
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskLoRaMeasurement.h
00001 /** 00002 * @file TaskLoRaMeasurement.h 00003 * 00004 * @author Adrian 00005 * @date 13.06.2016 00006 */ 00007 00008 #include <Thread.h> 00009 #include <Queue.h> 00010 #include <Mutex.h> 00011 #include "LoRa.h " 00012 #include "LoRaMeasuermentMessage.h " 00013 #include "main.h" 00014 00015 #ifndef APP_TASKLORAMEASUREMENT_H_ 00016 #define APP_TASKLORAMEASUREMENT_H_ 00017 00018 class TaskLoRaMeasurement { 00019 public: 00020 TaskLoRaMeasurement(LoRa*,Mutex*, Queue<LoRaMeasurementMessage,LORA_MEASUREMENT_QUEUE_LENGHT>*); 00021 TaskLoRaMeasurement(LoRa*,Mutex*,Queue<LoRaMeasurementMessage,LORA_MEASUREMENT_QUEUE_LENGHT>*, 00022 osPriority, uint32_t, unsigned char*); 00023 virtual ~TaskLoRaMeasurement(); 00024 00025 osStatus start(); 00026 osStatus stop(); 00027 00028 TASK_STATE getState(); 00029 00030 private: 00031 rtos::Thread* thread; 00032 rtos::Queue<LoRaMeasurementMessage,LORA_MEASUREMENT_QUEUE_LENGHT>* queue; 00033 rtos::Mutex* mutexLoRa ; 00034 osPriority priority; 00035 uint32_t stack_size; 00036 unsigned char *stack_pointer; 00037 00038 TASK_STATE state; 00039 00040 LoRa* lora; 00041 00042 static void callBack(void const *); 00043 void measureSignal(); 00044 00045 void setQueue(Queue<LoRaMeasurementMessage,LORA_MEASUREMENT_QUEUE_LENGHT>*); 00046 void setMutex(Mutex*); 00047 void setPriority(osPriority); 00048 void setStackSize(uint32_t); 00049 void setStackPointer(unsigned char*); 00050 00051 void setState(TASK_STATE); 00052 00053 }; 00054 00055 #endif /* APP_TASKLORAMEASUREMENT_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
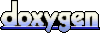