EmbUnit library for mbed. EmbUnit is a unit testing framework for embedded software. (more info: http://embunit.sourceforge.net/ )
stdImpl.cpp
00001 /* 00002 * COPYRIGHT AND PERMISSION NOTICE 00003 * 00004 * Copyright (c) 2003 Embedded Unit Project 00005 * 00006 * All rights reserved. 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining 00009 * a copy of this software and associated documentation files (the 00010 * "Software"), to deal in the Software without restriction, including 00011 * without limitation the rights to use, copy, modify, merge, publish, 00012 * distribute, and/or sell copies of the Software, and to permit persons 00013 * to whom the Software is furnished to do so, provided that the above 00014 * copyright notice(s) and this permission notice appear in all copies 00015 * of the Software and that both the above copyright notice(s) and this 00016 * permission notice appear in supporting documentation. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00019 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00020 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT 00021 * OF THIRD PARTY RIGHTS. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR 00022 * HOLDERS INCLUDED IN THIS NOTICE BE LIABLE FOR ANY CLAIM, OR ANY 00023 * SPECIAL INDIRECT OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER 00024 * RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF 00025 * CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN 00026 * CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00027 * 00028 * Except as contained in this notice, the name of a copyright holder 00029 * shall not be used in advertising or otherwise to promote the sale, 00030 * use or other dealings in this Software without prior written 00031 * authorization of the copyright holder. 00032 * 00033 * $Id: stdImpl.c,v 1.3 2004/02/10 16:15:25 arms22 Exp $ 00034 */ 00035 #include "stdImpl.h" 00036 00037 char* stdimpl_strcpy(char *dst, const char *src) 00038 { 00039 char *start = dst; 00040 char c; 00041 do { 00042 c = *src; 00043 *dst = c; 00044 src++; 00045 dst++; 00046 } while (c); 00047 return start; 00048 } 00049 00050 char* stdimpl_strcat(char *dst, const char *src) 00051 { 00052 char *start = dst; 00053 char c; 00054 do { 00055 c = *dst; 00056 dst++; 00057 } while (c); 00058 dst--; 00059 do { 00060 c = *src; 00061 *dst = c; 00062 src++; 00063 dst++; 00064 } while (c); 00065 return start; 00066 } 00067 00068 char* stdimpl_strncat(char *dst, const char *src,unsigned int count) 00069 { 00070 char *start = dst; 00071 char c; 00072 do { 00073 c = *dst; 00074 dst++; 00075 } while (c); 00076 dst--; 00077 if (count) { 00078 do { 00079 c = *src; 00080 *dst = c; 00081 src++; 00082 dst++; 00083 count--; 00084 } while (c && count); 00085 *dst = '\0'; 00086 } 00087 return start; 00088 } 00089 00090 int stdimpl_strlen(const char *str) 00091 { 00092 const char *estr = str; 00093 char c; 00094 do { 00095 c = *estr; 00096 estr++; 00097 } while (c); 00098 return ((int)(estr - str - 1)); 00099 } 00100 00101 int stdimpl_strcmp(const char *s1, const char *s2) 00102 { 00103 char c1,c2; 00104 do { 00105 c1 = *s1++; 00106 c2 = *s2++; 00107 } while ((c1) && (c2) && (c1==c2)); 00108 return c1 - c2; 00109 } 00110 00111 static char* _xtoa(unsigned long v,char *string, int r, int is_neg) 00112 { 00113 char *start = string; 00114 char buf[33],*p; 00115 00116 p = buf; 00117 00118 do { 00119 *p++ = "0123456789abcdef"[(v % r) & 0xf]; 00120 } while (v /= r); 00121 00122 if (is_neg) { 00123 *p++ = '-'; 00124 } 00125 00126 do { 00127 *string++ = *--p; 00128 } while (buf != p); 00129 00130 *string = '\0'; 00131 00132 return start; 00133 } 00134 00135 char* stdimpl_itoa(int v,char *string,int r) 00136 { 00137 if ((r == 10) && (v < 0)) { 00138 return _xtoa((unsigned long)(-v), string, r, 1); 00139 } 00140 return _xtoa((unsigned long)(v), string, r, 0); 00141 }
Generated on Wed Jul 13 2022 18:17:56 by
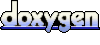