PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Embed:
(wiki syntax)
Show/hide line numbers
timer_11u6x.h
00001 /* 00002 * @brief LPC11u6x 16/32-bit Timer/PWM control functions 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2013 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __TIMER_11U6X_H_ 00033 #define __TIMER_11U6X_H_ 00034 00035 #include "lpc_defs.h" 00036 #include <stdint.h> 00037 00038 /* Static data/function define */ 00039 #define STATIC static 00040 /* External data/function define */ 00041 #define EXTERN extern 00042 #define INLINE inline 00043 #define bool uint8_t 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** @defgroup TIMER_11U6X CHIP: LPC11u6x 16/32-bit Timer driver 00050 * @ingroup CHIP_11U6X_Drivers 00051 * <b>IMPORTANT NOTE ABOUT lpc11u6x TIMERS</b><br> 00052 * For timer 0 on both the 16-bit and 32-bit timers, the capture functions 00053 * use index 0 for capture 0 functions and index 2 for capture 1 functions, 00054 * while timer 1 for both the 16-bit and 32-bit timers uses index 0 and 00055 * index 1. Use care when selecting 00056 * The LPC11U6X User manual is inconsistent in it's designation of capture 00057 * channels for each timer. See the comments for each function for special 00058 * handling per timer when dealing with capture channels. 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief 32-bit Standard timer register block structure 00064 */ 00065 typedef struct { /*!< TIMERn Structure */ 00066 __IO uint32_t IR ; /*!< Interrupt Register. The IR can be written to clear interrupts. The IR can be read to identify which of eight possible interrupt sources are pending. */ 00067 __IO uint32_t TCR ; /*!< Timer Control Register. The TCR is used to control the Timer Counter functions. The Timer Counter can be disabled or reset through the TCR. */ 00068 __IO uint32_t TC ; /*!< Timer Counter. The 32 bit TC is incremented every PR+1 cycles of PCLK. The TC is controlled through the TCR. */ 00069 __IO uint32_t PR ; /*!< Prescale Register. The Prescale Counter (below) is equal to this value, the next clock increments the TC and clears the PC. */ 00070 __IO uint32_t PC ; /*!< Prescale Counter. The 32 bit PC is a counter which is incremented to the value stored in PR. When the value in PR is reached, the TC is incremented and the PC is cleared. The PC is observable and controllable through the bus interface. */ 00071 __IO uint32_t MCR ; /*!< Match Control Register. The MCR is used to control if an interrupt is generated and if the TC is reset when a Match occurs. */ 00072 __IO uint32_t MR[4]; /*!< Match Register. MR can be enabled through the MCR to reset the TC, stop both the TC and PC, and/or generate an interrupt every time MR matches the TC. */ 00073 __IO uint32_t CCR ; /*!< Capture Control Register. The CCR controls which edges of the capture inputs are used to load the Capture Registers and whether or not an interrupt is generated when a capture takes place. */ 00074 __IO uint32_t CR[4]; /*!< Capture Register. CR is loaded with the value of TC when there is an event on the CAPn.0 input. */ 00075 __IO uint32_t EMR ; /*!< External Match Register. The EMR controls the external match pins MATn.0-3 (MAT0.0-3 and MAT1.0-3 respectively). */ 00076 __I uint32_t RESERVED0[12]; 00077 __IO uint32_t CTCR ; /*!