PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Fork of PokittoLib by
SoftwareI2C.cpp
00001 /* 00002 * mbed Library to use a software master i2c interface on any GPIO pins 00003 * Copyright (c) 2012 Christopher Pepper 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 #include "SoftwareI2C.h" 00008 00009 /** 00010 * @brief Initializes interface 00011 * @param sda GPIO pin to use as I2C SDA 00012 * @param scl GPIO pin to use as I2C SCL 00013 */ 00014 00015 SoftwareI2C::SoftwareI2C(PinName sda, PinName scl) : _sda(sda) , _scl(scl) { 00016 _scl.output(); 00017 _scl.mode(OpenDrain); 00018 _sda.output(); 00019 _sda.mode(OpenDrain); 00020 00021 _device_address = 0; 00022 _frequency_delay = 1; 00023 00024 initialise(); 00025 } 00026 00027 SoftwareI2C::~SoftwareI2C() { 00028 00029 } 00030 00031 /** 00032 * @brief Read 1 or more bytes from the I2C slave 00033 * @param device_address The address of the device to read from 00034 * @param data An allocated array to read the data into 00035 * @param data_bytes Number of bytes to read (must be equal to or less then the allocated memory in data) 00036 */ 00037 void SoftwareI2C::read(uint8_t device_address, uint8_t* data, uint8_t data_bytes) { 00038 if (data == 0 || data_bytes == 0) return; 00039 00040 device_address = device_address | 0x01; 00041 start(); 00042 putByte(device_address); 00043 getAck(); 00044 for (int x = 0; x < data_bytes; ++x) { 00045 data[x] = getByte(); 00046 if ( x < (data_bytes -1)) { //ack all but the final byte 00047 giveAck(); 00048 } 00049 } 00050 stop(); 00051 } 00052 00053 /** 00054 * @brief Write 1 or more bytes to the I2C slave 00055 * @param device_address The address of the device to write to 00056 * @param data An array to write the data from 00057 * @param data_bytes Number of bytes to write from array 00058 */ 00059 void SoftwareI2C::write(uint8_t device_address, uint8_t* data, uint8_t data_bytes) { 00060 if (data == 0 || data_bytes == 0) return; 00061 00062 device_address = device_address & 0xFE; 00063 start(); 00064 putByte(device_address); 00065 getAck(); 00066 for ( int x = 0; x < data_bytes; ++x ) { 00067 putByte(data[x]); 00068 getAck(); 00069 } 00070 stop(); 00071 } 00072 00073 /** 00074 * @brief Write 1 byte to the I2C slave 00075 * @param device_address The address of the device to write to 00076 * @param byte The data to write 00077 */ 00078 void SoftwareI2C::write(uint8_t device_address, uint8_t byte) { 00079 device_address = device_address & 0xFE; 00080 start(); 00081 putByte(device_address); 00082 getAck(); 00083 putByte(byte); 00084 getAck(); 00085 stop(); 00086 } 00087 00088 /** 00089 * @brief Read 1 or more bytes from the I2C slave at the specified memory address 00090 * @param device_address The address of the device to read from 00091 * @param start_address The memory address to read from 00092 * @param data The allocated array to read into 00093 * @param data_bytes The number of bytes to read 00094 */ 00095 void SoftwareI2C::randomRead(uint8_t device_address, uint8_t start_address, uint8_t* data, uint8_t data_bytes) { 00096 if (data == 0 || data_bytes == 0) return; 00097 00098 device_address = device_address & 0xFE; 00099 start(); 00100 putByte(device_address); 00101 if (!getAck()) { 00102 return; 00103 } 00104 putByte(start_address); 00105 if (!getAck()) { 00106 return; 00107 } 00108 00109 device_address=device_address | 0x01; 00110 start(); 00111 putByte(device_address); 00112 if (!getAck()) { 00113 return; 00114 } 00115 for ( int x = 0; x < data_bytes; ++x) { 00116 data[x] = getByte(); 00117 if (x != (data_bytes - 1)) giveAck(); 00118 } 00119 stop(); 00120 } 00121 00122 /** 00123 * @brief Write 1 byte to the I2C slave at the specified memory address 00124 * @param device_address The address of the device to write to 00125 * @param start_address The memory address to write to 00126 * @param byte The data to write 00127 */ 00128 void SoftwareI2C::randomWrite(uint8_t device_address, uint8_t start_address, uint8_t byte) { 00129 device_address = device_address & 0xFE; 00130 start(); 00131 putByte(device_address); 00132 getAck(); 00133 putByte(start_address); 00134 getAck(); 00135 putByte(byte); 00136 getAck(); 00137 stop(); 00138 } 00139 00140 /** 00141 * @brief Write 1 or more bytes to the I2C slave at the specified memory address 00142 * @param device_address The address of the device to write to 00143 * @param start_address The memory address to write to 00144 * @param data The data to write 00145 * @param data_bytes The number of bytes to write 00146 */ 00147 void SoftwareI2C::randomWrite(uint8_t device_address, uint8_t start_address, uint8_t* data, uint8_t data_bytes) { 00148 if (data == 0 || data_bytes == 0) return; 00149 00150 device_address = device_address & 0xFE; 00151 start(); 00152 putByte(device_address); 00153 getAck(); 00154 putByte(start_address); 00155 getAck(); 00156 for ( int x = 0; x <= data_bytes; ++x ) { 00157 putByte(data[x]); 00158 getAck(); 00159 } 00160 stop(); 00161 } 00162 00163 /** 00164 * @brief Read 2 bytes from the I2C slave at the specified memory address and return them as an 16bit unsigned integer 00165 * @param device_address The address of the device to read from 00166 * @param start_address The memory address to read from 00167 * @return MSB 16bit unsigned integer 00168 */ 00169 uint16_t SoftwareI2C::read16(uint8_t device_address, uint8_t start_address) { 00170 uint8_t short_array[2] = {0, 0}; 00171 randomRead(device_address, start_address, short_array, 2 ); 00172 uint16_t value = 0; 00173 value = short_array[0] << 8; 00174 value |= short_array[1]; 00175 00176 return value; 00177 } 00178 00179 /** 00180 * @brief Read 3 bytes from the I2C slave at the specified memory address and return them as an 32bit unsigned integer 00181 * @param device_address The address of the device to read from 00182 * @param start_address The memory address to read from 00183 * @return MSB 32bit unsigned integer 00184 */ 00185 uint32_t SoftwareI2C::read24(uint8_t device_address, uint8_t start_address) { 00186 uint8_t value_array[4] = {0, 0, 0}; 00187 randomRead(device_address, start_address, value_array, 3 ); 00188 uint32_t value = 0; 00189 value = value_array[0] << 16; 00190 value |= value_array[1] << 8; 00191 value |= value_array[2]; 00192 00193 return value; 00194 } 00195 00196 /** 00197 * @brief Read 4 bytes from the I2C slave at the specified memory address and return them as an 32bit unsigned integer 00198 * @param device_address The address of the device to read from 00199 * @param start_address The memory address to read from 00200 * @return MSB 32bit unsigned integer 00201 */ 00202 uint32_t SoftwareI2C::read32(uint8_t device_address, uint8_t start_address) { 00203 uint8_t value_array[4] = {0, 0, 0, 0}; 00204 randomRead(device_address, start_address, value_array, 4 ); 00205 uint32_t value = 0; 00206 value = value_array[0] << 24; 00207 value |= value_array[1] << 16; 00208 value |= value_array[2] << 8; 00209 value |= value_array[3]; 00210 00211 return value; 00212 } 00213
Generated on Tue Jul 12 2022 18:08:13 by
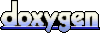