
ADC Spike2
Embed:
(wiki syntax)
Show/hide line numbers
adc.h
00001 /* mbed Library - ADC 00002 * Copyright (c) 2010, sblandford 00003 * released under MIT license http://mbed.org/licence/mit 00004 */ 00005 00006 #ifndef MBED_ADC_H 00007 #define MBED_ADC_H 00008 00009 #include "mbed.h" 00010 #define XTAL_FREQ 12000000 00011 #define MAX_ADC_CLOCK 13000000 00012 #define CLKS_PER_SAMPLE 64 00013 00014 class ADC { 00015 public: 00016 00017 //Initialize ADC with ADC maximum sample rate of 00018 //sample_rate and system clock divider of cclk_div 00019 //Maximum recommened sample rate is 184000 00020 ADC(int sample_rate, int cclk_div); 00021 00022 //Enable/disable ADC on pin according to state 00023 //and also select/de-select for next conversion 00024 void setup(PinName pin, int state); 00025 00026 //Return enabled/disabled state of ADC on pin 00027 int setup(PinName pin); 00028 00029 //Enable/disable burst mode according to state 00030 void burst(int state); 00031 00032 //Select channel already setup 00033 void select(PinName pin); 00034 00035 //Return burst mode enabled/disabled 00036 int burst(void); 00037 00038 /*Set start condition and edge according to mode: 00039 0 - No start (this value should be used when clearing PDN to 0). 00040 1 - Start conversion now. 00041 2 - Start conversion when the edge selected by bit 27 occurs on the P2.10 / EINT0 / NMI pin. 00042 3 - Start conversion when the edge selected by bit 27 occurs on the P1.27 / CLKOUT / 00043 USB_OVRCRn / CAP0.1 pin. 00044 4 - Start conversion when the edge selected by bit 27 occurs on MAT0.1. Note that this does 00045 not require that the MAT0.1 function appear on a device pin. 00046 5 - Start conversion when the edge selected by bit 27 occurs on MAT0.3. Note that it is not 00047 possible to cause the MAT0.3 function to appear on a device pin. 00048 6 - Start conversion when the edge selected by bit 27 occurs on MAT1.0. Note that this does 00049 not require that the MAT1.0 function appear on a device pin. 00050 7 - Start conversion when the edge selected by bit 27 occurs on MAT1.1. Note that this does 00051 not require that the MAT1.1 function appear on a device pin. 00052 When mode >= 2, conversion is triggered by edge: 00053 0 - Rising edge 00054 1 - Falling edge 00055 */ 00056 void startmode(int mode, int edge); 00057 00058 //Return startmode state according to mode_edge=0: mode and mode_edge=1: edge 00059 int startmode(int mode_edge); 00060 00061 //Start ADC conversion 00062 void start(void); 00063 00064 //Set interrupt enable/disable for pin to state 00065 void interrupt_state(PinName pin, int state); 00066 00067 //Return enable/disable state of interrupt for pin 00068 int interrupt_state(PinName pin); 00069 00070 //Attach custom interrupt handler replacing default 00071 void attach(void(*fptr)(void)); 00072 00073 //Restore default interrupt handler 00074 void detach(void); 00075 00076 //Append custom interrupt handler for pin 00077 void append(PinName pin, void(*fptr)(uint32_t value)); 00078 00079 //Unappend custom interrupt handler for pin 00080 void unappend(PinName pin); 00081 00082 //Append custom global interrupt handler 00083 void append(void(*fptr)(int chan, uint32_t value)); 00084 00085 //Unappend custom global interrupt handler 00086 void unappend(void); 00087 00088 //Set ADC offset to a value 0-7 00089 void offset(int offset); 00090 00091 //Return current ADC offset 00092 int offset(void); 00093 00094 //Return value of ADC on pin 00095 int read(PinName pin); 00096 00097 //Return DONE flag of ADC on pin 00098 int done(PinName pin); 00099 00100 //Return OVERRUN flag of ADC on pin 00101 int overrun(PinName pin); 00102 00103 //Return actual ADC clock 00104 int actual_adc_clock(void); 00105 00106 //Return actual maximum sample rate 00107 int actual_sample_rate(void); 00108 00109 //Return pin ID of ADC channel 00110 PinName channel_to_pin(int chan); 00111 00112 //Return pin number of ADC channel 00113 int channel_to_pin_number(int chan); 00114 00115 00116 private: 00117 int _pin_to_channel(PinName pin); 00118 uint32_t _data_of_pin(PinName pin); 00119 00120 int _adc_clk_freq; 00121 void adcisr(void); 00122 static void _adcisr(void); 00123 static ADC *instance; 00124 00125 uint32_t _adc_data[8]; 00126 void(*_adc_isr[8])(uint32_t value); 00127 void(*_adc_g_isr)(int chan, uint32_t value); 00128 void(*_adc_m_isr)(void); 00129 }; 00130 00131 #endif
Generated on Mon Jul 18 2022 10:50:02 by
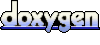