< Count Control Register. The CTCR selects between Timer and Counter mode, and in Counter mode selects the signal and edge(s) for counting. */ 00078 __IO uint32_t PWMC; 00079 } LPC_TIMER_T; 00080 00081 /** Macro to clear interrupt pending */ 00082 #define TIMER_IR_CLR(n) _BIT(n) 00083 00084 /** Macro for getting a timer match interrupt bit */ 00085 #define TIMER_MATCH_INT(n) (_BIT((n) & 0x0F)) 00086 /** Macro for getting a capture event interrupt bit */ 00087 #define TIMER_CAP_INT(n) (_BIT((((n) & 0x0F) + 4))) 00088 00089 /** Timer/counter enable bit */ 00090 #define TIMER_ENABLE ((uint32_t) (1 << 0)) 00091 /** Timer/counter reset bit */ 00092 #define TIMER_RESET ((uint32_t) (1 << 1)) 00093 00094 /** Bit location for interrupt on MRx match, n = 0 to 3 */ 00095 #define TIMER_INT_ON_MATCH(n) (_BIT(((n) * 3))) 00096 /** Bit location for reset on MRx match, n = 0 to 3 */ 00097 #define TIMER_RESET_ON_MATCH(n) (_BIT((((n) * 3) + 1))) 00098 /** Bit location for stop on MRx match, n = 0 to 3 */ 00099 #define TIMER_STOP_ON_MATCH(n) (_BIT((((n) * 3) + 2))) 00100 00101 /** Bit location for CAP.n on CRx rising edge, n = 0 to 3 */ 00102 #define TIMER_CAP_RISING(n) (_BIT(((n) * 3))) 00103 /** Bit location for CAP.n on CRx falling edge, n = 0 to 3 */ 00104 #define TIMER_CAP_FALLING(n) (_BIT((((n) * 3) + 1))) 00105 /** Bit location for CAP.n on CRx interrupt enable, n = 0 to 3 */ 00106 #define TIMER_INT_ON_CAP(n) (_BIT((((n) * 3) + 2))) 00107 00108 /** 00109 * @brief Initialize a timer 00110 * @param pTMR : Pointer to timer IP register address 00111 * @return Nothing 00112 */ 00113 void Chip_TIMER_Init(LPC_TIMER_T *pTMR); 00114 00115 /** 00116 * @brief Shutdown a timer 00117 * @param pTMR : Pointer to timer IP register address 00118 * @return Nothing 00119 */ 00120 void Chip_TIMER_DeInit(LPC_TIMER_T *pTMR); 00121 00122 /** 00123 * @brief Determine if a match interrupt is pending 00124 * @param pTMR : Pointer to timer IP register address 00125 * @param matchnum : Match interrupt number to check 00126 * @return false if the interrupt is not pending, otherwise true 00127 * @note Determine if the match interrupt for the passed timer and match 00128 * counter is pending.<br> 00129 */ 00130 STATIC INLINE bool Chip_TIMER_MatchPending(LPC_TIMER_T *pTMR, int8_t matchnum) 00131 { 00132 return (bool) ((pTMR->IR & TIMER_MATCH_INT(matchnum)) != 0); 00133 } 00134 00135 /** 00136 * @brief Determine if a capture interrupt is pending 00137 * @param pTMR : Pointer to timer IP register address 00138 * @param capnum : Capture interrupt number to check 00139 * @return false if the interrupt is not pending, otherwise true 00140 * @note Determine if the capture interrupt for the passed capture pin is 00141 * pending.<br> 00142 * <b>Special handling for timer 0</b><br> 00143 * For 16-bit and 32-bit timers 0, select channel 2 to check the capture 00144 * interrupt status for channel 1. For 16-bit and 32-bit timers 1, select 00145 * channel 1. (User manual designation of channel 1 is capture slot 2 00146 * for timer 0). 00147 */ 00148 STATIC INLINE bool Chip_TIMER_CapturePending(LPC_TIMER_T *pTMR, int8_t capnum) 00149 { 00150 return (bool) ((pTMR->IR & TIMER_CAP_INT(capnum)) != 0); 00151 } 00152 00153 /** 00154 * @brief Clears a (pending) match interrupt 00155 * @param pTMR : Pointer to timer IP register address 00156 * @param matchnum : Match interrupt number to clear 00157 * @return Nothing 00158 * @note Clears a pending timer match interrupt.