PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Fork of PokittoLib by
Pokitto_settings.h
00001 /**************************************************************************/ 00002 /*! 00003 @file Pokitto_settings.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 00038 #ifndef POKITTO_SETTINGS_H 00039 #define POKITTO_SETTINGS_H 00040 00041 #include "My_settings.h" 00042 00043 #ifdef PROJ_BOARDREV 00044 #define POK_BOARDREV PROJ_BOARDREV // which revision of Pokitto board 00045 #else 00046 #define POK_BOARDREV 2 // default is boardrev 2 (the 4-layer board) 00047 #endif 00048 00049 /** LOGO */ 00050 #ifdef PROJ_STARTUPLOGO 00051 #define POK_DISPLAYLOGO PROJ_STARTUPLOGO// if enabled, show logo at start 00052 #else 00053 #define POK_DISPLAYLOGO 1 00054 #endif 00055 00056 #define POK_ENABLE_REFRESHWITHWAIT 0 // choose whether waiting in application refreshes display or not 00057 #define POK_ENABLE_FPSCOUNTER 0 // turn off to save some cpu 00058 #define POK_ENABLE_SD 1 // Define true to include SD library 00059 #define POK_LOADER_COUNTDOWN 3 //how many seconds waiting for C press for loader 00060 00061 #ifndef PROJ_ENABLE_SOUND 00062 #define POK_ENABLE_SOUND 1 00063 #else 00064 #define POK_ENABLE_SOUND PROJ_ENABLE_SOUND 00065 #endif 00066 00067 #ifndef PROJ_GBSOUND 00068 #if POK_ENABLE_SOUND > 0 00069 #define POK_GBSOUND 0 00070 #endif 00071 #else 00072 #define POK_GBSOUND PROJ_GBSOUND 00073 #endif 00074 00075 00076 #ifndef PROJ_STREAMING_MUSIC 00077 #if POK_ENABLE_SOUND > 0 00078 #define POK_STREAMING_MUSIC 1 // Define true to stream music from SD 00079 #endif 00080 #else 00081 #define POK_STREAMING_MUSIC PROJ_STREAMING_MUSIC 00082 #endif // PROJ_STREAMING_MUSIC 00083 00084 #ifndef PROJ_ENABLE_SYNTH 00085 #define POK_ENABLE_SYNTH 0 00086 #else 00087 #define POK_ENABLE_SYNTH PROJ_ENABLE_SYNTH 00088 #endif // PROJ_ENABLE_SYNTH 00089 00090 00091 /** CONSOLE **/ 00092 #define POK_USE_CONSOLE 1 //if debugging console is available or not 00093 #define POK_CONSOLE_VISIBLE_AT_STARTUP 1 // whaddaya think ? 00094 #define POK_CONSOLE_INTERVAL 1000 // interval in ms how often console is drawn 00095 #if POK_USE_CONSOLE > 0 // this prevents trying to log messages if console is disabled 00096 #define POK_CONSOLE_LOG_BUTTONS 0 // if console logs keypresses 00097 #define POK_CONSOLE_LOG_COLLISIONS 1 // if console logs collisions 00098 #endif // POK_USE_CONSOLE 00099 #define CONSOLEBUFSIZE 20 00100 #define POK_SHOW_VOLUME 0 // volumebar drawn after console if enabled 00101 #define VOLUMEBAR_TIMEOUT 10 // frames before disappearing 00102 00103 /** PROJECT LIBRARY TYPE **/ 00104 // Tiled mode can NOT be buffered mode (fast mode, arduboy mode, gamebuino mode etc) 00105 #if PROJ_TILEDMODE > 0 00106 #define POK_TILEDMODE 1 00107 #ifdef PROJ_TILEWIDTH 00108 #define POK_TILE_W PROJ_TILEWIDTH 00109 #else 00110 #define POK_TILE_W 11 00111 #endif // PROJ_TILEWIDTH 00112 #if POK_TILE_W == 11 00113 #define POK_TILES_X 20 00114 #define LCDWIDTH 220 00115 #elif POK_TILE_W == 