
Complete Code
Dependencies: 4DGL-uLCD-SE mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "uLCD_4DGL.h" 00004 00005 uLCD_4DGL uLCD(p9, p10, p11); // create a global lcd object 00006 //ADXL345 accelerometer(p5, p6, p7, p8);//create a global accelerometer object 00007 Serial pc(USBTX, USBRX);// for debugging 00008 Mutex lcd_mutex;// Create the mutex lock object 00009 //PwmOut speaker(p21); //create a PwmOut object for the speaker 00010 //SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00011 AnalogIn jsx(p19); 00012 //AnalogIn jsy(p20); 00013 00014 int playerx, playery, ob1x, ob1y, ob2x, ob2y, ob3x, ob3y; 00015 int x=0,y=0; 00016 double obwait=0.2; 00017 00018 void thread1(void const *args){ 00019 //generates obstacles 00020 while(true){ 00021 00022 //lcd_mutex.lock();//acquire lock 00023 /*if(ob1y>127 && playerx==26){ 00024 ob1x=26; 00025 //ob1y=-16; 00026 ob1y=0; 00027 } 00028 00029 if(ob2y>127 && playerx==58){ 00030 ob2x=58; 00031 //ob2y=-16; 00032 ob2y=0; 00033 } 00034 00035 if(ob3y>127 && playerx==90){ 00036 ob3x=90; 00037 //ob3y=-16; 00038 ob3y=0; 00039 }*/ 00040 00041 lcd_mutex.lock();//acquire lock 00042 uLCD.filled_rectangle(ob1x,ob1y,ob1x+15,ob1y+15,0x000000);//erase previous position 00043 ob1y=ob1y+16; 00044 00045 uLCD.filled_rectangle(ob2x,ob2y,ob2x+15,ob2y+15,0x000000);//erase previous position 00046 ob2y=ob2y+16; 00047 00048 uLCD.filled_rectangle(ob3x,ob3y,ob3x+15,ob3y+15,0x000000);//erase previous position 00049 ob3y=ob3y+16; 00050 00051 if(ob1y>127 && playerx==26){ 00052 ob1x=26; 00053 //ob1y=-16; 00054 ob1y=16; 00055 } 00056 00057 if(ob2y>127 && playerx==58){ 00058 ob2x=58; 00059 //ob2y=-16; 00060 ob2y=16; 00061 } 00062 00063 if(ob3y>127 && playerx==90){ 00064 ob3x=90; 00065 //ob3y=-16; 00066 ob3y=16; 00067 } 00068 00069 00070 //for(x=0;x<10;x++){ 00071 uLCD.filled_rectangle(ob1x,ob1y,ob1x+15,ob1y+15,0xFF3300);//change position 00072 uLCD.filled_rectangle(ob2x,ob2y,ob2x+15,ob2y+15,0xFF3300);//change position 00073 uLCD.filled_rectangle(ob3x,ob3y,ob3x+15,ob3y+15,0xFF3300);//change position 00074 //} 00075 00076 lcd_mutex.unlock();//release lock 00077 00078 y++; 00079 if(y%50==0) 00080 obwait=(obwait/2); 00081 if(obwait<0.0005) 00082 obwait=0.0005; 00083 pc.printf("y=%d\nobwait=%f\n", y, obwait); 00084 00085 lcd_mutex.lock(); 00086 00087 uLCD.text_width(1); //4X size text 00088 uLCD.text_height(1); 00089 uLCD.locate(0,0); 00090 uLCD.set_font(FONT_7X8); 00091 uLCD.text_mode(OPAQUE); 00092 uLCD.printf("Sc=%d",y); 00093 uLCD.locate(10,0); 00094 uLCD.printf("Sp=%d",((y/50)+1)); 00095 00096 lcd_mutex.unlock(); 00097 00098 wait(obwait); 00099 } 00100 } 00101 00102 00103 00104 00105 int main(){ 00106 float jx; 00107 uLCD.cls();// clear the LCD 00108 00109 uLCD.text_width(2); //2X size text 00110 