<br> 00159 */ 00160 STATIC INLINE void Chip_TIMER_ClearMatch(LPC_TIMER_T *pTMR, int8_t matchnum) 00161 { 00162 pTMR->IR = TIMER_IR_CLR(matchnum); 00163 } 00164 00165 /** 00166 * @brief Clears a (pending) capture interrupt 00167 * @param pTMR : Pointer to timer IP register address 00168 * @param capnum : Capture interrupt number to clear 00169 * @return Nothing 00170 * @note Clears a pending timer capture interrupt.<br> 00171 * <b>Special handling for timer 0</b><br> 00172 * For 16-bit and 32-bit timers 0, select channel 2 to check the capture 00173 * interrupt status for channel 1. For 16-bit and 32-bit timers 1, select 00174 * channel 1. (User manual designation of channel 1 is capture slot 2 00175 * for timer 0). 00176 */ 00177 STATIC INLINE void Chip_TIMER_ClearCapture(LPC_TIMER_T *pTMR, int8_t capnum) 00178 { 00179 pTMR->IR = (0x10 << capnum); 00180 } 00181 00182 /** 00183 * @brief Enables the timer (starts count) 00184 * @param pTMR : Pointer to timer IP register address 00185 * @return Nothing 00186 * @note Enables the timer to start counting. 00187 */ 00188 STATIC INLINE void Chip_TIMER_Enable(LPC_TIMER_T *pTMR) 00189 { 00190 pTMR->TCR |= TIMER_ENABLE; 00191 } 00192 00193 /** 00194 * @brief Disables the timer (stops count) 00195 * @param pTMR : Pointer to timer IP register address 00196 * @return Nothing 00197 * @note Disables the timer to stop counting. 00198 */ 00199 STATIC INLINE void Chip_TIMER_Disable(LPC_TIMER_T *pTMR) 00200 { 00201 pTMR->TCR &= ~TIMER_ENABLE; 00202 } 00203 00204 /** 00205 * @brief Returns the current timer count 00206 * @param pTMR : Pointer to timer IP register address 00207 * @return Current timer terminal count value 00208 * @note Returns the current timer terminal count. 00209 */ 00210 STATIC INLINE uint32_t Chip_TIMER_ReadCount(LPC_TIMER_T *pTMR) 00211 { 00212 return pTMR->TC ; 00213 } 00214 00215 /** 00216 * @brief Returns the current prescale count 00217 * @param pTMR : Pointer to timer IP register address 00218 * @return Current timer prescale count value 00219 * @note Returns the current prescale count. 00220 */ 00221 STATIC INLINE uint32_t Chip_TIMER_ReadPrescale(LPC_TIMER_T *pTMR) 00222 { 00223 return pTMR->PC ; 00224 } 00225 00226 /** 00227 * @brief Sets the prescaler value 00228 * @param pTMR : Pointer to timer IP register address 00229 * @param prescale : Prescale value to set the prescale register to 00230 * @return Nothing 00231 * @note Sets the prescale count value. 00232 */ 00233 STATIC INLINE void Chip_TIMER_PrescaleSet(LPC_TIMER_T *pTMR, uint32_t prescale) 00234 { 00235 pTMR->PR = prescale; 00236 } 00237 00238 /** 00239 * @brief Sets a timer match value 00240 * @param pTMR : Pointer to timer IP register address 00241 * @param matchnum : Match timer to set match count for 00242 * @param matchval : Match value for the selected match count 00243 * @return Nothing 00244 * @note Sets one of the timer match values. 00245 */ 00246 STATIC INLINE void Chip_TIMER_SetMatch(LPC_TIMER_T *pTMR, int8_t matchnum, uint32_t matchval) 00247 { 00248 pTMR->MR [matchnum] = matchval; 00249 } 00250 00251 /** 00252 * @brief Reads a capture register 00253 * @param pTMR : Pointer to timer IP register address 00254 * @param capnum : Capture register to read 00255 * @return The selected capture register value 00256 * @note Returns the selected capture register value.