
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
UARTTunnel.cpp
00001 /* 00002 * UARTTunnel.cpp 00003 * 00004 * Created on: 10.06.2016 00005 * Author: Adrian 00006 */ 00007 00008 #include "UARTTunnel.h" 00009 00010 UART_Tunnel::UART_Tunnel() { 00011 this->uartSerial = new mbed::RawSerial(XBEE_DOUT,XBEE_DIN); 00012 this->usbSerial = new mbed::RawSerial(USBTX,USBRX); 00013 00014 uartSerial->baud(9600); 00015 usbSerial->baud(9600); 00016 uartSerial->attach(this,&UART_Tunnel::onUartRx,this->uartSerial->RxIrq); 00017 usbSerial->attach(this,&UART_Tunnel::onUsbRx,this->usbSerial->RxIrq); 00018 00019 } 00020 UART_Tunnel::UART_Tunnel(mbed::RawSerial* uartSerial,mbed::RawSerial* usbSerial){ 00021 this->uartSerial = uartSerial; 00022 this->usbSerial = usbSerial; 00023 00024 uartSerial->attach(this,&UART_Tunnel::onUartRx,this->uartSerial->RxIrq); 00025 usbSerial->attach(this,&UART_Tunnel::onUsbRx,this->usbSerial->RxIrq); 00026 } 00027 00028 UART_Tunnel::~UART_Tunnel() { 00029 // TODO Auto-generated destructor stub 00030 } 00031 00032 void UART_Tunnel::onUartRx(){ 00033 if(uartSerial->readable()){ 00034 usbSerial->putc(uartSerial->getc()); 00035 } 00036 } 00037 00038 void UART_Tunnel::onUsbRx(){ 00039 if(usbSerial->readable()){ 00040 uartSerial->putc(usbSerial->getc()); 00041 } 00042 00043 } 00044
Generated on Wed Jul 13 2022 09:23:48 by
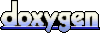