uLCD.text_height(2); 00111 00112 00113 uLCD.locate(0,2); 00114 00115 uLCD.printf("\nAuto Race\n");//display the Title 00116 wait(5); 00117 00118 uLCD.cls(); 00119 00120 ob1x=26; 00121 ob1y=140; 00122 00123 ob2x=58; 00124 ob2y=140; 00125 00126 ob3x=90; 00127 ob3y=140; 00128 00129 playerx=58; 00130 playery=112; 00131 00132 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x0000FF); 00133 00134 uLCD.filled_rectangle(110,16,116,127,0xFFFF00); 00135 uLCD.filled_rectangle(15,16,21,127,0xFFFF00); 00136 00137 uLCD.filled_rectangle(116,16,127,127,0x009900); 00138 uLCD.filled_rectangle(0,16,15,127,0x009900); 00139 00140 uLCD.line(0,15,127,15,0xFFFF00); 00141 00142 //uLCD.line(81.5,0,81.5,12,WHITE); 00143 //uLCD.line(48.5,0,48.5,12,WHITE); 00144 00145 uLCD.line(81.5,16,81.5,28,WHITE); 00146 uLCD.line(48.5,16,48.5,28,WHITE); 00147 00148 uLCD.line(81.5,32,81.5,44,WHITE); 00149 uLCD.line(48.5,32,48.5,44,WHITE); 00150 00151 uLCD.line(81.5,48,81.5,60,WHITE); 00152 uLCD.line(48.5,48,48.5,60,WHITE); 00153 00154 uLCD.line(81.5,64,81.5,76,WHITE); 00155 uLCD.line(48.5,64,48.5,76,WHITE); 00156 00157 uLCD.line(81.5,80,81.5,92,WHITE); 00158 uLCD.line(48.5,80,48.5,92,WHITE); 00159 00160 uLCD.line(81.5,96,81.5,108,WHITE); 00161 uLCD.line(48.5,96,48.5,108,WHITE); 00162 00163 uLCD.line(81.5,112,81.5,124,WHITE); 00164 uLCD.line(48.5,112,48.5,124,WHITE); 00165 00166 Thread t1(thread1); 00167 00168 while(true){ 00169 jx=jsx; 00170 pc.printf("%f\n",jx); 00171 if(playerx == 58){ 00172 lcd_mutex.lock();//acquire lock 00173 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x000000); 00174 lcd_mutex.unlock();//release lock 00175 if(jsx>.9){ 00176 playerx+=32; 00177 } 00178 else if(jsx<.1){ 00179 playerx-=32; 00180 } 00181 lcd_mutex.lock();//acquire lock 00182 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x0000FF); 00183 lcd_mutex.unlock();//release lock 00184 //wait(0.5); 00185 } 00186 00187 /* if((playerx==ob1x && playery==ob1y) || (playerx==ob2x && playery==ob2y) || (playerx==ob3x && playery==ob3y)){ 00188 //if((playerx==ob3x && playery==ob3y)){ 00189 00190 //if((playerx==ob1x) || (playerx==ob2x) || (playerx==ob3x)){ 00191 thread2.signal_set(0x1); 00192 lcd_mutex.lock(); 00193 uLCD.cls(); 00194 00195 uLCD.text_width(2); //2X size text 00196 uLCD.text_height(2); 00197 uLCD.color(RED); 00198 uLCD.locate(2,2); 00199 uLCD.printf("\n GAME \n OVER!