PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Fork of PokittoLib by
HWLCD.h
00001 /**************************************************************************/ 00002 /*! 00003 @file HWLCD.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #ifndef __HWLCD_H__ 00038 #define __HWLCD_H__ 00039 00040 #include "mbed.h" 00041 #include "gpio_api.h" 00042 #include "pinmap.h" 00043 00044 #define write_command write_command_16 00045 #define write_data write_data_16 00046 00047 namespace Pokitto { 00048 00049 00050 extern void initBacklight(); 00051 extern void setBacklight(float); 00052 extern void lcdFillSurface(uint16_t); 00053 extern void lcdPixel(int16_t x, int16_t y, uint16_t c); 00054 extern void setWindow(uint8_t x1, uint8_t y1, uint8_t x2, uint8_t y2); 00055 extern void lcdTile(int16_t x0, int16_t y0, int16_t width, int16_t height, uint16_t* gfx); 00056 extern void lcdRectangle(int16_t x, int16_t y,int16_t x2, int16_t y2, uint16_t color); 00057 extern void lcdInit(); 00058 extern void lcdSleep(); 00059 extern void lcdWakeUp(); 00060 extern void lcdRefresh(uint8_t *, uint16_t*); 00061 extern void lcdRefreshAB(uint8_t *, uint16_t*); 00062 extern void lcdRefreshGB(uint8_t *, uint16_t*); 00063 extern void lcdRefreshRegionMode1(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint8_t * scrbuf, uint16_t * paletteptr); 00064 extern void lcdRefreshMode1(uint8_t *, uint16_t*); 00065 extern void lcdRefreshMode2(uint8_t *, uint16_t*); 00066 extern void lcdRefreshMode3(uint8_t *, uint16_t*); 00067 extern void lcdRefreshModeGBC(uint8_t *, uint16_t*); 00068 /** Update LCD from 1-bit tile mode */ 00069 extern void lcdRefreshT1(uint8_t*, uint8_t*, uint8_t*, uint16_t*); 00070 extern void lcdClear(); 00071 extern void lcdFill(uint16_t); 00072 /** Blit one word of data*/ 00073 extern void blitWord(uint16_t); 00074 00075 /**************************************************************************/ 00076 /** PINS AND PORTS **/ 00077 /**************************************************************************/ 00078 00079 #if POK_BOARDREV == 1 00080 /** 2-layer board version 1.3 **/ 00081 #define LCD_CD_PORT 0 00082 #define LCD_CD_PIN 2 00083 #define LCD_WR_PORT 1 00084 #define LCD_WR_PIN 23 00085 #define LCD_RD_PORT 1 00086 #define LCD_RD_PIN 24 00087 #define LCD_RES_PORT 1 00088 #define LCD_RES_PIN 28 00089 #else 00090 /** 4-layer board version 2.1 **/ 00091 #define LCD_CD_PORT 0 00092 #define LCD_CD_PIN 2 00093 #define LCD_WR_PORT 1 00094 #define LCD_WR_PIN 12 00095 #define LCD_RD_PORT 1 00096 #define LCD_RD_PIN 24 00097 #define LCD_RES_PORT 1 00098 #define LCD_RES_PIN 0 00099 #endif 00100 00101 /**************************************************************************/ 00102 /** LCD CONTROL MACROS **/ 00103 /**************************************************************************/ 00104 00105 #define CLR_RESET LPC_GPIO_PORT->CLR[LCD_RES_PORT] = 1 << LCD_RES_PIN; //RST = (0); // Clear pin 00106 #define SET_RESET LPC_GPIO_PORT->SET[LCD_RES_PORT] = 1 << LCD_RES_PIN; // RST = (1); // Set pin 00107 00108 #define CLR_CD { LPC_GPIO_PORT->CLR[LCD_CD_PORT] = 1 << LCD_CD_PIN; } // RS = (0); // Clear pin 00109 #define SET_CD { LPC_GPIO_PORT->SET[LCD_CD_PORT] = 1 << LCD_CD_PIN; }// RS = (1); // Set pin 00110 00111 #define CLR_WR { LPC_GPIO_PORT->CLR[LCD_WR_PORT] = 1 << LCD_WR_PIN; __asm("nop");__asm("nop");}//WR = (0); // Clear pin 00112 #define SET_WR LPC_GPIO_PORT->SET[LCD_WR_PORT] = 1 << LCD_WR_PIN; //WR = (1); // Set pin 00113 00114 #define CLR_RD