PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Embed:
(wiki syntax)
Show/hide line numbers
PokittoButtons.cpp
Go to the documentation of this file.
00001 /**************************************************************************/ 00002 /*! 00003 @file PokittoButtons.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #include "PokittoCore.h " 00038 00039 using namespace Pokitto; 00040 00041 uint8_t Buttons::pins[NUM_BTN]; 00042 uint8_t Buttons::states[NUM_BTN]; 00043 uint8_t Buttons::buttons_state; 00044 uint8_t Buttons::buttons_held; 00045 uint8_t Buttons::buttons_released; // from LSB up,down,left,right,a,b,c 00046 uint16_t Buttons::cHWLongPress = CHWLONGPRESSTIMEOUT; 00047 00048 00049 void Buttons::begin() { 00050 #ifndef POK_SIM 00051 Pokitto::initButtons(); 00052 #endif // POK_SIM 00053 } 00054 00055 void Buttons::update() { 00056 #if POK_USE_CONSOLE 00057 if (console.conscounter) return; 00058 #endif // POK_USE_CONSOLE 00059 #ifndef POK_SIM 00060 /** HARDWARE CODE **/ 00061 for (uint8_t thisButton = 0; thisButton < NUM_BTN; thisButton++) { 00062 if (Pokitto::heldStates[thisButton]) { //if button pressed 00063 states[thisButton]++; //increase button hold time 00064 if (states[thisButton]==0xFF) states[thisButton]=2; // PREVENT WRAPAROUND!!!! 00065 } else { 00066 if (states[thisButton] == 0)//button idle 00067 continue; 00068 if (states[thisButton] == 0xFF)//if previously released 00069 states[thisButton] = 0; //set to idle 00070 else 00071 states[thisButton] = 0xFF; //button just released 00072 } 00073 } 00074 #else 00075 /** POK_SIM code **/ 00076 simulator.pollButtons(); 00077 for (uint8_t thisButton = 0; thisButton < NUM_BTN; thisButton++) { 00078 uint8_t temp=0; 00079 switch (thisButton) { 00080 case 0: 00081 temp = simulator.leftHeld(); break; 00082 case 1: 00083 temp = simulator.upHeld(); break; 00084 case 2: 00085 temp = simulator.rightHeld(); break; 00086 case 3: 00087 temp = simulator.downHeld(); break; 00088 case 4: 00089 temp = simulator.aHeld(); break; 00090 case 5: 00091 temp = simulator.bHeld(); break; 00092 case 6: 00093 temp = simulator.cHeld(); break; 00094 default: 00095 break; 00096 } 00097 00098 if (temp == HIGH) { //if button pressed 00099 states[thisButton]++; //increase button hold time 00100 if (states[thisButton]==0xFF) states[thisButton] = 2; //prevent wraparound and retrigger 00101 } else { 00102 if (states[thisButton] == 0)//button idle 00103 continue; 00104 if (states[thisButton] == 0xFF)//if previously released 00105 states[thisButton] = 0; //set to idle 00106 else 00107 states[thisButton] = 0xFF; //button just released 00108 } 00109 } 00110 00111 #endif // POK_SIM 00112 } 00113 00114 /* 00115 * Returns true when 'button' is pressed. 00116 * The button has to be released for it to be triggered again. 00117 */ 00118 bool Buttons::pressed(uint8_t button) { 00119 if (states[button] == 1) 00120 return true; 00121 else 00122 return false; 00123 } 00124 00125 /* 00126 * return true if 'button' is released 00127 */ 00128 bool Buttons::released(uint8_t button) { 00129 if (states[button] == 0xFF) 00130 return true; 00131 else 00132 return false; 00133 } 00134 00135 /** 00136 * returns true ONCE when 'button' is held for 'time' frames 00137 * @param button The button's ID 00138 * @param time How much frames button must be held, between 1 and 254. 