\n"); 00200 wait(2); 00201 break; 00202 00203 uLCD.cls(); 00204 wait(2); 00205 lcd_mutex.unlock(); 00206 00207 } 00208 */ 00209 else if(playerx == 26){ 00210 lcd_mutex.lock();//acquire lock 00211 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x000000); 00212 lcd_mutex.unlock();//release lock 00213 if(jsx>.9){ 00214 playerx+=32; 00215 } 00216 lcd_mutex.lock();//acquire lock 00217 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x0000FF); 00218 lcd_mutex.unlock();//release lock 00219 //wait(0.5); 00220 } 00221 00222 00223 00224 00225 /* if((playerx==ob1x && playery==ob1y) || (playerx==ob2x && playery==ob2y) || (playerx==ob3x && playery==ob3y)){ 00226 //if((playerx==ob3x && playery==ob3y)){ 00227 00228 //if((playerx==ob1x) || (playerx==ob2x) || (playerx==ob3x)){ 00229 lcd_mutex.lock(); 00230 uLCD.cls(); 00231 00232 uLCD.text_width(2); //2X size text 00233 uLCD.text_height(2); 00234 uLCD.color(RED); 00235 uLCD.locate(2,2); 00236 uLCD.printf("\n GAME \n OVER!\n"); 00237 wait(2); 00238 break; 00239 00240 uLCD.cls(); 00241 wait(2); 00242 lcd_mutex.unlock(); 00243 00244 } 00245 */ 00246 else if(playerx==90) { 00247 lcd_mutex.lock();//acquire lock 00248 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x000000); 00249 lcd_mutex.unlock();//release lock 00250 if(jsx<.1){ 00251 playerx-=32; 00252 } 00253 lcd_mutex.lock();//acquire lock 00254 uLCD.filled_rectangle(playerx,playery,playerx+15,playery+15,0x0000FF); 00255 lcd_mutex.unlock();//release lock 00256 //wait(0.5); 00257 } 00258 //lcd_mutex.lock(); 00259 if((playerx==ob1x && playery==ob1y) || (playerx==ob2x && playery==ob2y) || (playerx==ob3x && playery==ob3y)){ 00260 lcd_mutex.lock(); 00261 uLCD.cls(); 00262 00263 uLCD.text_width(2); //2X size text 00264 uLCD.text_height(2); 00265 uLCD.color(RED); 00266 uLCD.locate(2,2); 00267 uLCD.printf("\n GAME \n OVER!\n"); 00268 wait(2); 00269 uLCD.cls(); 00270 uLCD.text_width(2); //2X size text 00271 uLCD.text_height(2); 00272 uLCD.locate(2,2); 00273 uLCD.printf("\nScore=%d\n",y); 00274 00275 break; 00276 00277 uLCD.cls(); 00278 wait(2); 00279 lcd_mutex.unlock(); 00280 00281 } 00282 /*if((playerx==ob2x && playery==ob2y)){ 00283 //if((playerx==ob3x && playery==ob3y)){ 00284 00285 //if((playerx==ob1x) || (playerx==ob2x) || (playerx==ob3x)){ 00286 lcd_mutex.lock(); 00287 uLCD.cls(); 00288 00289 uLCD.text_width(2); //2X size text 00290 uLCD.text_height(2); 00291 uLCD.color(RED); 00292 uLCD.locate(2,2); 00293 uLCD.printf("\n GAME \n OVER!\n"); 00294 wait(2); 00295 break; 00296 00297 uLCD.cls(); 00298 wait(2); 00299 lcd_mutex.unlock(); 00300 00301 } 00302 if((playerx==ob3x && playery==ob3y)){ 00303 //if((playerx==ob3x && playery==ob3y)){ 00304 00305 //if((playerx==ob1x) || (playerx==ob2x) || (playerx==ob3x)){ 00306 lcd_mutex.lock(); 00307 uLCD.cls(); 00308 00309 uLCD.text_width(2); //2X size text 00310 uLCD.text_height(2); 00311 uLCD.color(RED); 00312 uLCD.locate(2,2); 00313 uLCD.printf("\n GAME \n OVER!\n"); 00314 wait(2); 00315 break; 00316 00317 uLCD.cls(); 00318 wait(2); 00319 lcd_mutex.unlock(); 00320 00321 }*/ 00322 } 00323 }
Generated on Fri Jul 22 2022 07:38:41 by
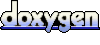