EmbUnit library for mbed. EmbUnit is a unit testing framework for embedded software. (more info: http://embunit.sourceforge.net/ )
TestCaller.h
00001 /* 00002 * COPYRIGHT AND PERMISSION NOTICE 00003 * 00004 * Copyright (c) 2003 Embedded Unit Project 00005 * 00006 * All rights reserved. 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining 00009 * a copy of this software and associated documentation files (the 00010 * "Software"), to deal in the Software without restriction, including 00011 * without limitation the rights to use, copy, modify, merge, publish, 00012 * distribute, and/or sell copies of the Software, and to permit persons 00013 * to whom the Software is furnished to do so, provided that the above 00014 * copyright notice(s) and this permission notice appear in all copies 00015 * of the Software and that both the above copyright notice(s) and this 00016 * permission notice appear in supporting documentation. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00019 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00020 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT 00021 * OF THIRD PARTY RIGHTS. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR 00022 * HOLDERS INCLUDED IN THIS NOTICE BE LIABLE FOR ANY CLAIM, OR ANY 00023 * SPECIAL INDIRECT OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER 00024 * RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF 00025 * CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN 00026 * CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00027 * 00028 * Except as contained in this notice, the name of a copyright holder 00029 * shall not be used in advertising or otherwise to promote the sale, 00030 * use or other dealings in this Software without prior written 00031 * authorization of the copyright holder. 00032 * 00033 * $Id: TestCaller.h,v 1.7 2004/02/10 16:19:29 arms22 Exp $ 00034 */ 00035 #ifndef __TESTCALLER_H__ 00036 #define __TESTCALLER_H__ 00037 00038 typedef struct __TestFixture TestFixture; 00039 typedef struct __TestFixture* TestFixtureRef;/*downward compatible*/ 00040 00041 struct __TestFixture { 00042 char *name; 00043 void(*test)(void); 00044 }; 00045 00046 #define new_TestFixture(name,test)\ 00047 {\ 00048 name,\ 00049 test,\ 00050 } 00051 00052 typedef struct __TestCaller TestCaller; 00053 typedef struct __TestCaller* TestCallerRef;/*downward compatible*/ 00054 00055 struct __TestCaller { 00056 TestImplement* isa; 00057 char *name; 00058 void(*setUp)(void); 00059 void(*tearDown)(void); 00060 int numberOfFixtuers; 00061 TestFixture *fixtuers; 00062 }; 00063 00064 extern const TestImplement TestCallerImplement; 00065 00066 #define new_TestCaller(name,sup,tdw,numberOfFixtuers,fixtuers)\ 00067 {\ 00068 (TestImplement*)&TestCallerImplement,\ 00069 name,\ 00070 sup,\ 00071 tdw,\ 00072 numberOfFixtuers,\ 00073 fixtuers,\ 00074 } 00075 00076 #endif/*__TESTCALLER_H__*/
Generated on Wed Jul 13 2022 18:17:56 by
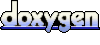