Port of the SHT2x example code from Sensirion
Dependents: sht21_test ENVLogger
SHT2x.h
00001 /* 00002 Permission is hereby granted, free of charge, to any person obtaining a copy 00003 of this software and associated documentation files (the "Software"), to deal 00004 in the Software without restriction, including without limitation the rights 00005 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00006 copies of the Software, and to permit persons to whom the Software is 00007 furnished to do so, subject to the following conditions: 00008 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00013 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00014 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00015 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00016 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00018 THE SOFTWARE. 00019 */ 00020 00021 //============================================================================= 00022 // Port of the SHT21 example code from Sensirion 00023 // port by Serge Sozonoff 00024 //============================================================================= 00025 00026 #include "mbed.h" 00027 #include "SHT21_I2C.h" 00028 00029 #ifndef SHT2x_H 00030 #define SHT2x_H 00031 00032 const int POLYNOMIAL = 0x131; //P(x)=x^8+x^5+x^4+1 = 100110001 00033 const int ACK = 1; 00034 const int NoACK = 0; 00035 00036 // sensor command 00037 enum etSHT2xCommand { 00038 TRIG_T_MEASUREMENT_HM = 0xE3, // command trig. temp meas. hold master 00039 TRIG_RH_MEASUREMENT_HM = 0xE5, // command trig. humidity meas. hold master 00040 TRIG_T_MEASUREMENT_POLL = 0xF3, // command trig. temp meas. no hold master 00041 TRIG_RH_MEASUREMENT_POLL = 0xF5, // command trig. humidity meas. no hold master 00042 USER_REG_W = 0xE6, // command writing user register 00043 USER_REG_R = 0xE7, // command reading user register 00044 SOFT_RESET = 0xFE // command soft reset 00045 }; 00046 00047 enum etSHT2xResolution { 00048 SHT2x_RES_12_14BIT = 0x00, // RH=12bit, T=14bit 00049 SHT2x_RES_8_12BIT = 0x01, // RH= 8bit, T=12bit 00050 SHT2x_RES_10_13BIT = 0x80, // RH=10bit, T=13bit 00051 SHT2x_RES_11_11BIT = 0x81, // RH=11bit, T=11bit 00052 SHT2x_RES_MASK = 0x81 // Mask for res. bits (7,0) in user reg. 00053 }; 00054 00055 enum etSHT2xEob { 00056 SHT2x_EOB_ON = 0x40, // end of battery 00057 SHT2x_EOB_MASK = 0x40, // Mask for EOB bit(6) in user reg. 00058 }; 00059 00060 enum etSHT2xHeater { 00061 SHT2x_HEATER_ON = 0x04, // heater on 00062 SHT2x_HEATER_OFF = 0x00, // heater off 00063 SHT2x_HEATER_MASK = 0x04, // Mask for Heater bit(2) in user reg. 00064 }; 00065 // measurement signal selection 00066 00067 enum etSHT2xMeasureType { 00068 HUMIDITY, 00069 TEMP 00070 }; 00071 00072 enum etI2cHeader { 00073 I2C_ADR_W = 128, // sensor I2C address + write bit 00074 I2C_ADR_R = 129 // sensor I2C address + read bit 00075 }; 00076 00077 // Error codes 00078 enum etError { 00079 ACK_ERROR = 0x01, 00080 TIME_OUT_ERROR = 0x02, 00081 CHECKSUM_ERROR = 0x04, 00082 UNIT_ERROR = 0x08 00083 }; 00084 00085 class SHT2x : public Base { 00086 public: 00087 SHT2x (PinName p_sda, PinName p_scl); 00088 00089 int SHT2x_CheckCrc(int data[], int nbrOfBytes, int checksum); 00090 int SHT2x_ReadUserRegister(int *pRegisterValue); 00091 int SHT2x_WriteUserRegister(int *pRegisterValue); 00092 int SHT2x_MeasurePoll(etSHT2xMeasureType eSHT2xMeasureType, int *pMeasurand); 00093 int SHT2x_MeasureHM(etSHT2xMeasureType eSHT2xMeasureType, int *pMeasurand); 00094 int SHT2x_SoftReset(); 00095 float SHT2x_CalcRH(int u16sRH); 00096 float SHT2x_CalcTemperatureC(int u16sT); 00097 int SHT2x_GetSerialNumber(int u8SerialNumber[]); 00098 float SHT2x_GetDewpoint(float h, float t); 00099 00100 protected: 00101 SHT_I2C i2c; 00102 int i2cWrite(int data); 00103 00104 private: 00105 00106 }; 00107 #endif
Generated on Wed Jul 13 2022 05:08:43 by
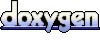