
Final 350 project
Dependencies: uzair Camera_LS_Y201 F7_Ethernet LCD_DISCO_F746NG NetworkAPI SDFileSystem mbed
jdarith.c
00001 /* 00002 * jdarith.c 00003 * 00004 * Developed 1997-2015 by Guido Vollbeding. 00005 * This file is part of the Independent JPEG Group's software. 00006 * For conditions of distribution and use, see the accompanying README file. 00007 * 00008 * This file contains portable arithmetic entropy decoding routines for JPEG 00009 * (implementing the ISO/IEC IS 10918-1 and CCITT Recommendation ITU-T T.81). 00010 * 00011 * Both sequential and progressive modes are supported in this single module. 00012 * 00013 * Suspension is not currently supported in this module. 00014 */ 00015 00016 #define JPEG_INTERNALS 00017 #include "jinclude.h" 00018 #include "jpeglib.h" 00019 00020 00021 /* Expanded entropy decoder object for arithmetic decoding. */ 00022 00023 typedef struct { 00024 struct jpeg_entropy_decoder pub; /* public fields */ 00025 00026 INT32 c; /* C register, base of coding interval + input bit buffer */ 00027 INT32 a; /* A register, normalized size of coding interval */ 00028 int ct; /* bit shift counter, # of bits left in bit buffer part of C */ 00029 /* init: ct = -16 */ 00030 /* run: ct = 0..7 */ 00031 /* error: ct = -1 */ 00032 int last_dc_val[MAX_COMPS_IN_SCAN]; /* last DC coef for each component */ 00033 int dc_context[MAX_COMPS_IN_SCAN]; /* context index for DC conditioning */ 00034 00035 unsigned int restarts_to_go; /* MCUs left in this restart interval */ 00036 00037 /* Pointers to statistics areas (these workspaces have image lifespan) */ 00038 unsigned char * dc_stats[NUM_ARITH_TBLS]; 00039 unsigned char * ac_stats[NUM_ARITH_TBLS]; 00040 00041 /* Statistics bin for coding with fixed probability 0.5 */ 00042 unsigned char fixed_bin[4]; 00043 } arith_entropy_decoder; 00044 00045 typedef arith_entropy_decoder * arith_entropy_ptr; 00046 00047 /* The following two definitions specify the allocation chunk size 00048 * for the statistics area. 00049 * According to sections F.1.4.4.1.3 and F.1.4.4.2, we need at least 00050 * 49 statistics bins for DC, and 245 statistics bins for AC coding. 00051 * 00052 * We use a compact representation with 1 byte per statistics bin, 00053 * thus the numbers directly represent byte sizes. 00054 * This 1 byte per statistics bin contains the meaning of the MPS 00055 * (more probable symbol) in the highest bit (mask 0x80), and the 00056 * index into the probability estimation state machine table 00057 * in the lower bits (mask 0x7F). 00058 */ 00059 00060 #define DC_STAT_BINS 64 00061 #define AC_STAT_BINS 256 00062 00063 00064 LOCAL(int) 00065 get_byte (j_decompress_ptr cinfo) 00066 /* Read next input byte; we do not support suspension in this module. */ 00067 { 00068 struct jpeg_source_mgr * src = cinfo->src; 00069 00070 if (src->bytes_in_buffer == 0) 00071 if (! (*src->fill_input_buffer) (cinfo)) 00072 ERREXIT(cinfo, JERR_CANT_SUSPEND); 00073 src->bytes_in_buffer--; 00074 return GETJOCTET(*src->next_input_byte++); 00075 } 00076 00077 00078 /* 00079 * The core arithmetic decoding routine (common in JPEG and JBIG). 00080 * This needs to go as fast as possible. 00081 * Machine-dependent optimization facilities 00082 * are not utilized in this portable implementation. 00083 * However, this code should be fairly efficient and 00084 * may be a good base for further optimizations anyway. 00085 * 00086 * Return value is 0 or 1 (binary decision). 00087 * 00088 * Note: I've changed the handling of the code base & bit 00089 * buffer register C compared to other implementations 00090 * based on the standards layout & procedures. 00091 * While it also contains both the actual base of the 00092 * coding interval (16 bits) and the next-bits buffer, 00093 * the cut-point between these two parts is floating 00094 * (instead of fixed) with the bit shift counter CT. 00095 * Thus, we also need only one (variable instead of 00096 * fixed size) shift for the LPS/MPS decision, and 00097 * we can do away with any renormalization update 00098 * of C (except for new data insertion, of course). 00099 * 00100 * I've also introduced a new scheme for accessing 00101 * the probability estimation state machine table, 00102 * derived from Markus Kuhn's JBIG implementation. 