
Dummy program to demonstrate problems: working code
Dependencies: SLCD mbed-rtos mbed
Fork of MNG_TC by
ThreadFunctions.h
00001 void reset_all(){ 00002 printf("resetting all\r\n"); 00003 // reset MNG_TC 00004 MNG_TC::init(); 00005 TC_list *tcp = VAR_SPACE::Head_node; 00006 while(tcp != NULL){ 00007 TC_list *temp = tcp->next_TC; 00008 delete tcp; 00009 tcp = temp; 00010 } 00011 00012 // reset COM_RCV_TC 00013 // handle reset 00014 00015 // reset data linked list 00016 data_list *dataptr = VAR_SPACE::head_data; 00017 while( dataptr != NULL ){ 00018 data_list *temp = dataptr->next; 00019 delete dataptr; 00020 dataptr = temp; 00021 } 00022 VAR_SPACE::head_data = new data_list; 00023 VAR_SPACE::rx_new_node = VAR_SPACE::head_data; 00024 } 00025 00026 void after_receive(void){ 00027 rx_timeout.detach(); 00028 VAR_SPACE::rx_new_node->val = 0x00; 00029 00030 if(first_time){ 00031 first_time = false; 00032 pass_time.attach(&after_pass, PASS_TIME_LIMIT); 00033 } 00034 00035 VAR_SPACE::new_tc_received = true; 00036 VAR_SPACE::mng_tmtc_thread->signal_set(0x01); 00037 } 00038 00039 00040 void com_rcv_tc_fun(const void *args){ 00041 00042 while(true){ 00043 Thread::signal_wait(0x01); 00044 00045 //~ allocate new node 00046 VAR_SPACE::rx_new_node->next = new data_list; 00047 VAR_SPACE::rx_new_node = VAR_SPACE::rx_new_node->next; 00048 VAR_SPACE::rx_new_node->next = NULL; 00049 00050 rx_timeout.attach(&after_receive, RX_TIMEOUT_LIMIT); 00051 } 00052 } 00053 00054 void com_mng_fun(const void *args){ 00055 while(true){ 00056 00057 Thread::signal_wait(0x01); 00058 ledr = !ledr; 00059 00060 if( VAR_SPACE::new_tc_received ){ 00061 VAR_SPACE::new_tc_received = false; 00062 struct data_list *haha = VAR_SPACE::head_data; 00063 00064 unsigned int count = 0; 00065 while( haha != NULL ){ 00066 ++count; 00067 printf("%x ", haha->val); 00068 haha = haha->next; 00069 } 00070 printf("\t count = %u \r\n", count); 00071 printf("new tc received : state = %u\r\n", VAR_SPACE::rx_state); 00072 00073 if( VAR_SPACE::rx_state == 0 ){ 00074 VAR_SPACE::data_node = VAR_SPACE::head_data; 00075 COM_RCV_TC::rx_rcv_tc(); 00076 MNG_TC::start_with(); 00077 if( MNG_TC::check_for_missing_TC() ){ 00078 printf("everything pass\r\n"); 00079 VAR_SPACE::rx_state = 1; 00080 MNG_TC::execute_TC(); 00081 } 00082 } 00083 else if( VAR_SPACE::rx_state == 3 ){ 00084 VAR_SPACE::data_node = VAR_SPACE::head_data; 00085 COM_RCV_TC::rx_rcv_tc(); 00086 MNG_TC::start_with(); 00087 if( MNG_TC::check_for_missing_TC() ){ 00088 VAR_SPACE::execute_obosc = true; 00089 VAR_SPACE::rx_state = 2; 00090 MNG_TC::execute_TC(); 00091 } 00092 } 00093 else{ 00094 // invalid state in main found reset 00095 reset_all(); 00096 } 00097 } 00098 } 00099 } 00100 00101 00102 //void com_pay_thread_fun(void const *args){ 00103 // while(true){ 00104 // VAR_SPACE::COM_payload_thread->signal_wait(0x01); 00105 // //read rtc time later 00106 // uint64_t RTC_time = 0; 00107 // Science_Data_Compression::complete_compression( VAR_SPACE::payload_dma_buffer , RTC_time); 00108 // } 00109 //}
Generated on Thu Jul 21 2022 03:45:37 by
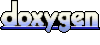