EmbUnit library for mbed. EmbUnit is a unit testing framework for embedded software. (more info: http://embunit.sourceforge.net/ )
TestRunner.cpp
00001 /* 00002 * COPYRIGHT AND PERMISSION NOTICE 00003 * 00004 * Copyright (c) 2003 Embedded Unit Project 00005 * 00006 * All rights reserved. 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining 00009 * a copy of this software and associated documentation files (the 00010 * "Software"), to deal in the Software without restriction, including 00011 * without limitation the rights to use, copy, modify, merge, publish, 00012 * distribute, and/or sell copies of the Software, and to permit persons 00013 * to whom the Software is furnished to do so, provided that the above 00014 * copyright notice(s) and this permission notice appear in all copies 00015 * of the Software and that both the above copyright notice(s) and this 00016 * permission notice appear in supporting documentation. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00019 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00020 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT 00021 * OF THIRD PARTY RIGHTS. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR 00022 * HOLDERS INCLUDED IN THIS NOTICE BE LIABLE FOR ANY CLAIM, OR ANY 00023 * SPECIAL INDIRECT OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER 00024 * RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF 00025 * CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN 00026 * CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00027 * 00028 * Except as contained in this notice, the name of a copyright holder 00029 * shall not be used in advertising or otherwise to promote the sale, 00030 * use or other dealings in this Software without prior written 00031 * authorization of the copyright holder. 00032 * 00033 * $Id: TestRunner.c,v 1.6 2004/02/10 16:19:29 arms22 Exp $ 00034 */ 00035 #include "config.h" 00036 #include "stdImpl.h" 00037 #include "Test.h" 00038 #include "TestListener.h" 00039 #include "TestResult.h" 00040 #include "TestRunner.h" 00041 00042 static TestResult result_; 00043 static Test* root_; 00044 00045 static void TestRunner_startTest(TestListner* self,Test* test) 00046 { 00047 stdimpl_print("."); 00048 } 00049 00050 static void TestRunner_endTest(TestListner* self,Test* test) 00051 { 00052 } 00053 00054 static void TestRunner_addFailure(TestListner* self,Test* test,char* msg,int line,char* file) 00055 { 00056 stdimpl_print("\r\n"); 00057 stdimpl_print(Test_name(root_)); 00058 stdimpl_print("."); 00059 stdimpl_print(Test_name(test)); 00060 { 00061 char buf[16]; 00062 stdimpl_print(" ("); 00063 stdimpl_print(file); 00064 stdimpl_print(" "); 00065 stdimpl_itoa(line, buf, 10); 00066 stdimpl_print(buf); 00067 stdimpl_print(") "); 00068 } 00069 stdimpl_print(msg); 00070 stdimpl_print("\r\n"); 00071 } 00072 00073 static const TestListnerImplement TestRunnerImplement = { 00074 (TestListnerStartTestCallBack) TestRunner_startTest, 00075 (TestListnerEndTestCallBack) TestRunner_endTest, 00076 (TestListnerAddFailureCallBack) TestRunner_addFailure, 00077 }; 00078 00079 static const TestListner testrunner_ = { 00080 (TestListnerImplement*)&TestRunnerImplement, 00081 }; 00082 00083 void TestRunner_start(void) 00084 { 00085 stdimpl_print("---Start EmbUnit---\r\n"); 00086 TestResult_init(&result_, (TestListner*)&testrunner_); 00087 } 00088 00089 void TestRunner_runTest(Test* test) 00090 { 00091 root_ = test; 00092 Test_run(test, &result_); 00093 } 00094 00095 void TestRunner_end(void) 00096 { 00097 char buf[16]; 00098 if (result_.failureCount) { 00099 stdimpl_print("\r\nrun "); 00100 stdimpl_itoa(result_.runCount, buf, 10); 00101 stdimpl_print(buf); 00102 stdimpl_print(" failures "); 00103 stdimpl_itoa(result_.failureCount, buf, 10); 00104 stdimpl_print(buf); 00105 stdimpl_print("\r\n"); 00106 } else { 00107 stdimpl_print("\r\nOK ("); 00108 stdimpl_itoa(result_.runCount, buf, 10); 00109 stdimpl_print(buf); 00110 stdimpl_print(" tests)\r\n"); 00111 } 00112 }
Generated on Wed Jul 13 2022 18:17:56 by
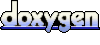