PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Embed:
(wiki syntax)
Show/hide line numbers
Synth.h
Go to the documentation of this file.
00001 /**************************************************************************/ 00002 /*! 00003 @file Synth.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Pokitto development stage library 00009 Software License Agreement 00010 00011 Copyright (c) 2015, Jonne Valola ("Author") 00012 All rights reserved. 00013 00014 This library is intended solely for the purpose of Pokitto development. 00015 00016 Redistribution and use in source and binary forms, with or without 00017 modification requires written permission from Author. 00018 */ 00019 /**************************************************************************/ 00020 00021 #ifndef SYNTH_H 00022 #define SYNTH_H 00023 00024 #include "Synth_osc.h " 00025 #include "Synth_song.h " 00026 00027 00028 /* PROPER WAY 00029 void f() {} 00030 00031 int main() 00032 { 00033 using FunctionPtr = void (*)(); 00034 00035 FunctionPtr ptr = f; 00036 } 00037 */ 00038 00039 #ifndef boolean 00040 typedef bool boolean; 00041 #endif 00042 00043 //extern void fakeISR(); // was defined in Rboy_soundsim.h 00044 00045 typedef void (*waveFunction)(OSC*); 00046 typedef void (*envFunction)(OSC*); 00047 typedef void (*mixFunction)(); 00048 00049 extern waveFunction Farr []; 00050 extern envFunction Earr []; 00051 extern mixFunction Marr []; // counts down 00052 extern mixFunction HWMarr []; // counts down 00053 /** COMMON TO BOTH HW AND SIM SOUND OUTPUT **/ 00054 00055 #define RBTRACKER_VERSION 0.03f 00056 00057 #define WOFF 0 00058 #define WSQUARE 1 00059 #define WSAW 2 00060 #define WTRI 3 00061 #define WNOISE 4 00062 #define WSAMPLE 5 00063 #define WPNOISE 5 00064 00065 #define OVERDRIVE 4 00066 00067 #define ARPSTEPMAX 4 // was 5 00068 #define PATTERNLENGTH 64 00069 #define MAXPATTERNS 10 00070 #define MAXBLOCKS 30 // 10 *3 00071 00072 #define VOLTICK 5 00073 #define ARPTICK 50 // 150 // was 200 00074 00075 #define NUMWAVES 5 00076 #define NUMENVELOPES 3 00077 #define NUMMIXES 4 00078 00079 extern void getNoteString(char *, uint8_t); 00080 00081 extern void playNote(uint8_t,uint8_t,uint8_t); 00082 extern void makeSampleInstruments(); 00083 00084 extern void setPitch(int); 00085 extern void setWave(int); 00086 extern void setVolume(int); 00087 extern void initAudio(); 00088 extern void testOsc(); 00089 extern void terminateSound(); 00090 extern void killSound(); 00091 extern void startSound(); 00092 extern void stopSound(); 00093 extern void updatePlayback(); // from flash 00094 extern void updatePlaybackSD(uint8_t); // from SD 00095 extern void initStreams(uint8_t); 00096 extern void emptyOscillators(); 00097 extern void emptyPatches(); 00098 extern void emptyBlocks(); 00099 extern void emptySong(); 00100 extern int openSongFromSD(char *); 00101 extern void writeChunkToSD(uint8_t *); 00102 extern void readChunkFromSD(uint8_t *); 00103 00104 00105 extern boolean playing, track1on, track2on, track3on, tableRefresh; 00106 extern uint16_t playerpos; 00107 extern uint16_t samplespertick, notetick; 00108 extern long samplesperpattern; 00109 00110 extern long readindex, writeindex; 00111 extern uint8_t tick, sequencepos; 00112 00113 extern SONG song; 00114 extern OSC osc1,osc2,osc3; 00115 extern OSC patch[]; 00116 extern BLOCK block[]; // array of blocks 00117 00118 #define MAX_ARPMODE 16 00119 00120 extern int8_t arptable[][5]; 00121 00122 extern uint16_t freqs[]; 00123 //extern uint16_t cincs[]; 00124 extern uint32_t cincs[]; 00125 00126 extern uint8_t xorshift8(); 00127 extern uint16_t xorshift16(); 00128 00129 extern uint16_t noiseval; 00130 00131 extern void setOSC(OSC*,byte, byte, byte, byte, byte, 00132 uint8_t, uint8_t, 00133 uint16_t, uint16_t, uint16_t, uint16_t, 00134 int16_t, int16_t, uint8_t, uint8_t, uint8_t); 00135 00136 extern void setOSC(OSC*,byte,byte,uint16_t, uint8_t, uint32_t); 00137 00138 extern void waveoff(OSC*); 00139 #endif // SYNTH_H 00140 00141
Generated on Tue Jul 12 2022 21:03:52 by
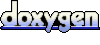