
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskHumidity.h
00001 /** 00002 * @file TaksHumidity.h 00003 * 00004 * @author Adrian 00005 * @date 30.05.2016 00006 * 00007 00008 */ 00009 #include "BME280.h" 00010 #include "BME280HumidityMessage.h " 00011 #include "main.h" 00012 00013 #ifndef TASKHUMIDITY_H_ 00014 #define TASKHUMIDITY_H_ 00015 00016 /** 00017 * @class TaskHumidity 00018 * @brief This TaskHumidity Class handles the humidity measurement using the BME280. 00019 * Starting the task using the start() starts the measurement. 00020 * It can be used alongside with other measurement Tasks inside the mbed::rtos 00021 * environment. The Task Class basically wraps mbeds Thread functionality. 00022 */ 00023 class TaskHumidity { 00024 00025 public: 00026 TaskHumidity(BME280*,Mutex*, Queue<BME280HumidityMessage,HUMIDITY_QUEUE_LENGHT>*); 00027 TaskHumidity(BME280*,Mutex*,Queue<BME280HumidityMessage,HUMIDITY_QUEUE_LENGHT>*, 00028 osPriority, uint32_t, unsigned char*); 00029 virtual ~TaskHumidity(); 00030 00031 00032 /** 00033 * Starts the task by building and its measurement 00034 * @return 00035 */ 00036 osStatus start(); 00037 00038 /** 00039 * Stops the task. Should only be used after start() was used 00040 * @return 00041 */ 00042 osStatus stop(); 00043 00044 00045 /** 00046 * Gets the actual state of the Task either RUNNING or SLEEPING 00047 * @return 00048 */ 00049 TASK_STATE getState(); 00050 00051 private: 00052 rtos::Thread* thread; 00053 rtos::Queue<BME280HumidityMessage,HUMIDITY_QUEUE_LENGHT>* queue; 00054 rtos::Mutex* mutexI2C ; 00055 osPriority priority; 00056 uint32_t stack_size; 00057 unsigned char *stack_pointer; 00058 00059 TASK_STATE state; 00060 00061 BME280* bme280; 00062 BME280_MODE bme280Mode; 00063 00064 00065 /** 00066 * @brief A Callback function thats called by the mbed::Thread of this TaskClass 00067 * @param 00068 */ 00069 static void callBack(void const *); 00070 00071 /** 00072 * @brief A thread safe method that measures the humidity. After measuring the humidity 00073 * it stores the humidity value inside a BME280HumidityMessage 00074 */ 00075 void measureHumidity(); 00076 00077 00078 /** 00079 * @brief Sets the message Queue of the Task where the measured values will be stored 00080 * after the measurement 00081 * @param queueHumidity the queue where the BME280Humidity will be stored 00082 */ 00083 void setQueue(Queue<BME280HumidityMessage,HUMIDITY_QUEUE_LENGHT>* queueHumdity); 00084 00085 /** 00086 * @brief Sets the mutex thats used for a thread safe measurement 00087 * @param mutexI2C the I2C mutex 00088 */ 00089 void setMutex(Mutex* mutexI2C); 00090 00091 /** 00092 * @brief Sets the priority of the Task 00093 * @param priority priority of the Task 00094 */ 00095 void setPriority(osPriority priority); 00096 00097 /** 00098 * @brief Sets the size of the Task 00099 * @param stackSize the stack size in Bytes 00100 */ 00101 void setStackSize(uint32_t stackSize); 00102 00103 /** 00104 * @brief Sets the stack pointer of for the task stack 00105 * @param stackPointer 00106 */ 00107 void setStackPointer(unsigned char* stackPointer); 00108 00109 00110 /** 00111 * @brief Sets the actual state of the Task. 00112 * @param taskState either RUNNING or SLEEPING 00113 */ 00114 void setState(TASK_STATE); 00115 }; 00116 00117 00118 #endif /* TASKHUMIDITY_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
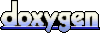