http://uk.farnell.com/batron/bthq21605v-cog-fsre-i2c/lcd-module-alphanumeric-2x16/dp/1220409
Dependents: PCF2119_16X2_LCD_test LPC1768BagSensor
PCF2119_16X2_LCD.cpp
00001 #include "PC2119_16X2_LCD.h" 00002 #include <stdarg.h> 00003 00004 void PC2119_16X2_LCD::Init() { 00005 // reset the LCD using external reset pin 00006 _reset = 1; 00007 wait_us(300); 00008 _reset = 0; 00009 wait_us(12000); 00010 00011 char buf[15]; 00012 buf[0] = INSTRUCTION_CTRL_BYTE; 00013 buf[1] = (FUNCTION_SET | 0x01); // Function set + extended instruction set 00014 buf[2] = CURSOR_DISLPAY_SHIFT; 00015 buf[3] = ICON_CTRL; 00016 buf[4] = VLCD_MULTIPLIER; //set Vlcd multiplier 00017 buf[5] = VLCD_CONTRAST; //set Vlcd Contrast 00018 00019 buf[6] = FUNCTION_SET; // basic instructions 00020 buf[7] = DISPLAY_CTL; 00021 buf[8] = ENTRY_MODE; 00022 buf[9] = CLEAR_DISPLAY; 00023 00024 _i2c.write(LCD_I2C_ADR, buf, 10); 00025 } 00026 00027 00028 PC2119_16X2_LCD::PC2119_16X2_LCD(PinName p_sda, PinName p_scl, PinName p_reset) : _i2c(p_sda, p_scl), _reset(p_reset) { 00029 00030 PC2119_16X2_LCD::Init(); 00031 } 00032 00033 PC2119_16X2_LCD::PC2119_16X2_LCD(I2C& p_i2c, PinName p_reset) : _i2c(p_i2c), _reset(p_reset) { 00034 00035 PC2119_16X2_LCD::Init(); 00036 00037 } 00038 00039 char PC2119_16X2_LCD::ascii_to_lcd(char ch) { 00040 char c = BLANK_CHAR; // default: white space 00041 00042 if ((ch>=' ') & (ch<='?')) 00043 c = ASCII_OFFSET + ch; 00044 if ((ch>='A') & (ch<='Z')) 00045 c = ASCII_OFFSET + ch; 00046 if ((ch>='a') & (ch<='z')) 00047 c = ASCII_OFFSET + ch; 00048 return c; 00049 } 00050 00051 void PC2119_16X2_LCD::write_xy(char c, char row, char column, bool raw) { 00052 char buf[0x10]; 00053 char adr; 00054 00055 if (row == 0) // line offset 00056 adr = column; 00057 else 00058 adr = DATA_CTRL_BYTE + column; 00059 00060 buf[0] = INSTRUCTION_CTRL_BYTE; // Enter function setting 00061 buf[1] = 0x80 + adr; // LCD adr counter set to "adr" 00062 00063 _i2c.write(LCD_I2C_ADR,(char *)buf,2); 00064 00065 buf[0] = DATA_CTRL_BYTE; // write to DDRAM 00066 buf[1] = raw ? c : ascii_to_lcd(c); 00067 00068 _i2c.write(LCD_I2C_ADR,(char *)buf,2); 00069 } 00070 00071 void PC2119_16X2_LCD::clear_line(char row) { 00072 if (row == 0 || row == 1) { 00073 char i=0; 00074 do 00075 write_xy(BLANK_CHAR, row, i); 00076 while ((i++<16)); 00077 } 00078 } 00079 00080 void PC2119_16X2_LCD::clear_display(void) { 00081 clear_line(0); // clear 1st line 00082 clear_line(1); // clear 2nd line 00083 return; 00084 } 00085 00086 void PC2119_16X2_LCD::write(char *b) { 00087 char buf[0x60]; 00088 int a; 00089 00090 buf[0]=INSTRUCTION_CTRL_BYTE; 00091 buf[1]=0x80; 00092 _i2c.write(LCD_I2C_ADR,(char *)buf,2); 00093 00094 a=0; 00095 buf[0]=DATA_CTRL_BYTE; 00096 00097 while (a!=strlen(b)) { 00098 buf[a+1]=ascii_to_lcd(b[a]); 00099 a++; 00100 } 00101 buf[a+1]=INSTRUCTION_CTRL_BYTE; 00102 00103 _i2c.write(LCD_I2C_ADR,buf,strlen(b)+1); 00104 00105 buf[0]=0x80; 00106 buf[1]=0x02; 00107 _i2c.write(LCD_I2C_ADR,(char *)buf,2); 00108 } 00109 00110 void PC2119_16X2_LCD::printf(const char * format, ... ) { 00111 // create and start the va_list 00112 va_list listPointer ; 00113 va_start( listPointer, format ) ; 00114 00115 // vsprintf is an example of 00116 // a function that works with 00117 // an "already started" va_list 00118 static char buf[ 1024 ] ; 00119 vsprintf( buf, format, listPointer ) ; 00120 00121 return write(buf); 00122 } 00123 00124 void PC2119_16X2_LCD::flip_display() { 00125 _i2c.start(); 00126 _i2c.write(LCD_I2C_ADR); 00127 _i2c.write(INSTRUCTION_CTRL_BYTE); // Control byte for Instruction 00128 _i2c.write(FUNCTION_SET | 0x01); // extended instruction mode 00129 _i2c.write(0x07); // P=1; Q=1; 00130 _i2c.write(FUNCTION_SET); 00131 _i2c.stop(); 00132 } 00133 00134 void PC2119_16X2_LCD::unflip_display() { 00135 _i2c.start(); 00136 _i2c.write(LCD_I2C_ADR); 00137 _i2c.write(INSTRUCTION_CTRL_BYTE); // Control byte for Instruction 00138 _i2c.write(FUNCTION_SET | 0x01); 00139 _i2c.write(0x04); // P=0; Q=0; 00140 _i2c.write(FUNCTION_SET); 00141 _i2c.stop(); 00142 }
Generated on Sat Jul 23 2022 00:56:26 by
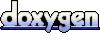