
Basic test program for the SHT2x library
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SHT2x.h" 00003 00004 SHT2x sht21(p9, p10); 00005 00006 DigitalOut myled(LED2); 00007 Serial pc(USBTX, USBRX); 00008 00009 int rh, temp; 00010 int error; 00011 float relHumidity, temperature; 00012 int userRegister; 00013 00014 int main() { 00015 sht21.SHT2x_SoftReset(); 00016 00017 error |= sht21.SHT2x_ReadUserRegister(&userRegister); //get actual user reg 00018 userRegister = (userRegister & ~SHT2x_RES_MASK) | SHT2x_RES_12_14BIT; 00019 error |= sht21.SHT2x_WriteUserRegister(&userRegister); //write changed user reg 00020 00021 while (1) { 00022 myled = 1; 00023 00024 pc.printf("program start\r\n"); 00025 error |= sht21.SHT2x_MeasureHM(HUMIDITY, &rh); 00026 error |= sht21.SHT2x_MeasureHM(TEMP, &temp); 00027 00028 if (error > 0) 00029 pc.printf("error code %d \r\n", error); 00030 00031 relHumidity = sht21.SHT2x_CalcRH(rh); 00032 temperature = sht21.SHT2x_CalcTemperatureC(temp); 00033 00034 pc.printf("RH value -> %f \r\n", relHumidity); 00035 pc.printf("Temp in C -> %f \r\n", temperature); 00036 pc.printf("Dew point %f \r\n", sht21.SHT2x_GetDewpoint(relHumidity, temperature)); 00037 00038 myled = 0; 00039 wait(1); 00040 } 00041 }
Generated on Thu Jul 14 2022 21:39:02 by
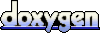