LPC_GPIO_PORT->CLR[LCD_RD_PORT] = 1 << LCD_RD_PIN; //RD = (0); // Clear pin 00115 #define SET_RD LPC_GPIO_PORT->SET[LCD_RD_PORT] = 1 << LCD_RD_PIN; //RD = (1); // Set pin 00116 00117 #define SET_CS //CS tied to ground 00118 #define CLR_CS 00119 00120 #define CLR_CS_CD_SET_RD_WR {CLR_CD; SET_RD; SET_WR;} 00121 #define CLR_CS_SET_CD_RD_WR {SET_CD; SET_RD; SET_WR;} 00122 #define SET_CD_RD_WR {SET_CD; SET_RD; SET_WR;} 00123 #define SET_WR_CS SET_WR; 00124 00125 #define SET_MASK_P2 LPC_GPIO_PORT->MASK[2] = ~(0x7FFF8); //mask P2_3 ...P2_18 00126 #define CLR_MASK_P2 LPC_GPIO_PORT->MASK[2] = 0; // all on 00127 00128 00129 00130 /**************************************************************************/ 00131 /** SETUP GPIO & DATA **/ 00132 /**************************************************************************/ 00133 00134 static void setup_gpio() 00135 { 00136 /** control lines **/ 00137 LPC_GPIO_PORT->DIR[LCD_CD_PORT] |= (1 << LCD_CD_PIN ); 00138 LPC_GPIO_PORT->DIR[LCD_WR_PORT] |= (1 << LCD_WR_PIN ); 00139 LPC_GPIO_PORT->DIR[LCD_RD_PORT] |= (1 << LCD_RD_PIN ); 00140 LPC_GPIO_PORT->DIR[LCD_RES_PORT] |= (1 << LCD_RES_PIN ); 00141 /** data lines **/ 00142 LPC_GPIO_PORT->DIR[2] |= (0xFFFF << 3); // P2_3...P2_18 as output 00143 00144 pin_mode(P2_3,PullNone); // turn off pull-up 00145 pin_mode(P2_4,PullNone); // turn off pull-up 00146 pin_mode(P2_5,PullNone); // turn off pull-up 00147 pin_mode(P2_6,PullNone); // turn off pull-up 00148 00149 pin_mode(P2_7,PullNone); // turn off pull-up 00150 pin_mode(P2_8,PullNone); // turn off pull-up 00151 pin_mode(P2_9,PullNone); // turn off pull-up 00152 pin_mode(P2_10,PullNone); // turn off pull-up 00153 00154 pin_mode(P2_11,PullNone); // turn off pull-up 00155 pin_mode(P2_12,PullNone); // turn off pull-up 00156 pin_mode(P2_13,PullNone); // turn off pull-up 00157 pin_mode(P2_14,PullNone); // turn off pull-up 00158 00159 pin_mode(P2_15,PullNone); // turn off pull-up 00160 pin_mode(P2_16,PullNone); // turn off pull-up 00161 pin_mode(P2_17,PullNone); // turn off pull-up 00162 pin_mode(P2_18,PullNone); // turn off pull-up 00163 } 00164 00165 00166 00167 00168 #define HI_BYTE(d) (LPC_GPIO->MPIN[1]= (d<<13)) //((d>>8)<<21)) 00169 #define LO_BYTE(d) (LPC_GPIO->MPIN[1]= (d<<21)) //because of mask makes no difference 00170 00171 // Macros to set data bus direction to input/output 00172 #define LCD_GPIO2DATA_SETINPUT GPIO_GPIO2DIR &= ~LCD_DATA_MASK 00173 #define LCD_GPIO2DATA_SETOUTPUT GPIO_GPIO2DIR |= LCD_DATA_MASK 00174 00175 00176 // Basic Color definitions 00177 #define COLOR_BLACK (uint16_t)(0x0000) 00178 #define COLOR_BLUE (uint16_t)(0x001F) 00179 #define COLOR_RED (uint16_t)(0xF800) 00180 #define COLOR_GREEN (uint16_t)(0x07E0) 00181 #define COLOR_CYAN (uint16_t)(0x07FF) 00182 #define COLOR_MAGENTA (uint16_t)(0xF81F) 00183 #define COLOR_YELLOW (uint16_t)(0xFFE0) 00184 #define COLOR_WHITE (uint16_t)(0xFFFF) 00185 00186 // Grayscale Values 00187 #define COLOR_GRAY_15 (uint16_t)(0x0861) // 15 15 15 00188 #define COLOR_GRAY_30 (uint16_t)(0x18E3) // 30 30 30 00189 #define COLOR_GRAY_50 (uint16_t)(0x3186) // 50 50 50 00190 #define COLOR_GRAY_80 (uint16_t)(0x528A) // 80 80 80 00191 #define COLOR_GRAY_128 (uint16_t)(0x8410) // 128 128 128 00192 #define COLOR_GRAY_200 (uint16_t)(0xCE59) // 200 200 200 00193 #define COLOR_GRAY_225 (uint16_t)(0xE71C) // 225 225 225 00194 00195 00196 } // namespace pokitto 00197 #endif // __HWLCD_H_ 00198
Generated on Tue Jul 12 2022 18:08:12 by
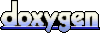