PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Embed:
(wiki syntax)
Show/hide line numbers
PokittoCore.h
Go to the documentation of this file.
00001 /**************************************************************************/ 00002 /*! 00003 @file PokittoCore.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #ifndef POKITTOCORE_H 00038 #define POKITTOCORE_H 00039 00040 #include <stdint.h> 00041 #include <math.h> 00042 #ifndef POK_SIM 00043 #include "pwmout_api.h" 00044 #include "HWButtons.h " 00045 #else 00046 #include "PokittoSimulator.h" 00047 #endif 00048 #if POK_USE_CONSOLE > 0 00049 #include "PokittoConsole.h " 00050 #endif // POK_USE_CONSOLE 00051 #if POK_ENABLE_SD > 0 00052 #include "PokittoDisk.h " 00053 #endif 00054 00055 #include "PokittoFonts.h " 00056 #include "PokittoPalettes.h" 00057 #include "PokittoDisplay.h " 00058 #include "PokittoButtons.h " 00059 #include "PokittoBattery.h " 00060 #include "PokittoBacklight.h " 00061 #include "PokittoSound.h " 00062 #include "PokittoFakeavr.h " 00063 00064 #define PALETTE_SIZE 256 00065 #undef PI 00066 #define PI 3.141592741f 00067 00068 // For GB compatibility 00069 #if PROJ_GAMEBUINO > 0 00070 extern void setup(); 00071 extern void loop(); 00072 #endif // PROJ_GAMEBUINO 00073 00074 extern uint32_t* ptimer; // re-directed tick counter 00075 00076 namespace Pokitto { 00077 00078 /** Core class. 00079 * The Core class is a class consisting of static data and methods. 00080 * It handles the lowlevel hardware functions of the Pokitto. 00081 * It is declared as static to prevent several instances running at same time. 00082 * Example: 00083 * @code 00084 * // A simple "Hello World!" program with Pokitto 00085 * 00086 * #include "Pokitto.h" 00087 * 00088 * Pokitto::Core myApp; 00089 * 00090 * int main() { 00091 * myApp.begin(); // This starts up the console (the display, buttons etc.) 00092 * while(myApp.isRunning()) { 00093 * if(myApp.Update()) { 00094 * myApp.display.print("Hello World!"); 00095 * } 00096 * } 00097 * } 00098 * @endcode 00099 */ 00100 00101 class Core 00102 { 00103 public: 00104 /** Create a Core runtime instance 00105 */ 00106 Core(); 00107 00108 /** Backlight component of the Core runtime */ 00109 static Backlight backlight; 00110 /** Buttons component of the Core runtime */ 00111 static Buttons buttons; 00112 /** Battery component of the Core runtime */ 00113 static Battery battery; 00114 /** Sound component of the Core runtime */ 00115 static Sound sound; 00116 /** Display component of the Core runtime */ 00117 static Display display; 00118 00119 // EXCECUTION CONTROL 00120 public: 00121 /** Initialize runtime (use this one) */ 00122 static void begin(); 00123 /** Initialize runtime (deprecated, avoid) */ 00124 static void init(); 00125 /** Initialize runtime with options (deprecated, avoid) */ 00126 static void init(uint8_t); 00127 /** Return run state (1 = running, 0 = shutting down) */ 00128 static bool isRunning(); 00129 /** Stop running */ 00130 static void quit(); 00131 //private: 00132 /** run_state is true as long as program is running */ 00133 static bool run_state; 00134 00135 public: 00136 // INITIALIZATION 00137 /** Initialize display */ 00138 static void initDisplay(); 00139 /** Initialize random generator */ 00140 static void initRandom(); 00141 /** Initialize GPIO */ 00142 static void initGPIO(); 00143 /** Initialize LCD */ 00144 static void initLCD(); 00145 /** Initialize Audio */ 00146 static void initAudio(); 00147 00148 00149 // DISPLAY 00150 public: 00151 /** Initialize backlight */ 00152 static void initBacklight(); 00153 00154 private: 00155 /** Backlight PWM pointer */ 00156 #ifndef POK_SIM 00157 static pwmout_t backlightpwm; 00158 #endif 00159 