12 00116 #define POK_TILES_X 18 00117 #define LCDWIDTH 216 00118 #elif POK_TILE_W == 8 00119 #define POK_TILES_X 27 00120 #define LCDWIDTH 216 00121 #elif POK_TILE_W == 32 00122 #define POK_TILES_X 6 00123 #define LCDWIDTH 220 00124 #elif POK_TILE_W == 10 00125 #define POK_TILES_X 22 00126 #define LCDWIDTH 220 00127 #elif POK_TILE_W == 14 00128 #define POK_TILES_X 15 00129 #define LCDWIDTH 210 00130 #endif 00131 #ifdef PROJ_TILEHEIGHT 00132 #define POK_TILE_H PROJ_TILEHEIGHT 00133 #else 00134 #define POK_TILE_H 11 00135 #endif // PROJ_TILEHEIGHT 00136 #if POK_TILE_H == 11 00137 #define POK_TILES_Y 16 00138 #define LCDHEIGHT 176 00139 #elif POK_TILE_H == 12 00140 #define POK_TILES_Y 14 00141 #define LCDHEIGHT 168 00142 #elif POK_TILE_H == 8 00143 #define POK_TILES_Y 22 00144 #define LCDHEIGHT 176 00145 #elif POK_TILE_H == 32 00146 #define POK_TILES_Y 5 00147 #define LCDHEIGHT 176 00148 #elif POK_TILE_H == 10 00149 #define POK_TILES_Y 17 00150 #define LCDHEIGHT 170 00151 #elif POK_TILE_H == 14 00152 #define POK_TILES_Y 12 00153 #define LCDHEIGHT 168 00154 #endif 00155 #else 00156 #if PROJ_GAMEBUINO > 0 00157 #define POK_GAMEBUINO_SUPPORT PROJ_GAMEBUINO // Define true to support Gamebuino library calls 00158 #define PROJ_SCREENMODE MODE_GAMEBUINO_16COLOR 00159 #define POK_STRETCH 1 00160 #define PICOPALETTE 0 00161 #define POK_COLORDEPTH 4 00162 #else 00163 #if PROJ_ARDUBOY > 0 00164 #define POK_ARDUBOY_SUPPORT PROJ_ARDUBOY // Define true to support Arduboy library calls 00165 #define PROJ_SCREENMODE MODE_ARDUBOY_16COLOR 00166 #define POK_COLORDEPTH 1 00167 #define POK_STRETCH 1 00168 #define POK_FPS 20 00169 #define PICOPALETTE 0 00170 #else 00171 #if PROJ_RBOY > 0 00172 #define PROJ_SCREENMODE MODE_GAMEBUINO_16COLOR 00173 #define POK_COLORDEPTH 1 00174 #define POK_STRETCH 0 00175 #define POK_FPS 40 00176 #define PICOPALETTE 0 00177 #else 00178 #if PROJ_GAMEBOY > 0 00179 #define PROJ_SCREENMODE MODE_GAMEBOY 00180 #define POK_COLORDEPTH 2 00181 #define POK_STRETCH 0 00182 #define POK_FPS 6 00183 #define PICOPALETTE 0 00184 #else 00185 #define POK_GAMEBUINO_SUPPORT 0 00186 #define POK_GAMEBOY_SUPPORT 0 00187 #define POK_ARDUBOY_SUPPORT 0 00188 #define PICOPALETTE 0 00189 #define POK_COLORDEPTH 4 00190 #endif // PROJ_GAMEBOY 00191 #endif // PROJ_RBOY 00192 #endif // PROJ_ARDUBOY 00193 #endif // PROJ_GAMEBUINO 00194 #endif // PROJ_TILEDMODE 00195 00196 /** SCREEN MODES TABLE -- DO NOT CHANGE THESE **/ 00197 00198 #define POK_LCD_W 220 //<- do not change !! 00199 #define POK_LCD_H 176 //<- do not change !! 00200 00201 #define MODE_NOBUFFER 0 //Size: 0 00202 #define BUFSIZE_NOBUFFER 0 00203 #define MODE_HI_4COLOR 1 //Size: 9680 00204 #define BUFSIZE_HI_4 9680 00205 #define MODE_FAST_16COLOR 2 //Size: 4840 00206 #define BUFSIZE_FAST_16 4840 00207 #define MODE_HI_16COLOR 3 00208 #define BUFSIZE_HI_16 19360 00209 #define MODE_GAMEBUINO_16COLOR 4 //Size: 2016 00210 #define BUFSIZE_GAMEBUINO_16 2016 00211 #define MODE_ARDUBOY_16COLOR 5 //Size: 4096 00212 #define