library for m3Dpi robot, based on the Pololu 3pi and m3pi. m3Dpi has multiple distance sensors, gyroscope, compass and accelerometer sensor to be fully aware of its environment. With the addition of xbee or nrf24n01 module it has wireless communication capabilities.
Dependencies: m3pi ADXL345_I2C HMC5583L ITG3200 PCA9547 TLC59116 VL6180x RGB-fun xbee
M3Dpi.h
00001 #pragma once 00002 00003 #include "m3pi.h" 00004 00005 #include "StatusLed.h" 00006 #include "Color.h" 00007 #include "LedRing.h" 00008 #include "hmc5583l.h" 00009 #include "ITG3200.h" 00010 #include "ADXL345_I2C.h" 00011 #include "PCA9547.h" 00012 //#include "vl6108x.h" 00013 #include "vl6180xManager.h" 00014 #include "xbee.h" 00015 #include "datatypes.h" 00016 00017 class M3Dpi : public m3pi 00018 { 00019 00020 public: 00021 M3Dpi(); 00022 00023 void setStatus(int color); 00024 void setStatus(Color* color); 00025 00026 void setLeds(int* colors); 00027 m3dpi::Distance getDistance(); 00028 m3dpi::Direction getDirection(); // compass 00029 m3dpi::Rotation getRotation(); // gyro 00030 m3dpi::Acceleration getAcceleration(); 00031 time_t getTime(); 00032 00033 // buttonhandler (interrupt based?) 00034 00035 //protected: // public for hardware testing only 00036 00037 I2C i2c; 00038 00039 StatusLed status; 00040 LedRing leds; 00041 00042 HMC5583L compass; 00043 ITG3200 gyro; 00044 ADXL345_I2C accelerometer; 00045 VL6180xManager distance; 00046 Xbee xbee; 00047 00048 static const PinName SDA = p28; 00049 static const PinName SCL = p27; 00050 static const PinName XBEE_TX = p13; 00051 static const PinName XBEE_RX = p14; 00052 static const PinName XBEE_RESET = p12; 00053 static const PinName STATUS_RED = p18; 00054 static const PinName STATUS_GREEN = p19; 00055 static const PinName STATUS_BLUE = p20; 00056 00057 static const int XBEE_BAUD = 57600; 00058 00059 virtual int _putc(int c); 00060 virtual int _getc(); 00061 00062 };
Generated on Thu Jul 14 2022 05:29:58 by
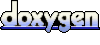