00160 // TIMEKEEPING 00161 public: 00162 /** Initialize runtime clock */ 00163 static void initClock(); 00164 /** Get value of time elapsed during program in milliseconds */ 00165 static uint32_t getTime(); 00166 /** Wait for n milliseconds */ 00167 static void wait(uint16_t); 00168 /** FPS */ 00169 static uint32_t fps_counter; 00170 static bool fps_counter_updated; 00171 private: 00172 /** Time of next refresh */ 00173 static uint32_t refreshtime; 00174 00175 // DIRECT TO SCREEN 00176 public: 00177 /** Display Pokitto logo */ 00178 static void showLogo(); 00179 static void showWarning(); 00180 static void setVolLimit(); 00181 00182 // BUTTON INPUT HANDLING 00183 public: 00184 static void initButtons(); 00185 static void pollButtons(); 00186 static uint8_t leftBtn(); 00187 static uint8_t rightBtn(); 00188 static uint8_t upBtn(); 00189 static uint8_t downBtn(); 00190 static uint8_t aBtn(); 00191 static uint8_t bBtn(); 00192 static uint8_t cBtn(); 00193 static uint8_t leftHeld(); 00194 static uint8_t rightHeld(); 00195 static uint8_t upHeld(); 00196 static uint8_t downHeld(); 00197 static uint8_t aHeld(); 00198 static uint8_t bHeld(); 00199 static uint8_t cHeld(); 00200 00201 static uint8_t leftReleased(); 00202 static uint8_t rightReleased(); 00203 static uint8_t upReleased(); 00204 static uint8_t downReleased(); 00205 static uint8_t aReleased(); 00206 static uint8_t bReleased(); 00207 static uint8_t cReleased(); 00208 00209 // AUDIO RELATED 00210 static uint8_t ampIsOn(); 00211 static void ampEnable(uint8_t); 00212 static uint8_t soundbyte; 00213 00214 // GB RELATED 00215 public: 00216 static void readSettings(); 00217 static void titleScreen(const char* name, const uint8_t *logo); 00218 static void titleScreen(const char* name); 00219 static void titleScreen(const uint8_t* logo); 00220 static void titleScreen(); 00221 static bool update(bool useDirectMode=false, uint8_t updRectX=0, uint8_t updRectY=0, uint8_t updRectW=LCDWIDTH, uint8_t updRectH=LCDHEIGHT); 00222 static uint32_t frameCount; 00223 static int8_t menu(const char* const* items, uint8_t length); 00224 static char* filemenu(char*); 00225 static char* filemenu(); 00226 static void keyboard(char* text, uint8_t length); 00227 static void popup(const char* text, uint8_t duration); 00228 static void setFrameRate(uint8_t fps); 00229 static void pickRandomSeed(); 00230 00231 static uint8_t getCpuLoad(); 00232 static uint16_t getFreeRam(); 00233 00234 static bool collidePointRect(int16_t x1, int16_t y1 ,int16_t x2 ,int16_t y2, int16_t w, int16_t h); 00235 static bool collideRectRect(int16_t x1, int16_t y1, int16_t w1, int16_t h1 ,int16_t x2 ,int16_t y2, int16_t w2, int16_t h2); 00236 static bool collideBitmapBitmap(int16_t x1, int16_t y1, const uint8_t* b1, int16_t x2, int16_t y2, const uint8_t* b2); 00237 00238 private: 00239 static uint8_t timePerFrame; 00240 static uint32_t nextFrameMillis; 00241 static void updatePopup(); 00242 static const char* popupText; 00243 static uint8_t popupTimeLeft; 00244 static void displayBattery(); 00245 static uint16_t frameDurationMicros; 00246 static uint32_t frameStartMicros, frameEndMicros; 00247 static uint8_t startMenuTimer; 00248 static int updateLoader(uint32_t,uint32_t); 00249 static uint32_t fps_refreshtime; 00250 static uint32_t fps_frameCount; 00251 00252 public: 00253 static uint8_t volbar_visible; 00254 static void drawvolbar(int,int,int, bool); 00255 static void askLoader(); 00256 static void jumpToLoader(); 00257 }; 00258 00259 // this is the instance used by the system 00260 extern Core core; 00261 00262 00263 } 00264 00265 #endif // POKITTOCORE_H 00266 00267 00268
Generated on Tue Jul 12 2022 21:03:51 by
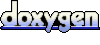