PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Embed:
(wiki syntax)
Show/hide line numbers
PokittoDisk.cpp
00001 /**************************************************************************/ 00002 /*! 00003 @file Pokitto_disk.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Pokitto development stage library 00009 Software License Agreement 00010 00011 Copyright (c) 2015, Jonne Valola ("Author") 00012 All rights reserved. 00013 00014 This library is intended solely for the purpose of Pokitto development. 00015 00016 Redistribution and use in source and binary forms, with or without 00017 modification requires written permission from Author. 00018 */ 00019 /**************************************************************************/ 00020 00021 #include "Pokitto.h " 00022 00023 #define SD_MOSI_PORT 0 00024 #define SD_MISO_PORT 0 00025 #define SD_SCK_PORT 0 00026 #define SD_CS_PORT 0 00027 #define SD_MOSI_PIN 9 00028 #define SD_MISO_PIN 8 00029 #define SD_SCK_PIN 6 00030 #define SD_CS_PIN 7 00031 00032 #if POK_ENABLE_SD > 0 00033 PFFS::BYTE res; 00034 PFFS::FATFS fs; /* File system object */ 00035 PFFS::FATDIR dir; /* Directory object */ 00036 PFFS::FILINFO fno; /* File information */ 00037 00038 //static FATFS *FatFs; /* Pointer to the file system object (logical drive) */ 00039 00040 bool diropened=false; 00041 00042 #define SPEAKER 3 00043 //#define BUFFER_SIZE 256 // was 128 00044 #define SONGLENGTH 0x1BFBCD // 1072223 00045 #define FILESIZE 0x1BFBCD 00046 00047 uint8_t filemode = FILE_MODE_UNINITIALIZED; 00048 char currentfile[15]; // holds current file's name 00049 00050 SPI device(CONNECT_MOSI,CONNECT_MISO,CONNECT_SCK); 00051 //DigitalOut mmccs(CONNECT_CS); 00052 00053 const char *get_filename_ext(const char *filename) { 00054 const char *dot = strrchr(filename, '.'); 00055 if(!dot || dot == filename) return ""; 00056 return dot + 1; 00057 } 00058 00059 __attribute__((section(".SD_Code"))) void initSDGPIO() { 00060 LPC_GPIO_PORT->DIR[SD_MOSI_PORT] |= (1 << SD_MOSI_PIN ); 00061 LPC_GPIO_PORT->DIR[SD_MISO_PORT] |= (1 << SD_MISO_PIN ); 00062 LPC_GPIO_PORT->DIR[SD_SCK_PORT] |= (1 << SD_SCK_PIN ); 00063 LPC_GPIO_PORT->DIR[SD_CS_PORT] |= (1 << SD_CS_PIN ); 00064 } 00065 00066 __attribute__((section(".SD_Code"))) int pokInitSD() { 00067 initSDGPIO(); 00068 res = PFFS::disk_initialize(); 00069 //res = disk_initialize(0); 00070 res = (pf_mount(&fs)); 00071 res = pf_opendir(&dir,""); 00072 if (res) diropened=false; 00073 else diropened=true; 00074 return res; 00075 } 00076 00077 00078 void emptyFname() { 00079 for (int i=0; i<13; i++) fno.fname[i]=NULL; 00080 } 00081 00082 /** PUBLIC FUNCTIONS **/ 00083 00084 char* getFirstDirEntry() { 00085 res=0; 00086 if (!diropened) { 00087 pokInitSD(); 00088 } 00089 res = pf_opendir(&dir,""); 00090 emptyFname(); 00091 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00092 if (res) return 0; 00093 while (res==0) { //while res is ok 00094 if ((fno.fattrib & 0x02)==0) { 00095 if (fno.fattrib & 0x10) { 00096 fno.fname[8]='.'; 00097 fno.fname[9]='D'; 00098 fno.fname[10]='I'; 00099 fno.fname[11]='R'; 00100 fno.fname[12]='\0'; 00101 } 00102 return fno.fname; 00103 } 00104 emptyFname(); 00105 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00106 if (res==0 && dir.index==0) break; 00107 } 00108 return 0; 00109 } 00110 00111 char* getNextDirEntry() { 00112 if (!diropened) pokInitSD(); 00113 emptyFname(); 00114 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00115 if (res==0) { 00116 while (fno.fattrib & 0x02 && !res) {emptyFname(); res = pf_readdir(&dir,&fno);} //system/hidden file 00117 if (fno.fattrib & 0x10) { 00118 int a=12; 00119 while (a) { 00120 fno.fname[a] = fno.fname[a-1]; 00121 a--; 00122 } 00123 if (fno.fname[0]) { 00124 fno.fname[0]='/'; 00125 a=0; 00126 while (fno.fname[a]) a++; 00127 fno.fname[a]='/'; 00128 } 00129 00130 /*fno.fname[a++]='.'; 00131 fno.fname[a++]='D'; 00132 fno.fname[a++]='I'; 00133 fno.fname[a++]='R'; 00134 fno.fname[a]='\0';*/ 00135 } 00136 return fno.fname; 00137 } 00138 return NULL; 00139 } 00140 00141 char* getNextFile (char* ext){ 00142 00143 if (!diropened) pokInitSD(); 00144 int a=1; 00145 emptyFname(); 00146 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00147 while (res==0 || a) { //while there are entries and 00148 if (dir.index==0) return 0; //end of list 00149 a = strcmp((const char*)get_filename_ext(fno.fname),(const char*)ext); // returns 0 if strings are identical 00150 if (strcmp(ext,"")==0 && (fno.fattrib & 0x10) == 0) a=0; 00151 if (a == 0 && (fno.fattrib & 0x10) == 0) return fno.fname; 00152 if (fno.fname[0]==NULL) return NULL; //end of files 00153 //if (fno.fattrib&0x10) return NULL; //its a directory 00154 emptyFname(); 00155 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00156 } 00157 return 0; 00158 } 00159 00160 00161 char* getNextFile() { 00162 return getNextFile(""); 00163 } 00164 00165 char* getFirstFile(char* ext) { 00166 res=0; 00167 if (!diropened) { 00168 pokInitSD(); 00169 } 00170 res = pf_opendir(&dir,""); 00171 emptyFname(); 00172 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00173 if (res) return 0; 00174 while (res==0 || (fno.fattrib & 0x10) == 0) { 00175 int a=0; 00176 a = strcmp((const char*)get_filename_ext(fno.fname),(const char*)ext); // returns 0 if strings are identical 00177 if (!strcmp(ext,"")) a=0; 00178 if ( a == 0 && (fno.fattrib & 0x10) == 0) return fno.fname; 00179 emptyFname(); 00180 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00181 if (fno.fname[0]==NULL) break; //end of directory reached, no files found 00182 if (res==0 && dir.index==0) break; 00183 } 00184 return 0; 00185 } 00186 00187 char* getFirstFile() { 00188 return getFirstFile(""); 00189 } 00190 00191 int isThisFileOpen(char* buffer){ 00192 int a=0; 00193 a = strcmp((const char*)buffer,(const char*)currentfile); // returns 0 if strings are identical 00194 if ( a == 0 && filemode != FILE_MODE_FAILED) return 1; 00195 return 0; 00196 } 00197 00198 int fileOK() { 00199 if (filemode != FILE_MODE_FAILED) return 1; 00200 return 0; 00201 } 00202 00203 uint8_t fileOpen(char* buffer, char fmode) { 00204 int err; 00205 if (filemode == FILE_MODE_UNINITIALIZED) { 00206 int a = pf_mount(&fs); 00207 if (a) return 1; // 1 means error in this context 00208 } 00209 00210 filemode = fmode; 00211 err = PFFS::pf_open(buffer); 00212 if (err==0) { 00213 strcpy(currentfile,(const char*)buffer); 00214 return 0; // 0 means all clear 00215 } 00216 // file open failed 00217 filemode = FILE_MODE_FAILED; 00218 return 1; // 1 means failed 00219 } 00220 00221 void fileClose() { 00222 filemode = FILE_MODE_UNINITIALIZED; 00223 for (uint8_t i=0; i<15; i++) currentfile[i]=0; 00224 } 00225 00226 char fileGetChar() { 00227 PFFS::BYTE buff[1]; 00228 PFFS::WORD br; 00229 int err = PFFS::pf_read(buff, 1, &br); /* Read data to the buff[] */ 00230 return buff[0]; 00231 } 00232 00233 void filePutChar(char c) { 00234 PFFS::WORD bw; 00235 PFFS::pf_write((const void*)&c, 1, &bw); 00236 PFFS::pf_write(0, 0, &bw); 00237 } 00238 00239 void fileWriteBytes(uint8_t * b, uint16_t n) { 00240 PFFS::WORD bw; 00241 PFFS::pf_write((const void*)&b, n, &bw); 00242 PFFS::pf_write(0, 0, &bw); 00243 } 00244 00245 uint16_t fileReadBytes(uint8_t * b, uint16_t n) { 00246 PFFS::WORD br; 00247 PFFS::pf_read(b, n, &br); /* Read data to the buff[] */ 00248 return br; /* Return number of bytes read */ 00249 } 00250 00251 void fileSeekAbsolute(long n) { 00252 res = PFFS::pf_lseek(n); 00253 } 00254 00255 void fileSeekRelative(long n) { 00256 if (n<0) if (fs.fptr < -n) n=-fs.fptr; 00257 else if (n>0) if (fs.fptr+n > fs.fsize) n=fs.fsize-fs.fptr; 00258 res = PFFS::pf_lseek(fs.fptr + n); 00259 } 00260 00261 void fileRewind() { 00262 res = PFFS::pf_lseek(0); 00263 } 00264 00265 void fileEnd() { 00266 res = PFFS::pf_lseek(fs.fsize); 00267 } 00268 00269 long int fileGetPosition() { 00270 return fs.fptr; 00271 } 00272 00273 uint8_t filePeek(long n) { 00274 PFFS::pf_lseek(n); 00275 return fileGetChar(); 00276 } 00277 00278 void filePoke(long n, uint8_t c) { 00279 PFFS::pf_lseek(n); 00280 filePutChar(c); 00281 } 00282 00283 int fileReadLine(char* destination, int maxchars) { 00284 int n=0; 00285 char c=1; 00286 char linebuf[80]; 00287 fileReadBytes((uint8_t*)linebuf,80); 00288 int index=0; 00289 while (c!=NULL) { 00290 c = linebuf[index++]; 00291 if (n == 0) { 00292 while (c == '\n' || c == '\r') c = linebuf[index++]; // skip empty lines 00293 } 00294 n++; 00295 if (c=='\n' || c=='\r' || n==maxchars-1) c=NULL; //prevent buffer overflow 00296 *destination++ = c; 00297 } 00298 fileSeekRelative(-80+index); //rewind 00299 return n; //number of characters read 00300 } 00301 00302 int dirOpen() { 00303 return PFFS::pf_opendir(&dir,""); 00304 } 00305 00306 int dirUp() { 00307 00308 return 0; 00309 } 00310 #endif // POK_ENABLE_SD 00311 00312 00313
Generated on Tue Jul 12 2022 21:03:51 by
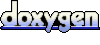