
Dummy program to demonstrate problems: working code
Dependencies: SLCD mbed-rtos mbed
Fork of MNG_TC by
COM_RCV_TC.h
00001 namespace COM_RCV_TC{ 00002 unsigned int bytes_read = 0; 00003 unsigned int tc_frames = 0; 00004 unsigned char tempString[136]; 00005 TC_list *return_this = NULL; 00006 00007 void inline attach_frame(unsigned int length){ 00008 // allocate new node 00009 // handle Head node 00010 if( tc_frames == 0 ){ 00011 VAR_SPACE::Head_node = new TC_list; 00012 VAR_SPACE::Head_node->next_TC = NULL; 00013 VAR_SPACE::last_node = VAR_SPACE::Head_node; 00014 } 00015 else{ 00016 VAR_SPACE::last_node->next_TC = new TC_list; 00017 VAR_SPACE::last_node = VAR_SPACE::last_node->next_TC; 00018 VAR_SPACE::last_node->next_TC = NULL; 00019 } 00020 00021 // allocate memory for string 00022 VAR_SPACE::last_node->TC_string = new unsigned char[length]; 00023 for(int i = 0 ; i < length ; ++i){ 00024 VAR_SPACE::last_node->TC_string[i] = tempString[i]; 00025 } 00026 00027 // short or long 00028 VAR_SPACE::last_node->short_or_long = (length == 11) ? true : false; 00029 00030 // crc pass or fail 00031 uint16_t crc_checksum = CRC::crc16_gen(tempString, length-2); 00032 if( ( (crc_checksum & 0xFF) == tempString[length-1]) && ( ((crc_checksum >> 8) & 0xFF) == tempString[length-2] ) ){ 00033 VAR_SPACE::last_node->crc_pass = true; 00034 } 00035 else{ 00036 VAR_SPACE::last_node->crc_pass = false; 00037 } 00038 00039 // PSC 00040 VAR_SPACE::last_node->packet_seq_count = VAR_SPACE::last_node->TC_string[0]; 00041 00042 // apid 00043 unsigned char tempChar = VAR_SPACE::last_node->TC_string[1]; 00044 VAR_SPACE::last_node->apid = (tempChar >> 6) & 3; 00045 00046 // abort on nack 00047 VAR_SPACE::last_node->abort_on_nack = (((tempChar >> 3) & 1) == 1) ? true : false; 00048 00049 // default values of enable and execution status 00050 VAR_SPACE::last_node->enabled = true; 00051 VAR_SPACE::last_node->exec_status = 0; 00052 00053 // printf("inside attach frame : frame num = %u\r\n", tc_frames); 00054 00055 // for(int i = 0 ; i < length ; ++i){ 00056 // std::bitset<8> b = VAR_SPACE::last_node->TC_string[i]; 00057 // cout << b << " "; 00058 // } 00059 // cout << ENDL; 00060 // for( int i = 0 ; i < length ; ++i){ 00061 // std::bitset<8> b = tempString[i]; 00062 // cout << b << " "; 00063 // } 00064 // cout << ENDL; 00065 00066 ++tc_frames; 00067 } 00068 00069 void flushData(const unsigned int& bytes, const unsigned char& outState){ 00070 if( (bytes == 11) && (outState == 7) ){ 00071 attach_frame(11); 00072 } 00073 else if( (bytes == 135) && (outState == 7) ){ 00074 attach_frame(135); 00075 } 00076 } 00077 00078 void rx_rcv_tc(void){ 00079 bool frame_started = false; 00080 bytes_read = 0; 00081 unsigned char state7e = 0; 00082 unsigned char outState = 0; 00083 unsigned int outByte = 0; 00084 bool chain_started = false; 00085 unsigned int byteCount = 0; 00086 00087 // read byte by byte 00088 while( VAR_SPACE::data_node != NULL ){ 00089 00090 unsigned char test_this = VAR_SPACE::data_node->val; 00091 ++bytes_read; 00092 00093 struct data_list *temp = VAR_SPACE::data_node->next; 00094 delete VAR_SPACE::data_node; 00095 VAR_SPACE::data_node = temp; 00096 if( bytes_read == 1 ){ 00097 VAR_SPACE::head_data = new struct data_list; 00098 VAR_SPACE::head_data->next = NULL; 00099 VAR_SPACE::rx_new_node = VAR_SPACE::head_data; 00100 } 00101 00102 // read bit by bit 00103 for(int i = 7 ; i >= 0 ; --i){ 00104 unsigned char tempBit = (test_this >> i) & 1; 00105 bool skipIteration = false; 00106 00107 if( tempBit == 1 ){ 00108 switch( state7e ){ 00109 case 0: 00110 state7e = 0; 00111 break; 00112 case 1: 00113 state7e = 2; 00114 break; 00115 case 2: 00116 state7e = 3; 00117 break; 00118 case 3: 00119 state7e = 4; 00120 break; 00121 case 4: 00122 state7e = 5; 00123 break; 00124 case 5: 00125 state7e = 6; 00126 break; 00127 case 6: 00128 state7e = 7; 00129 break; 00130 case 7: 00131 // error reset 00132 state7e = 0; 00133 chain_started = false; 00134 frame_started = false; 00135 byteCount = 0; 00136 outByte = 0; 00137 outState = 0; 00138 skipIteration = true; 00139 break; 00140 } 00141 } 00142 else{ 00143 switch( state7e ){ 00144 case 0: 00145 case 1: 00146 case 2: 00147 case 3: 00148 case 4: 00149 case 5: 00150 state7e = 1; 00151 break; 00152 case 6: 00153 state7e = 1; 00154 skipIteration = true; 00155 break; 00156 case 7: 00157 state7e = 0; 00158 // detected 7e 00159 // printf("detected 7e : chain start : %u, frame_start : %u\r\n", (chain_started ? 1 : 0), (frame_started ? 1 : 0) ); 00160 if( !chain_started ){ 00161 chain_started = true; 00162 frame_started = true; 00163 byteCount = 0; 00164 outByte = 0; 00165 outState = 0; 00166 skipIteration = true; 00167 } 00168 else{ 00169 flushData(byteCount, outState); 00170 byteCount = 0; 00171 outState = 0; 00172 outByte = 0; 00173 skipIteration = true; 00174 } 00175 break; 00176 } 00177 } 00178 if( (!skipIteration) && (frame_started) ){ 00179 // write bit to output 00180 switch( outState ){ 00181 case 0: 00182 outState = 1; 00183 tempString[outByte] = tempBit << 7; 00184 break; 00185 case 1: 00186 outState = 2; 00187 tempString[outByte] += tempBit << 6; 00188 break; 00189 case 2: 00190 outState = 3; 00191 tempString[outByte] += tempBit << 5; 00192 break; 00193 case 3: 00194 outState = 4; 00195 tempString[outByte] += tempBit << 4; 00196 break; 00197 case 4: 00198 outState = 5; 00199 tempString[outByte] += tempBit << 3; 00200 break; 00201 case 5: 00202 outState = 6; 00203 tempString[outByte] += tempBit << 2; 00204 break; 00205 case 6: 00206 outState = 7; 00207 tempString[outByte] += tempBit << 1; 00208 break; 00209 case 7: 00210 // printf("wrote a byte in tempString\r\n"); 00211 outState = 0; 00212 tempString[outByte] += tempBit; 00213 ++outByte; 00214 // exceeded tc length discard 00215 if(outByte > 135){ 00216 outByte = 0; 00217 } 00218 ++byteCount; 00219 break; 00220 } 00221 } 00222 } 00223 } 00224 } 00225 }
Generated on Thu Jul 21 2022 03:45:37 by
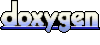