Library for taking datasheet corrected relative humidity measurements with HIH-4030 humidity sensor
Embed:
(wiki syntax)
Show/hide line numbers
hih-4030.cpp
00001 #include "hih-4030.h" 00002 00003 HIH4030::HIH4030(PinName vout):vout_(vout) { 00004 00005 } 00006 00007 /* 00008 gives humidity as a ratio of VDD 00009 */ 00010 float HIH4030::ratioHumidity(){ 00011 //poll analogue in 00012 return vout_.read(); 00013 } 00014 00015 /* 00016 gives humidity as a percentage - numbers taken from datasheet 00017 */ 00018 00019 float HIH4030::sensorRH(){ 00020 //poll analogue in 00021 sample = vout_.read()*5; //multiply by 5 as sample is a decimal of Vdd 00022 return (sample-0.958)/0.0307; 00023 } 00024 00025 /* 00026 gives humidity adjusted for temperature (in degrees C) - numbers taken from datasheet 00027 */ 00028 00029 float HIH4030::trueSensorRH(float temperature){ 00030 float rh = sensorRH(); 00031 temperature = temperature*0.00216; 00032 return rh/(1.0546 - temperature); 00033 }
Generated on Wed Jul 13 2022 01:49:11 by
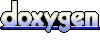