BUFSIZE_ARDUBOY_16 4096 00213 #define MODE_HI_MONOCHROME 6 //Size: 4840 00214 #define BUFSIZE_HI_MONO 4840 00215 #define MODE_HI_GRAYSCALE 7 //Size: 9680 00216 #define BUFSIZE_HI_GS 9680 00217 #define MODE_GAMEBOY 8 00218 #define BUFSIZE_GAMEBOY 5760 00219 #define MODE_UZEBOX 9 00220 #define MODE_TVOUT 10 00221 #define MODE_LAMENES 11 00222 #define BUFSIZE_LAMENES 7680 00223 #define MODE_256_COLOR 12 00224 #define BUFSIZE_MODE_12 4176 // 72 x 58 00225 // Tiled modes 00226 #define MODE_TILED_1BIT 1001 00227 #define MODE_TILED_8BIT 1002 00228 00229 00230 00231 /** SCREENMODE - USE THIS SELECTION FOR YOUR PROJECT **/ 00232 00233 #if POK_TILEDMODE > 0 00234 #ifndef PROJ_TILEBITDEPTH 00235 #define PROJ_TILEBITDEPTH 8 //default tiling mode is 256 color mode! 00236 #endif // PROJ_TILEBITDEPTH 00237 #if PROJ_TILEBITDEPTH == 1 00238 #define POK_SCREENMODE MODE_TILED_1BIT 00239 #define POK_COLORDEPTH 1 00240 #else 00241 #define POK_SCREENMODE MODE_TILED_8BIT 00242 #define POK_COLORDEPTH 8 00243 #endif // PROJ_TILEBITDEPTH 00244 #else 00245 #ifndef PROJ_SCREENMODE 00246 #undef POK_COLORDEPTH 00247 #ifdef PROJ_HIRES 00248 #if PROJ_HIRES > 0 00249 #define POK_SCREENMODE MODE_HI_4COLOR 00250 #undef POK_COLORDEPTH 00251 #define POK_COLORDEPTH 2 00252 #elif PROJ_HICOLOR > 0 00253 #define POK_SCREENMODE MODE_256_COLOR 00254 #undef POK_COLORDEPTH 00255 #define POK_COLORDEPTH 8 00256 #else 00257 #define POK_SCREENMODE MODE_FAST_16COLOR 00258 #undef POK_COLORDEPTH 00259 #define POK_COLORDEPTH 4 00260 #endif // PROJ_HIRES 00261 #else 00262 #define POK_SCREENMODE MODE_FAST_16COLOR 00263 #define POK_COLORDEPTH 4 00264 #endif // PROJ_HIRES 00265 #else 00266 #define POK_SCREENMODE PROJ_SCREENMODE 00267 #endif 00268 #endif // POK_TILEDMODE 00269 00270 /* DEFINE SCREENMODE AS THE MAXIMUM SCREEN SIZE NEEDED BY YOUR APP ... SEE SIZES LISTED ABOVE */ 00271 00272 /** AUTOMATIC COLOR DEPTH SETTING - DO NOT CHANGE **/ 00273 #ifndef POK_COLORDEPTH 00274 #define POK_COLORDEPTH 4 // 1...5 is valid 00275 #endif // POK_COLORDEPTH 00276 00277 /** AUTOMATIC SCREEN BUFFER SIZE CALCULATION - DO NOT CHANGE **/ 00278 #if POK_SCREENMODE == 0 00279 #define POK_SCREENBUFFERSIZE 0 00280 #define LCDWIDTH POK_LCD_W 00281 #define LCDHEIGHT POK_LCD_H 00282 #define POK_BITFRAME 0 00283 #elif POK_SCREENMODE == MODE_HI_MONOCHROME 00284 #define POK_SCREENBUFFERSIZE POK_LCD_W*POK_LCD_H*POK_COLORDEPTH/8 00285 #define LCDWIDTH POK_LCD_W 00286 #define LCDHEIGHT POK_LCD_H 00287 #define POK_BITFRAME 4840 00288 #elif POK_SCREENMODE == MODE_HI_16COLOR 00289 #define POK_SCREENBUFFERSIZE POK_LCD_W*POK_LCD_H/2 00290 #define LCDWIDTH 220 00291 #define LCDHEIGHT 176 00292 #define POK_BITFRAME 4840 00293 #elif POK_SCREENMODE == MODE_HI_4COLOR || POK_SCREENMODE == MODE_HI_GRAYSCALE 00294 #define POK_SCREENBUFFERSIZE POK_LCD_W*POK_LCD_H*POK_COLORDEPTH/4 00295 #define LCDWIDTH POK_LCD_W 00296 #define LCDHEIGHT POK_LCD_H 00297 #define POK_BITFRAME 4840 00298 #elif POK_SCREENMODE == MODE_FAST_16COLOR 00299 #define