
Racing Robots Session
Fork of racing_robots by
Embed:
(wiki syntax)
Show/hide line numbers
robot_logic.h
00001 #ifndef H_ROBOT_LOGIC 00002 #define H_ROBOT_LOGIC 00003 00004 #include "mbed.h" 00005 #include "m3pi.h" 00006 00007 typedef enum { 00008 LED_1 = 0, 00009 LED_2 = 1, 00010 LED_3 = 2, 00011 LED_4 = 3, 00012 LED_5 = 4, 00013 LED_6 = 5, 00014 LED_7 = 6, 00015 LED_8 = 7 00016 } LedIndex; 00017 00018 typedef enum { 00019 LED_ON = 0, 00020 LED_OFF = 1, 00021 LED_TOGGLE = 2 00022 } LedState; 00023 00024 00025 /** 00026 * Drive the robot forward or backward. 00027 * If the robot was turning it will stop turning and drive in a straight line. 00028 * 00029 * @param speed The speed percentage with which to drive forward or backward. 00030 * Can range from -100 (full throttle backward) to +100 (full throttle forward). 00031 */ 00032 void drive(int speed); 00033 00034 /** 00035 * Turn the robot left or right while driving. 00036 * 00037 * @param turnspeed The percentage with which to turn the robot. 00038 * Can range from -100 (full throttle left) to +100 (full throttle right). 00039 */ 00040 void turn(int turnspeed); 00041 00042 /** 00043 * Stop the robot. 00044 */ 00045 void stop(void); 00046 00047 /** 00048 * Calibrate the line follow sensors. 00049 * Take note that the robot should be placed over the line 00050 * before this function is called and that it will rotate to 00051 * both sides. 00052 */ 00053 void sensor_calibrate(void); 00054 00055 /** 00056 * Read the value from the line sensor. The returned value indicates the 00057 * position of the line. The value ranges from -100 to +100 where -100 is 00058 * fully left, +100 is fully right and 0 means the line is detected in the middle. 00059 * 00060 * @return The position of the line with a range of -100 to +100. 00061 */ 00062 int line_sensor(void); 00063 00064 /** 00065 * Initialize the PID drive control with 00066 * the P, I and D factors. 00067 * 00068 * @param p The P factor 00069 * @param i The I factor 00070 * @param d The D factor 00071 */ 00072 void pid_init(int p, int i, int d); 00073 00074 /** 00075 * Determine PID turnspeed with which the robot should 00076 * turn to follow the line at the given position. 00077 * 00078 * @param line_position The position of the line in a range of [-100, +100] 00079 * 00080 * @return The turnspeed in a range of [-100, +100] 00081 */ 00082 int pid_turn(int line_position); 00083 00084 /** 00085 *Show speed, turn and sensor data on the LCD 00086 */ 00087 void show_stats(); 00088 00089 /** 00090 * Shows the name of the robot on the display. 00091 * 00092 * @param name C character string (null-terminated) with the name of the robot (max 8 chars) 00093 */ 00094 void show_name(char * name); 00095 00096 00097 /** 00098 * Turn on, off or toggle a specific LED 00099 * @param i the LED number LED_0 .. LED_7 00100 * @param state the LED state LED_ON, LED_OFF, LED_TOGGLE 00101 * @example led(LED_0, LED_ON); turns LED 0 on. 00102 */ 00103 void led(LedIndex i, LedState state); 00104 00105 /** 00106 * Wait for an approximate number of milliseconds. 00107 * 00108 * @param milliseconds The number of milliseconds to wait. 00109 */ 00110 void await(int milliseconds); 00111 00112 #endif
Generated on Sun Jul 24 2022 11:47:09 by
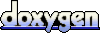