
AirsoftTimer software based on mbed
Dependencies: mbed TextLCD keypad
Board.h
00001 #ifndef BOARD_H 00002 #define BOARD_H 00003 00004 #include "LCD.h" 00005 #include "Leds.h" 00006 #include "Key.h" 00007 #include "Buzzer.h" 00008 #include "Button.h" 00009 #include "Keyboard.h" 00010 00011 #include "FPointer.h" 00012 00013 // class for debug leds 00014 // class for eeprom memory 00015 // class for wireless (nordic chip or xbee) 00016 00017 struct Pinouts{ 00018 PinName button; 00019 PinName key; 00020 LedPins leds; 00021 PinName buzzer; 00022 KeyboardPins keyboard; 00023 LcdPins lcd; 00024 }; 00025 00026 class Board{ 00027 public: 00028 Board(Pinouts pinouts); 00029 00030 LCD* lcd; 00031 Leds* leds; 00032 Key* key; 00033 Button* button; 00034 Keyboard* keyboard; 00035 Buzzer* buzzer; 00036 00037 uint32_t keyboardButton(uint32_t index); 00038 00039 template<class T> 00040 void attach(T* item, uint32_t(T::*method)(uint32_t)){ 00041 _callback.attach(item, method); 00042 } 00043 00044 protected: 00045 FPointer _callback; // Called after each input 00046 00047 00048 }; 00049 00050 #endif
Generated on Tue Jul 12 2022 21:04:09 by
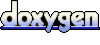