<br> 00257 * <b>Special handling for timer 0</b><br> 00258 * For 16-bit and 32-bit timers 0, select channel 2 to get the capture 00259 * count for channel 1. For 16-bit and 32-bit timers 1, select 00260 * channel 1. (User manual designation of channel 1 is capture slot 2 00261 * for timer 0). 00262 */ 00263 STATIC INLINE uint32_t Chip_TIMER_ReadCapture(LPC_TIMER_T *pTMR, int8_t capnum) 00264 { 00265 return pTMR->CR [capnum]; 00266 } 00267 00268 /** 00269 * @brief Resets the timer terminal and prescale counts to 0 00270 * @param pTMR : Pointer to timer IP register address 00271 * @return Nothing 00272 */ 00273 void Chip_TIMER_Reset(LPC_TIMER_T *pTMR); 00274 00275 /** 00276 * @brief Enables a match interrupt that fires when the terminal count 00277 * matches the match counter value. 00278 * @param pTMR : Pointer to timer IP register address 00279 * @param matchnum : Match timer, 0 to 3 00280 * @return Nothing 00281 */ 00282 STATIC INLINE void Chip_TIMER_MatchEnableInt(LPC_TIMER_T *pTMR, int8_t matchnum) 00283 { 00284 pTMR->MCR |= TIMER_INT_ON_MATCH(matchnum); 00285 } 00286 00287 /** 00288 * @brief Disables a match interrupt for a match counter. 00289 * @param pTMR : Pointer to timer IP register address 00290 * @param matchnum : Match timer, 0 to 3 00291 * @return Nothing 00292 */ 00293 STATIC INLINE void Chip_TIMER_MatchDisableInt(LPC_TIMER_T *pTMR, int8_t matchnum) 00294 { 00295 pTMR->MCR &= ~TIMER_INT_ON_MATCH(matchnum); 00296 } 00297 00298 /** 00299 * @brief For the specific match counter, enables reset of the terminal count register when a match occurs 00300 * @param pTMR : Pointer to timer IP register address 00301 * @param matchnum : Match timer, 0 to 3 00302 * @return Nothing 00303 */ 00304 STATIC INLINE void Chip_TIMER_ResetOnMatchEnable(LPC_TIMER_T *pTMR, int8_t matchnum) 00305 { 00306 pTMR->MCR |= TIMER_RESET_ON_MATCH(matchnum); 00307 } 00308 00309 /** 00310 * @brief For the specific match counter, disables reset of the terminal count register when a match occurs 00311 * @param pTMR : Pointer to timer IP register address 00312 * @param matchnum : Match timer, 0 to 3 00313 * @return Nothing 00314 */ 00315 STATIC INLINE void Chip_TIMER_ResetOnMatchDisable(LPC_TIMER_T *pTMR, int8_t matchnum) 00316 { 00317 pTMR->MCR &= ~TIMER_RESET_ON_MATCH(matchnum); 00318 } 00319 00320 /** 00321 * @brief Enable a match timer to stop the terminal count when a 00322 * match count equals the terminal count. 00323 * @param pTMR : Pointer to timer IP register address 00324 * @param matchnum : Match timer, 0 to 3 00325 * @return Nothing 00326 */ 00327 STATIC INLINE void Chip_TIMER_StopOnMatchEnable(LPC_TIMER_T *pTMR, int8_t matchnum) 00328 { 00329 pTMR->MCR |= TIMER_STOP_ON_MATCH(matchnum); 00330 } 00331 00332 /** 00333 * @brief Disable stop on match for a match timer. Disables a match timer 00334 * to stop the terminal count when a match count equals the terminal count. 00335 * @param pTMR : Pointer to timer IP register address 00336 * @param matchnum : Match timer, 0 to 3 00337 * @return Nothing 00338 */ 00339 STATIC INLINE void Chip_TIMER_StopOnMatchDisable(LPC_TIMER_T *pTMR, int8_t matchnum) 00340 { 00341 pTMR->MCR &= ~TIMER_STOP_ON_MATCH(matchnum); 00342 } 00343 00344 /** 00345 * @brief Enables capture on on rising edge of selected CAP signal for the 00346 * selected capture register, enables the selected CAPn.capnum signal to load 00347 * the capture register with the terminal coount on a rising edge. 00348 * @param pTMR : Pointer to timer IP register address 00349 * @param capnum : Capture signal/register to use 00350 * @return Nothing 00351 * @note <b>Special handling for timer 0</b><br> 00352 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00353 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00354 * slot 2). 