
Now supports DS18B20 and DS18S20 Maxim/Dallas one-wire thermometer devices. Also supports DS18S20 in 9, 10, 11, and 12 bit resolution modes. 'Use Address' mode now checks if the correct device type is present, and informs the user which device to use. Correct temperature conversion times now used in non-parasitic mode. The device should be placed at least 6 inches (15 cm) from the mbed board in order to accurately read ambient temperature.
DS18B20.cpp
00001 /* 00002 * DS18B20. Maxim DS18B20 One-Wire Thermometer. 00003 * Uses the OneWireCRC library. 00004 * 00005 * Copyright (C) <2010> Petras Saduikis <petras@petras.co.uk> 00006 * 00007 * This file is part of OneWireThermometer. 00008 * 00009 * OneWireThermometer is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * OneWireThermometer is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with OneWireThermometer. If not, see <http://www.gnu.org/licenses/>. 00021 */ 00022 00023 #include "DS18B20.h" 00024 #include "DebugTrace.h" 00025 00026 DebugTrace pc_ds18B20(ON, TO_SERIAL); 00027 00028 DS18B20::DS18B20(bool crcOn, bool useAddr, bool parasitic, PinName pin) : 00029 OneWireThermometer(crcOn, useAddr, parasitic, pin, DS18B20_ID) 00030 { 00031 } 00032 00033 void DS18B20::setResolution(eResolution resln) 00034 { 00035 // as the write to the configuration register involves a write to the 00036 // high and low alarm bytes, need to read these registers first 00037 // and copy them back on the write 00038 00039 BYTE read_data[THERMOM_SCRATCHPAD_SIZE]; 00040 BYTE write_data[ALARM_CONFIG_SIZE]; 00041 00042 if (readAndValidateData(read_data)) 00043 { 00044 // copy alarm and config data to write data 00045 for (int k = 2; k < 5; k++) 00046 { 00047 write_data[k - 2] = read_data[k]; 00048 } 00049 int config = write_data[2]; 00050 config &= 0x9F; 00051 config ^= (resln << 5); 00052 write_data[2] = config; 00053 00054 resetAndAddress(); 00055 oneWire.writeByte(WRITESCRATCH); 00056 for (int k = 0; k < 3; k++) 00057 { 00058 oneWire.writeByte(write_data[k]); 00059 } 00060 00061 // remember it so we can use the correct delay in reading the temperature 00062 // for parasitic power 00063 resolution = resln; 00064 } 00065 } 00066 00067 float DS18B20::calculateTemperature(BYTE* data) 00068 { 00069 bool signBit = false; 00070 if (data[TEMPERATURE_MSB] & 0x80) signBit = true; 00071 00072 int read_temp = (data[TEMPERATURE_MSB] << 8) + data[TEMPERATURE_LSB]; 00073 if (signBit) 00074 { 00075 read_temp = (read_temp ^ 0xFFFF) + 1; // two's complement 00076 read_temp *= -1; 00077 } 00078 00079 int resolution = (data[CONFIG_REG_BYTE] & 0x60) >> 5; // mask off bits 6,5 and move to 1,0 00080 switch (resolution) 00081 { 00082 case nineBit: // 0.5 deg C increments 00083 read_temp &= 0xFFF8; // bits 2,1,0 are undefined 00084 pc_ds18B20.traceOut("9 bit resolution ...\r\n"); 00085 break; 00086 case tenBit: // 0.25 deg C increments 00087 read_temp &= 0xFFFC; // bits 1,0 are undefined 00088 pc_ds18B20.traceOut("10 bit resolution ...\r\n"); 00089 break; 00090 case elevenBit: // 0.125 deg C increments 00091 read_temp &= 0xFFFE; // bit 0 is undefined 00092 pc_ds18B20.traceOut("11 bit resolution ...\r\n"); 00093 break; 00094 case twelveBit: // 0.0625 deg C increments 00095 pc_ds18B20.traceOut("12 bit resolution ...\r\n"); 00096 break; 00097 } 00098 float realTemp = (float)read_temp/16 ; 00099 00100 pc_ds18B20.traceOut("TEMP_READ/REAL TEMP: %f \r\n", realTemp); 00101 00102 return realTemp; 00103 }
Generated on Sat Jul 16 2022 06:12:12 by
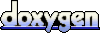