00139 * @return true when 'button' is held for 'time' frames 00140 */ 00141 bool Buttons::held(uint8_t button, uint8_t time){ 00142 if(states[button] == (time+1)) 00143 return true; 00144 else 00145 return false; 00146 } 00147 00148 /** 00149 * returns true every 'period' frames when 'button' is held 00150 * @param button The button's ID 00151 * @param period How much frames button must be held, between 1 and 254. 00152 * @return true if the button is held for the given time 00153 */ 00154 bool Buttons::repeat(uint8_t button, uint8_t period) { 00155 if (period <= 1) { 00156 if ((states[button] != 0xFF) && (states[button])) 00157 return true; 00158 } else { 00159 if ((states[button] != 0xFF) && ((states[button] % period) == 1)) 00160 return true; 00161 } 00162 return false; 00163 } 00164 00165 /** 00166 * 00167 * @param button The button's ID 00168 * @return The number of frames during which the button has been held. 00169 */ 00170 uint8_t Buttons::timeHeld(uint8_t button){ 00171 if(states[button] != 0xFF) 00172 return states[button]; 00173 else 00174 return 0; 00175 00176 } 00177 00178 void Buttons::pollButtons() { 00179 #ifdef POK_SIM 00180 simulator.pollButtons(); 00181 #else 00182 uint8_t buttons_state_old = buttons_state; 00183 buttons_state = 0; // clear all 00184 if (upBtn()) buttons_state |= (1<<UPBIT); 00185 if (downBtn()) buttons_state |= (1<<DOWNBIT); 00186 if (leftBtn()) buttons_state |= (1<<LEFTBIT); 00187 if (rightBtn()) buttons_state |= (1<<RIGHTBIT); 00188 if (aBtn()) buttons_state |= (1<<ABIT); 00189 if (bBtn()) buttons_state |= (1<<BBIT); 00190 if (cBtn()) buttons_state |= (1<<CBIT); 00191 buttons_held = buttons_state & buttons_state_old; // only if both 1, end result is 1 00192 buttons_released = ~buttons_state & buttons_state_old; // if now zero, then 1 AND previous 1 = 1 00193 #endif // POK_SIM 00194 } 00195 00196 uint8_t Buttons::aBtn() { 00197 #ifdef POK_SIM 00198 return simulator.aBtn(); 00199 #else 00200 return Pokitto::heldStates[BTN_A]; 00201 #endif // POK_SIM 00202 } 00203 00204 00205 uint8_t Buttons::bBtn() { 00206 #ifdef POK_SIM 00207 return simulator.bBtn(); 00208 #else 00209 return Pokitto::heldStates[BTN_B]; 00210 #endif // POK_SIM 00211 } 00212 00213 uint8_t Buttons::cBtn() { 00214 uint8_t c; 00215 #ifdef POK_SIM 00216 c = simulator.cBtn(); 00217 #else 00218 c = Pokitto::heldStates[BTN_C]; 00219 #endif // POK_SIM 00220 return c; 00221 } 00222 00223 uint8_t Buttons::leftBtn() { 00224 #ifdef POK_SIM 00225 return simulator.leftBtn(); 00226 #else 00227 return Pokitto::heldStates[BTN_LEFT]; 00228 #endif // POK_SIM 00229 } 00230 00231 uint8_t Buttons::rightBtn() { 00232 #ifdef POK_SIM 00233 return simulator.rightBtn(); 00234 #else 00235 return Pokitto::heldStates[BTN_RIGHT]; 00236 #endif // POK_SIM 00237 } 00238 00239 uint8_t Buttons::upBtn() { 00240 #ifdef POK_SIM 00241 return simulator.upBtn(); 00242 #else 00243 return Pokitto::heldStates[BTN_UP]; 00244 #endif // POK_SIM 00245 } 00246 00247 uint8_t Buttons::downBtn() { 00248 #ifdef POK_SIM 00249 return simulator.downBtn(); 00250 #else 00251 return Pokitto::heldStates[BTN_DOWN]; 00252 #endif // POK_SIM 00253 } 00254 00255 00256 00257 00258 00259 00260 00261 00262 //** EOF **// 00263
Generated on Tue Jul 12 2022 21:03:51 by
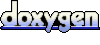