00355 */ 00356 STATIC INLINE void Chip_TIMER_CaptureRisingEdgeEnable(LPC_TIMER_T *pTMR, int8_t capnum) 00357 { 00358 pTMR->CCR |= TIMER_CAP_RISING(capnum); 00359 } 00360 00361 /** 00362 * @brief Disables capture on on rising edge of selected CAP signal. For the 00363 * selected capture register, disables the selected CAPn.capnum signal to load 00364 * the capture register with the terminal coount on a rising edge. 00365 * @param pTMR : Pointer to timer IP register address 00366 * @param capnum : Capture signal/register to use 00367 * @return Nothing 00368 * @note <b>Special handling for timer 0</b><br> 00369 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00370 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00371 * slot 2). 00372 */ 00373 STATIC INLINE void Chip_TIMER_CaptureRisingEdgeDisable(LPC_TIMER_T *pTMR, int8_t capnum) 00374 { 00375 pTMR->CCR &= ~TIMER_CAP_RISING(capnum); 00376 } 00377 00378 /** 00379 * @brief Enables capture on on falling edge of selected CAP signal. For the 00380 * selected capture register, enables the selected CAPn.capnum signal to load 00381 * the capture register with the terminal coount on a falling edge. 00382 * @param pTMR : Pointer to timer IP register address 00383 * @param capnum : Capture signal/register to use 00384 * @return Nothing 00385 * @note <b>Special handling for timer 0</b><br> 00386 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00387 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00388 * slot 2). 00389 */ 00390 STATIC INLINE void Chip_TIMER_CaptureFallingEdgeEnable(LPC_TIMER_T *pTMR, int8_t capnum) 00391 { 00392 pTMR->CCR |= TIMER_CAP_FALLING(capnum); 00393 } 00394 00395 /** 00396 * @brief Disables capture on on falling edge of selected CAP signal. For the 00397 * selected capture register, disables the selected CAPn.capnum signal to load 00398 * the capture register with the terminal coount on a falling edge. 00399 * @param pTMR : Pointer to timer IP register address 00400 * @param capnum : Capture signal/register to use 00401 * @return Nothing 00402 * @note <b>Special handling for timer 0</b><br> 00403 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00404 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00405 * slot 2). 00406 */ 00407 STATIC INLINE void Chip_TIMER_CaptureFallingEdgeDisable(LPC_TIMER_T *pTMR, int8_t capnum) 00408 { 00409 pTMR->CCR &= ~TIMER_CAP_FALLING(capnum); 00410 } 00411 00412 /** 00413 * @brief Enables interrupt on capture of selected CAP signal. For the 00414 * selected capture register, an interrupt will be generated when the enabled 00415 * rising or falling edge on CAPn.capnum is detected. 00416 * @param pTMR : Pointer to timer IP register address 00417 * @param capnum : Capture signal/register to use 00418 * @return Nothing 00419 * @note <b>Special handling for timer 0</b><br> 00420 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00421 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00422 * slot 2). 