PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Fork of PokittoLib by
PokittoDisk.cpp
00001 /**************************************************************************/ 00002 /*! 00003 @file Pokitto_disk.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Pokitto development stage library 00009 Software License Agreement 00010 00011 Copyright (c) 2015, Jonne Valola ("Author") 00012 All rights reserved. 00013 00014 This library is intended solely for the purpose of Pokitto development. 00015 00016 Redistribution and use in source and binary forms, with or without 00017 modification requires written permission from Author. 00018 */ 00019 /**************************************************************************/ 00020 00021 #include "Pokitto.h " 00022 00023 #define SD_MOSI_PORT 0 00024 #define SD_MISO_PORT 0 00025 #define SD_SCK_PORT 0 00026 #define SD_CS_PORT 0 00027 #define SD_MOSI_PIN 9 00028 #define SD_MISO_PIN 8 00029 #define SD_SCK_PIN 6 00030 #define SD_CS_PIN 7 00031 00032 #if POK_ENABLE_SD > 0 00033 BYTE res; 00034 FATFS fs; /* File system object */ 00035 FATDIR dir; /* Directory object */ 00036 FILINFO fno; /* File information */ 00037 00038 //static FATFS *FatFs; /* Pointer to the file system object (logical drive) */ 00039 00040 bool diropened=false; 00041 00042 #define SPEAKER 3 00043 //#define BUFFER_SIZE 256 // was 128 00044 #define SONGLENGTH 0x1BFBCD // 1072223 00045 #define FILESIZE 0x1BFBCD 00046 00047 uint8_t filemode = FILE_MODE_UNINITIALIZED; 00048 char currentfile[15]; // holds current file's name 00049 00050 SPI device(CONNECT_MOSI,CONNECT_MISO,CONNECT_SCK); 00051 //DigitalOut mmccs(CONNECT_CS); 00052 00053 const char *get_filename_ext(const char *filename) { 00054 const char *dot = strrchr(filename, '.'); 00055 if(!dot || dot == filename) return ""; 00056 return dot + 1; 00057 } 00058 00059 __attribute__((section(".SD_Code"))) void initSDGPIO() { 00060 LPC_GPIO_PORT->DIR[SD_MOSI_PORT] |= (1 << SD_MOSI_PIN ); 00061 LPC_GPIO_PORT->DIR[SD_MISO_PORT] |= (1 << SD_MISO_PIN ); 00062 LPC_GPIO_PORT->DIR[SD_SCK_PORT] |= (1 << SD_SCK_PIN ); 00063 LPC_GPIO_PORT->DIR[SD_CS_PORT] |= (1 << SD_CS_PIN ); 00064 } 00065 00066 __attribute__((section(".SD_Code"))) int pokInitSD() { 00067 initSDGPIO(); 00068 res = disk_initialize(); 00069 res = (pf_mount(&fs)); 00070 res = pf_opendir(&dir,""); 00071 if (res) diropened=false; 00072 else diropened=true; 00073 return res; 00074 } 00075 00076 00077 /** PUBLIC FUNCTIONS **/ 00078 00079 char* getFirstDirEntry() { 00080 res=0; 00081 if (!diropened) { 00082 pokInitSD(); 00083 } 00084 res = pf_opendir(&dir,""); 00085 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00086 if (res) return 0; 00087 while (res==0) { //while res is ok 00088 if ((fno.fattrib & 0x02)==0) { 00089 if (fno.fattrib & 0x10) { 00090 fno.fname[8]='.'; 00091 fno.fname[9]='D'; 00092 fno.fname[10]='I'; 00093 fno.fname[11]='R'; 00094 fno.fname[12]='\0'; 00095 } 00096 return fno.fname; 00097 } 00098 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00099 if (res==0 && dir.index==0) break; 00100 } 00101 return 0; 00102 } 00103 00104 char* getNextDirEntry() { 00105 if (!diropened) pokInitSD(); 00106 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00107 if (res==0) { 00108 while (fno.fattrib & 0x02 && !res) res = pf_readdir(&dir,&fno); //system/hidden file 00109 if (fno.fattrib & 0x10) { 00110 int a=12; 00111 while (a) { 00112 fno.fname[a] = fno.fname[a-1]; 00113 a--; 00114 } 00115 if (fno.fname[0]) { 00116 fno.fname[0]='/'; 00117 a=0; 00118 while (fno.fname[a]) a++; 00119 fno.fname[a]='/'; 00120 } 00121 00122 /*fno.fname[a++]='.'; 00123 fno.fname[a++]='D'; 00124 fno.fname[a++]='I'; 00125 fno.fname[a++]='R'; 00126 fno.fname[a]='\0';*/ 00127 } 00128 return fno.fname; 00129 } 00130 return NULL; 00131 } 00132 00133 char* getNextFile (char* ext){ 00134 00135 if (!diropened) pokInitSD(); 00136 int a=1; 00137 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00138 while (res==0 || a) { //while there are entries and 00139 if (dir.index==0) return 0; //end of list 00140 a = strcmp((const char*)get_filename_ext(fno.fname),(const char*)ext); // returns 0 if strings are identical 00141 if (strcmp(ext,"")==0 && (fno.fattrib & 0x10) == 0) a=0; 00142 if (a == 0 && (fno.fattrib & 0x10) == 0) return fno.fname; 00143 if (fno.fattrib&0x10) return NULL; //its a directory 00144 } 00145 return 0; 00146 } 00147 00148 00149 char* getNextFile() { 00150 return getNextFile(""); 00151 } 00152 00153 char* getFirstFile(char* ext) { 00154 res=0; 00155 if (!diropened) { 00156 pokInitSD(); 00157 } 00158 res = pf_opendir(&dir,""); 00159 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00160 if (res) return 0; 00161 while (res==0 || (fno.fattrib & 0x10) == 0) { 00162 int a=0; 00163 a = strcmp((const char*)get_filename_ext(fno.fname),(const char*)ext); // returns 0 if strings are identical 00164 if (!strcmp(ext,"")) a=0; 00165 if ( a == 0 && (fno.fattrib & 0x10) == 0) return fno.fname; 00166 res = pf_readdir(&dir,&fno); //returns 0 if everything is OK 00167 if (res==0 && dir.index==0) break; 00168 } 00169 return 0; 00170 } 00171 00172 char* getFirstFile() { 00173 return getFirstFile(""); 00174 } 00175 00176 int isThisFileOpen(char* buffer){ 00177 int a=0; 00178 a = strcmp((const char*)buffer,(const char*)currentfile); // returns 0 if strings are identical 00179 if ( a == 0 && filemode != FILE_MODE_FAILED) return 1; 00180 return 0; 00181 } 00182 00183 int fileOK() { 00184 if (filemode != FILE_MODE_FAILED) return 1; 00185 return 0; 00186 } 00187 00188 uint8_t fileOpen(char* buffer, char fmode) { 00189 int err; 00190 if (filemode == FILE_MODE_UNINITIALIZED) { 00191 int a = pf_mount(&fs); 00192 if (a) return 1; // 1 means error in this context 00193 } 00194 00195 filemode = fmode; 00196 err = pf_open(buffer); 00197 if (err==0) { 00198 strcpy(currentfile,(const char*)buffer); 00199 return 0; // 0 means all clear 00200 } 00201 // file open failed 00202 filemode = FILE_MODE_FAILED; 00203 return 1; // 1 means failed 00204 } 00205 00206 void fileClose() { 00207 filemode = FILE_MODE_UNINITIALIZED; 00208 for (uint8_t i=0; i<15; i++) currentfile[i]=0; 00209 } 00210 00211 int fileGetChar() { 00212 BYTE buff[1]; 00213 WORD br; 00214 int err = pf_read(buff, 1, &br); /* Read data to the buff[] */ 00215 return buff[0]; 00216 } 00217 00218 void filePutChar(char c) { 00219 WORD bw; 00220 pf_write((const void*)&c, 1, &bw); 00221 pf_write(0, 0, &bw); 00222 } 00223 00224 void fileWriteBytes(uint8_t * b, uint16_t n) { 00225 WORD bw; 00226 pf_write((const void*)&b, n, &bw); 00227 pf_write(0, 0, &bw); 00228 } 00229 00230 uint16_t fileReadBytes(uint8_t * b, uint16_t n) { 00231 WORD br; 00232 pf_read(b, n, &br); /* Read data to the buff[] */ 00233 return br; /* Return number of bytes read */ 00234 } 00235 00236 void fileSeekAbsolute(long n) { 00237 res = pf_lseek(n); 00238 } 00239 00240 void fileSeekRelative(long n) { 00241 if (n<0) if (fs.fptr < -n) n=-fs.fptr; 00242 else if (n>0) if (fs.fptr+n > fs.fsize) n=fs.fsize-fs.fptr; 00243 res = pf_lseek(fs.fptr + n); 00244 } 00245 00246 void fileRewind() { 00247 res = pf_lseek(0); 00248 } 00249 00250 void fileEnd() { 00251 res = pf_lseek(fs.fsize); 00252 } 00253 00254 long int fileGetPosition() { 00255 return fs.fptr; 00256 } 00257 00258 uint8_t filePeek(long n) { 00259 pf_lseek(n); 00260 return fileGetChar(); 00261 } 00262 00263 void filePoke(long n, uint8_t c) { 00264 pf_lseek(n); 00265 filePutChar(c); 00266 } 00267 00268 int dirOpen() { 00269 return pf_opendir(&dir,""); 00270 } 00271 00272 int dirUp() { 00273 00274 return 0; 00275 } 00276 00277 #endif // POK_ENABLE_SD 00278 00279
Generated on Tue Jul 12 2022 18:08:12 by
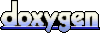