POK_SCREENBUFFERSIZE (POK_LCD_W/2)*(POK_LCD_H/2)*POK_COLORDEPTH/8 00300 #define XCENTER POK_LCD_W/4 00301 #define YCENTER POK_LCD_H/4 00302 #define LCDWIDTH 110 00303 #define LCDHEIGHT 88 00304 #define POK_BITFRAME 1210 00305 #elif POK_SCREENMODE == MODE_256_COLOR 00306 #define POK_SCREENBUFFERSIZE 72*58 00307 #define XCENTER 36 00308 #define YCENTER 29 00309 #define LCDWIDTH 72 00310 #define LCDHEIGHT 58 00311 #define POK_BITFRAME 72*58 00312 #elif POK_SCREENMODE == MODE_GAMEBUINO_16COLOR 00313 #define POK_SCREENBUFFERSIZE (84/2)*(48/2)*POK_COLORDEPTH/8 00314 #define LCDWIDTH 84 00315 #define LCDHEIGHT 48 00316 #define POK_BITFRAME 504 00317 #elif POK_SCREENMODE == MODE_ARDUBOY_16COLOR 00318 #define POK_SCREENBUFFERSIZE (128/2)*(64/2)*POK_COLORDEPTH/8 00319 #define LCDWIDTH 128 00320 #define LCDHEIGHT 64 00321 #define POK_BITFRAME 1024 00322 #elif POK_SCREENMODE == MODE_LAMENES 00323 #define POK_SCREENBUFFERSIZE (128)*(120)*POK_COLORDEPTH/8 00324 #define LCDWIDTH 128 00325 #define LCDHEIGHT 120 00326 #define POK_BITFRAME 1210 00327 #elif POK_SCREENMODE == MODE_GAMEBOY 00328 #define POK_SCREENBUFFERSIZE (160)*(144)/4 00329 #define LCDWIDTH 160 00330 #define LCDHEIGHT 144 00331 #define POK_BITFRAME 2880 00332 #else 00333 #define POK_SCREENBUFFERSIZE 0 00334 #endif // POK_SCREENMODE 00335 00336 #ifndef POK_STRETCH 00337 #define POK_STRETCH 1 // Stretch Gamebuino display 00338 #endif 00339 #ifndef POK_FPS 00340 #define POK_FPS 20 00341 #endif 00342 #define POK_FRAMEDURATION 1000/POK_FPS 00343 00344 /** SCROLL TEXT VS. WRAP AROUND WHEN PRINTING **/ 00345 #define SCROLL_TEXT 1 00346 00347 /** AUDIO **/ 00348 #define POK_AUD_PIN P2_19 00349 #define POK_AUD_PWM_US 31 00350 #define POK_AUD_FREQ 11025 //16000 //14285 //24000 // 14285 // 57143 // 8000 //11025// audio update frequency in Hz 00351 #define POK_CINC_MULTIPLIER 2 // multiplies synth cycle table 00352 #define POK_STREAMFREQ_HALVE 0 // if true, stream update freq is half audio freq 00353 #define POK_STREAM_LOOP 1 //master switch 00354 00355 #define POK_USE_DAC 1 // is DAC in use in this project 00356 #define POK_USE_EXT 0 // if extension port is in use or not 00357 #define POK_STREAM_TO_DAC 1 // 1 = stream from SD to DAC, synthesizer to PWM, 0 = opposite 00358 00359 00360 #define POK_BACKLIGHT_PIN P2_2 00361 #define POK_BACKLIGHT_INITIALVALUE 0.3f 00362 00363 #define POK_BATTERY_PIN1 P0_22 // read battery level through these pins 00364 #define POK_BATTERY_PIN2 P0_23 00365 00366 #define POK_BTN_A_PIN P1_9 00367 #define POK_BTN_B_PIN P1_4 00368 #define POK_BTN_C_PIN P1_10 00369 #define POK_BTN_UP_PIN P1_13 00370 #define POK_BTN_DOWN_PIN P1_3 00371 #define POK_BTN_LEFT_PIN P1_25 00372 #define POK_BTN_RIGHT_PIN P1_7 00373 00374 #define UPBIT 0 00375 #define DOWNBIT 1 00376 #define LEFTBIT 2 00377 #define RIGHTBIT 3 00378 #define ABIT 4 00379 #define BBIT 5 00380 #define CBIT 6 00381 00382 #endif // POKITTO_SETTINGS_H 00383
Generated on Tue Jul 12 2022 18:08:12 by
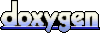