simple RGB led library
Dependents: m3Dpi MQTT-Thermostat-example Final_project_Tran Final_project_Tran ... more
RGB.h
00001 00002 #include "mbed.h" 00003 #include "Color.h" 00004 00005 #ifndef RGB_H 00006 #define RGB_H 00007 00008 00009 /** RGB class 00010 * Used to control RGB leds using PWM modulation to dim the individual colors. By combining red, green and blue 00011 * a great amount of colors can be created. This class can accept color objects or colors in hexadecimal notation (web color notation) 00012 * Example usage: 00013 * @code 00014 * 00015 * #include "mbed.h" 00016 * #include "RGB.h" 00017 * RGB led(p23,p24,p25); 00018 * 00019 * void main(){ 00020 * led.off(); 00021 * 00022 * wait(1.0); 00023 * 00024 * // setting the color using the Color enum with named colors 00025 * led.setColor(Color::RED); 00026 * 00027 * // setting the color using a hexadecimal notated integer (yellow) 00028 * led.setColor(0xFFFF00); 00029 * 00030 * // setting the color using an instance of the Color class 00031 * Color* myColor = new Color(0.0,1.0,0.0); 00032 * led.setColor(myColor); 00033 * delete myColor; 00034 * } 00035 * @endcode 00036 */ 00037 class RGB{ 00038 public: 00039 00040 static const int OFF = 0; 00041 00042 /** Create a new RGB instance 00043 * @param r_pin mbed PinName that supports PWM output assigned to the red led 00044 * @param g_pin mbed PinName that supports PWM output assigned to the green led 00045 * @param b_pin mbed PinName that supports PWM output assigned to the blue led 00046 */ 00047 RGB(PinName r_pin, PinName g_pin, PinName b_pin); 00048 ~RGB(); 00049 00050 /** Set the color by giving an instance of an Color object 00051 * @param color Pointer to an instance of an Color object 00052 * @ref Color 00053 */ 00054 00055 void setColor(Color* color); 00056 00057 /** Set the color by giving an integer in hexadecimal notation 00058 * @param color Color in hexadecimal notation (hex triplet). 24-bit RGB color as used in web colors. 00059 * @note Each color is made up of 8 bits, 0xRRGGBB 00060 */ 00061 void setColor(int color); 00062 00063 /** Get the current color of the RGB led 00064 * @return instance of Color class containing the current set color 00065 * @ref Color 00066 */ 00067 Color* getColor(); 00068 00069 00070 /// Turn the led off 00071 void off(); 00072 00073 private: 00074 00075 PwmOut* r_out; 00076 PwmOut* g_out; 00077 PwmOut* b_out; 00078 00079 Color* color; 00080 00081 void setPwmColor(int value, PwmOut* output); 00082 00083 }; 00084 00085 #endif
Generated on Tue Jul 12 2022 13:58:34 by
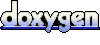