First Publish. Works fine.
Dependents: unzen_sample_LPC4088_quickstart
unzen_hal.h
00001 #ifndef _UNZEN_HAL_H_ 00002 #define _UNZEN_HAL_H_ 00003 00004 #include "mbed.h" 00005 00006 namespace unzen 00007 { 00008 // Set up I2S peripheral to ready to start. 00009 // By this HAL, the I2S have to become : 00010 // - slave mode 00011 // - clock must be ready 00012 void hal_i2s_setup(void); 00013 00014 // configure the pins of I2S and then, wait for WS. 00015 // This waiting is important to avoid the delay between TX and RX. 00016 // The HAL API will wait for the WS changes from left to right then return. 00017 // The procesure is : 00018 // 1. configure WS pin as GPIO 00019 // 2. wait the WS rising edge 00020 // 3. configure all pins as I2S 00021 void hal_i2s_pin_config_and_wait_ws(void); 00022 00023 00024 // Start I2S transfer. Interrupt starts 00025 void hal_i2s_start(void); 00026 00027 // returns the IRQ ID for I2S RX interrupt 00028 IRQn_Type hal_get_i2s_irq_id(void); 00029 00030 // returns the IRQ ID for process IRQ. Typically, this is allocated to the reserved IRQ. 00031 IRQn_Type hal_get_process_irq_id(void); 00032 00033 // The returned value must be compatible with CMSIS NVIC_SetPriority() API. That mean, it is integer like 0, 1, 2... 00034 unsigned int hal_get_i2s_irq_priority_level(void); 00035 00036 // The returned value must be compatible with CMSIS NVIC_SetPriority() API. That mean, it is integer like 0, 1, 2... 00037 unsigned int hal_get_process_irq_priority_level(void); 00038 00039 00040 // reutun the intenger value which tells how much data have to be transfered for each 00041 // interrupt. For example, if the stereo 32bit data ( total 64 bit ) have to be sent, 00042 // have to return 2. 00043 unsigned int hal_data_per_sample(void); 00044 00045 // get data from I2S RX peripheral. Where sample is one audio data. Stereo data is constructed by 2 samples. 00046 void hal_get_i2s_rx_data( int & sample); 00047 00048 // put data into I2S TX peripheral. Where sample is one audio data. Stereo data is constructed by 2 samples. 00049 void hal_put_i2s_tx_data( int sample ); 00050 } 00051 00052 00053 #endif
Generated on Wed Jul 13 2022 06:48:06 by
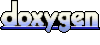