00423 */ 00424 STATIC INLINE void Chip_TIMER_CaptureEnableInt(LPC_TIMER_T *pTMR, int8_t capnum) 00425 { 00426 pTMR->CCR |= TIMER_INT_ON_CAP(capnum); 00427 } 00428 00429 /** 00430 * @brief Disables interrupt on capture of selected CAP signal 00431 * @param pTMR : Pointer to timer IP register address 00432 * @param capnum : Capture signal/register to use 00433 * @return Nothing 00434 * @note <b>Special handling for timer 0</b><br> 00435 * For 16-bit and 32-bit timers 0, select channel 2 to set channel 1. For 16-bit and 00436 * 32-bit timers 1, select channel 1. (User manual designation of channel 1 is capture 00437 * slot 2). 00438 */ 00439 STATIC INLINE void Chip_TIMER_CaptureDisableInt(LPC_TIMER_T *pTMR, int8_t capnum) 00440 { 00441 pTMR->CCR &= ~TIMER_INT_ON_CAP(capnum); 00442 } 00443 00444 /** 00445 * @brief Standard timer initial match pin state and change state 00446 */ 00447 typedef enum IP_TIMER_PIN_MATCH_STATE { 00448 TIMER_EXTMATCH_DO_NOTHING = 0, /*!< Timer match state does nothing on match pin */ 00449 TIMER_EXTMATCH_CLEAR = 1, /*!< Timer match state sets match pin low */ 00450 TIMER_EXTMATCH_SET = 2, /*!< Timer match state sets match pin high */ 00451 TIMER_EXTMATCH_TOGGLE = 3 /*!< Timer match state toggles match pin */ 00452 } TIMER_PIN_MATCH_STATE_T; 00453 00454 /** 00455 * @brief Sets external match control (MATn.matchnum) pin control. For the pin 00456 * selected with matchnum, sets the function of the pin that occurs on 00457 * a terminal count match for the match count. 00458 * @param pTMR : Pointer to timer IP register address 00459 * @param initial_state : Initial state of the pin, high(1) or low(0) 00460 * @param matchState : Selects the match state for the pin 00461 * @param matchnum : MATn.matchnum signal to use 00462 * @return Nothing 00463 * @note For the pin selected with matchnum, sets the function of the pin that occurs on 00464 * a terminal count match for the match count. 00465 */ 00466 void Chip_TIMER_ExtMatchControlSet(LPC_TIMER_T *pTMR, int8_t initial_state, 00467 TIMER_PIN_MATCH_STATE_T matchState, int8_t matchnum); 00468 00469 /** 00470 * @brief Standard timer clock and edge for count source 00471 */ 00472 typedef enum IP_TIMER_CAP_SRC_STATE { 00473 TIMER_CAPSRC_RISING_PCLK = 0, /*!< Timer ticks on PCLK rising edge */ 00474 TIMER_CAPSRC_RISING_CAPN = 1, /*!< Timer ticks on CAPn.x rising edge */ 00475 TIMER_CAPSRC_FALLING_CAPN = 2, /*!< Timer ticks on CAPn.x falling edge */ 00476 TIMER_CAPSRC_BOTH_CAPN = 3 /*!< Timer ticks on CAPn.x both edges */ 00477 } TIMER_CAP_SRC_STATE_T; 00478 00479 /** 00480 * @brief Sets timer count source and edge with the selected passed from CapSrc. 00481 * If CapSrc selected a CAPn pin, select the specific CAPn pin with the capnum value. 00482 * @param pTMR : Pointer to timer IP register address 00483 * @param capSrc : timer clock source and edge 00484 * @param capnum : CAPn.capnum pin to use (if used) 00485 * @return Nothing 00486 * @note If CapSrc selected a CAPn pin, select the specific CAPn pin with the capnum value. 00487 */ 00488 STATIC INLINE void Chip_TIMER_TIMER_SetCountClockSrc(LPC_TIMER_T *pTMR, 00489 TIMER_CAP_SRC_STATE_T capSrc, 00490 int8_t capnum) 00491 { 00492 pTMR->CTCR = (uint32_t) capSrc | ((uint32_t) capnum) << 2; 00493 } 00494 00495 /** 00496 * @} 00497 */ 00498 00499 #ifdef __cplusplus 00500 } 00501 #endif 00502 00503 #endif /* __TIMER_11U6X_H_ */ 00504
Generated on Tue Jul 12 2022 21:03:52 by
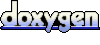