
Racing Robots remote control using xbee and API mode
Dependencies: XBeeLib mbed racing_robots
main.cpp
00001 #include "robot_logic.h" 00002 00003 #include <cstdint> 00004 #include "mbed.h" 00005 #include "XBeeLib.h" 00006 #if defined(ENABLE_LOGGING) 00007 #include "DigiLoggerMbedSerial.h" 00008 using namespace DigiLog; 00009 #endif 00010 00011 using namespace XBeeLib; 00012 00013 DigitalOut led1(LED1); 00014 00015 Serial *log_serial; 00016 00017 Timeout stopper; 00018 00019 /** Callback function, invoked at packet reception */ 00020 static void receive_cb(const RemoteXBeeZB& remote, bool broadcast, const uint8_t *const data, uint16_t len) 00021 { 00022 const uint64_t remote_addr64 = remote.get_addr64(); 00023 00024 log_serial->printf("\r\nGot a %s RX packet [%08x:%08x|%04x], len %d\r\nData: ", broadcast ? "BROADCAST" : "UNICAST", UINT64_HI32(remote_addr64), UINT64_LO32(remote_addr64), remote.get_addr16(), len); 00025 00026 for (int i = 0; i < len; i++) 00027 log_serial->printf("%02x ", data[i]); 00028 00029 log_serial->printf("\r\n"); 00030 00031 switch(data[0]){ 00032 case 0x10: // speed + turnradius 00033 00034 int speed = int32_t((int8_t) data[1]); 00035 int turnspeed = int32_t((int8_t) data[2]); 00036 drive(speed); 00037 turn(turnspeed); 00038 stopper.attach(&stop, 0.1); 00039 break; 00040 } 00041 } 00042 00043 00044 int speed; 00045 00046 void init() 00047 { 00048 00049 } 00050 00051 void loop() 00052 { 00053 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00054 log_serial->baud(9600); 00055 log_serial->printf("Sample application to demo how to receive unicast and broadcast data with the XBeeZB\r\n\r\n"); 00056 log_serial->printf(XB_LIB_BANNER); 00057 00058 #if defined(ENABLE_LOGGING) 00059 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00060 #endif 00061 00062 XBeeZB xbee = XBeeZB(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00063 00064 /* Register callbacks */ 00065 xbee.register_receive_cb(&receive_cb); 00066 00067 RadioStatus const radioStatus = xbee.init(); 00068 MBED_ASSERT(radioStatus == Success); 00069 00070 /* Wait until the device has joined the network */ 00071 log_serial->printf("Waiting for device to join the network: "); 00072 while (!xbee.is_joined()) { 00073 wait_ms(1000); 00074 log_serial->printf("."); 00075 } 00076 log_serial->printf("OK\r\n"); 00077 led1 = 1; 00078 00079 while (true) { 00080 xbee.process_rx_frames(); 00081 } 00082 00083 delete(log_serial); 00084 00085 }
Generated on Wed Jul 13 2022 20:57:39 by
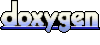