return false; 00171 }; 00172 00173 /* 00174 * Called by USBDevice layer. Set interface/alternate of the device. 00175 * 00176 * @param interface Number of the interface to be configured 00177 * @param alternate Number of the alternate to be configured 00178 * @returns true if class handles this request 00179 */ 00180 virtual bool USBCallback_setInterface(uint16_t interface, uint8_t alternate) { return false; }; 00181 00182 /* 00183 * Get device descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00184 * 00185 * @returns pointer to the device descriptor 00186 */ 00187 virtual uint8_t * deviceDesc(); 00188 00189 /* 00190 * Get configuration descriptor 00191 * 00192 * @returns pointer to the configuration descriptor 00193 */ 00194 virtual uint8_t * configurationDesc(){ 00195 if (_intf) 00196 return _intf->configurationDesc(); 00197 return NULL; 00198 }; 00199 00200 /* 00201 * Get string lang id descriptor 00202 * 00203 * @return pointer to the string lang id descriptor 00204 */ 00205 virtual uint8_t * stringLangidDesc(); 00206 00207 /* 00208 * Get string manufacturer descriptor 00209 * 00210 * @returns pointer to the string manufacturer descriptor 00211 */ 00212 virtual uint8_t * stringImanufacturerDesc(); 00213 00214 /* 00215 * Get string product descriptor 00216 * 00217 * @returns pointer to the string product descriptor 00218 */ 00219 virtual uint8_t * stringIproductDesc(); 00220 00221 /* 00222 * Get string serial descriptor 00223 * 00224 * @returns pointer to the string serial descriptor 00225 */ 00226 virtual uint8_t * stringIserialDesc(); 00227 00228 /* 00229 * Get string configuration descriptor 00230 * 00231 * @returns pointer to the string configuration descriptor 00232 */ 00233 virtual uint8_t * stringIConfigurationDesc(); 00234 00235 /* 00236 * Get string interface descriptor 00237 * 00238 * @returns pointer to the string interface descriptor 00239 */ 00240 virtual uint8_t * stringIinterfaceDesc(); 00241 00242 /* 00243 * Get the length of the report descriptor 00244 * 00245 * @returns length of the report descriptor 00246 */ 00247 virtual uint16_t reportDescLength() { return 0; }; 00248 00249 CONTROL_TRANSFER * getTransferPtr(void); 00250 00251 virtual bool bind(USBInterface *intf) { 00252 _intf = intf; 00253 return true; 00254 } 00255 00256 uint16_t VENDOR_ID; 00257 uint16_t PRODUCT_ID; 00258 uint16_t PRODUCT_RELEASE; 00259 00260 protected: 00261 virtual void busReset(void); 00262 virtual void EP0setupCallback(void); 00263 virtual void EP0out(void); 00264 virtual void EP0in(void); 00265 virtual void connectStateChanged(unsigned int connected); 00266 virtual void suspendStateChanged(unsigned int suspended); 00267 uint8_t * findDescriptor(uint8_t descriptorType); 00268 00269 00270 private: 00271 bool addRateFeedbackEndpoint(uint8_t endpoint, uint32_t maxPacket); 00272 bool requestGetDescriptor(void); 00273 bool controlOut(void); 00274 bool controlIn(void); 00275 bool requestSetAddress(void); 00276 bool requestSetConfiguration(void); 00277 bool requestSetFeature(void); 00278 bool requestClearFeature(void); 00279 bool requestGetStatus(void); 00280 bool requestSetup(void); 00281 bool controlSetup(void); 00282 void decodeSetupPacket(uint8_t *data, SETUP_PACKET *packet); 00283 bool requestGetConfiguration(void); 00284 bool requestGetInterface(void); 00285 bool requestSetInterface(void); 00286 00287 CONTROL_TRANSFER transfer; 00288 USB_DEVICE device; 00289 00290 uint16_t currentInterface; 00291 uint8_t currentAlternate; 00292 00293 USBInterface *_intf; 00294 }; 00295 00296 00297 #endif
Generated on Tue Jul 12 2022 20:44:58 by
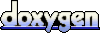