
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskTemperature.h
00001 /** 00002 * @file TaskTemperature.h 00003 * 00004 * @author Adrian 00005 * @date 30.05.2016 00006 * 00007 */ 00008 00009 #include "BME280.h" 00010 #include "BME280TemperatureMessage.h " 00011 #include "main.h" 00012 00013 #ifndef TASKTEMPERATURE_H_ 00014 #define TASKTEMPERATURE_H_ 00015 00016 /** 00017 * @class TaskTemperature 00018 * @brief This TaskTemperature Class handles the temperature measurement using the BME280. 00019 * Starting the task using the start() starts the measurement of all axis. 00020 * It can be used alongside with other measurement Tasks inside the mbed::rtos 00021 * environment. The Task Class basically wraps mbeds Thread functionality. 00022 */ 00023 class TaskTemperature { 00024 public: 00025 TaskTemperature(BME280*,Mutex*, Queue<BME280TemperatureMessage,TEMPERATURE_QUEUE_LENGHT>*); 00026 TaskTemperature(BME280*,Mutex*,Queue<BME280TemperatureMessage,TEMPERATURE_QUEUE_LENGHT>*, 00027 osPriority, uint32_t, unsigned char*); 00028 virtual ~TaskTemperature(); 00029 00030 00031 /** 00032 * @brief Starts the task by building it and connecting a callback function to 00033 * the mbed::Thread 00034 * @return 00035 */ 00036 osStatus start(); 00037 00038 /** 00039 * @brief Stops the task. Should only be used after start() was used 00040 * @return 00041 */ 00042 osStatus stop(); 00043 00044 00045 /** 00046 * @brief Gets the actual state of the Task either RUNNING or SLEEPING 00047 * @return 00048 */ 00049 TASK_STATE getState(); 00050 00051 private: 00052 rtos::Thread* thread; 00053 rtos::Queue<BME280TemperatureMessage,TEMPERATURE_QUEUE_LENGHT>* queue; 00054 rtos::Mutex* mutexI2C ; 00055 osPriority priority; 00056 uint32_t stack_size; 00057 unsigned char *stack_pointer; 00058 00059 TASK_STATE state; 00060 00061 BME280* bme280; 00062 00063 00064 /** 00065 * @brief A Callback function thats called by the mbed::Thread of this TaskClass 00066 * @param 00067 */ 00068 static void callBack(void const *); 00069 00070 /** 00071 * @brief A thread safe method that measures the temperature. After measuring 00072 * the temperature it stores the data inside a BME280TemperatureMessage 00073 */ 00074 void measureTemperature(); 00075 00076 00077 /** 00078 * @brief Sets the message Queue of the Task where the measured values will be stored 00079 * after the measurement 00080 * @param queueGyro the queue where the MPU9250GyroscopeMessage will be stored 00081 */ 00082 void setQueue(Queue<BME280TemperatureMessage,TEMPERATURE_QUEUE_LENGHT>* queueTemperature); 00083 00084 /** 00085 * @brief Sets the mutex thats used for a thread safe measurement 00086 * @param mutexI2C the I2C mutex 00087 */ 00088 void setMutex(Mutex* mutexI2C); 00089 00090 /** 00091 * @brief Sets the priority of the Task 00092 * @param priority priority of the Task 00093 */ 00094 void setPriority(osPriority priority); 00095 00096 /** 00097 * @brief Sets the size of the Task 00098 * @param stackSize the stack size in Bytes 00099 */ 00100 void setStackSize(uint32_t stackSize); 00101 00102 /** 00103 * @brief Sets the stack pointer of for the task stack 00104 * @param stackPointer 00105 */ 00106 void setStackPointer(unsigned char* stackPointer); 00107 00108 00109 /** 00110 * @brief Sets the actual state of the Task. 00111 * @param taskState either RUNNING or SLEEPING 00112 */ 00113 void setState(TASK_STATE taskState); 00114 }; 00115 00116 #endif /* TASKTEMPERATURE_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
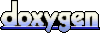