00103 */ 00104 00105 LOCAL(int) 00106 arith_decode (j_decompress_ptr cinfo, unsigned char *st) 00107 { 00108 register arith_entropy_ptr e = (arith_entropy_ptr) cinfo->entropy; 00109 register unsigned char nl, nm; 00110 register INT32 qe, temp; 00111 register int sv, data; 00112 00113 /* Renormalization & data input per section D.2.6 */ 00114 while (e->a < 0x8000L) { 00115 if (--e->ct < 0) { 00116 /* Need to fetch next data byte */ 00117 if (cinfo->unread_marker) 00118 data = 0; /* stuff zero data */ 00119 else { 00120 data = get_byte(cinfo); /* read next input byte */ 00121 if (data == 0xFF) { /* zero stuff or marker code */ 00122 do data = get_byte(cinfo); 00123 while (data == 0xFF); /* swallow extra 0xFF bytes */ 00124 if (data == 0) 00125 data = 0xFF; /* discard stuffed zero byte */ 00126 else { 00127 /* Note: Different from the Huffman decoder, hitting 00128 * a marker while processing the compressed data 00129 * segment is legal in arithmetic coding. 00130 * The convention is to supply zero data 00131 * then until decoding is complete. 00132 */ 00133 cinfo->unread_marker = data; 00134 data = 0; 00135 } 00136 } 00137 } 00138 e->c = (e->c << 8) | data; /* insert data into C register */ 00139 if ((e->ct += 8) < 0) /* update bit shift counter */ 00140 /* Need more initial bytes */ 00141 if (++e->ct == 0) 00142 /* Got 2 initial bytes -> re-init A and exit loop */ 00143 e->a = 0x8000L; /* => e->a = 0x10000L after loop exit */ 00144 } 00145 e->a <<= 1; 00146 } 00147 00148 /* Fetch values from our compact representation of Table D.3(D.2): 00149 * Qe values and probability estimation state machine 00150 */ 00151 sv = *st; 00152 qe = jpeg_aritab[sv & 0x7F]; /* => Qe_Value */ 00153 nl = qe & 0xFF; qe >>= 8; /* Next_Index_LPS + Switch_MPS */ 00154 nm = qe & 0xFF; qe >>= 8; /* Next_Index_MPS */ 00155 00156 /* Decode & estimation procedures per sections D.2.4 & D.2.5 */ 00157 temp = e->a - qe; 00158 e->a = temp; 00159 temp <<= e->ct; 00160 if (e->c >= temp) { 00161 e->c -= temp; 00162 /* Conditional LPS (less probable symbol) exchange */ 00163 if (e->a < qe) { 00164 e->a = qe; 00165 *st = (sv & 0x80) ^ nm; /* Estimate_after_MPS */ 00166 } else { 00167 e->a = qe; 00168 *st = (sv & 0x80) ^ nl; /* Estimate_after_LPS */ 00169 sv ^= 0x80; /* Exchange LPS/MPS */ 00170 } 00171 } else if (e->a < 0x8000L) { 00172 /* Conditional MPS (more probable symbol) exchange */ 00173 if (e->a < qe) { 00174 *st = (sv & 0x80) ^ nl; /* Estimate_after_LPS */ 00175 sv ^= 0x80; /* Exchange LPS/MPS */ 00176 } else { 00177 *st = (sv & 0x80) ^ nm; /* Estimate_after_MPS */ 00178 } 00179 } 00180 00181 return sv >> 7; 00182 } 00183 00184 00185 /* 00186 * Check for a restart marker & resynchronize decoder. 00187 */ 00188 00189 LOCAL(void) 00190 process_restart (j_decompress_ptr cinfo) 00191 { 00192 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00193 int ci; 00194 jpeg_component_info * compptr; 00195 00196 /* Advance past the RSTn marker */ 00197 if (! (*cinfo->marker->read_restart_marker) (cinfo)) 00198 ERREXIT(cinfo, JERR_CANT_SUSPEND); 00199 00200 /* Re-initialize statistics areas */ 00201 for (ci = 0; ci < cinfo->comps_in_scan; ci++) { 00202 compptr = cinfo->cur_comp_info[ci]; 00203 if (! cinfo->progressive_mode || (cinfo->Ss == 0 && cinfo->Ah == 0)) { 00204 MEMZERO(entropy->dc_stats[compptr->dc_tbl_no], DC_STAT_BINS); 00205 /* Reset DC predictions to 0 */ 00206 entropy->last_dc_val[ci] = 0; 00207 entropy->dc_context[ci] = 0; 00208 } 00209 if ((! cinfo->progressive_mode && cinfo->lim_Se) || 00210 (cinfo->progressive_mode && cinfo->Ss)) { 00211 MEMZERO(entropy->ac_stats[compptr->ac_tbl_no], AC_STAT_BINS); 00212 } 00213 } 00214 00215 /* Reset arithmetic decoding variables */ 00216 entropy->c = 0; 00217 entropy->a = 0; 00218 entropy->ct = -16; /* force reading 2 initial bytes to fill C */ 00219 00220 /* Reset restart counter */ 00221 entropy->restarts_to_go = cinfo->restart_interval; 00222 } 00223 00224 00225 /* 00226 * Arithmetic MCU decoding. 00227 * Each of these routines decodes and returns one MCU's worth of 00228 * arithmetic-compressed coefficients. 00229 * The coefficients are reordered from zigzag order into natural array order, 00230 * but are not dequantized. 00231 * 00232 * The i'th block of the MCU is stored into the block pointed to by 00233 * MCU_data[i]. WE ASSUME THIS AREA IS INITIALLY ZEROED BY THE CALLER. 00234 */ 00235 00236 /* 00237 * MCU decoding for DC initial scan (either spectral selection, 00238 * or first pass of successive approximation). 00239 */ 00240 00241 METHODDEF(boolean) 00242 decode_mcu_DC_first (j_decompress_ptr cinfo, JBLOCKROW *MCU_data) 00243 { 00244 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00245 JBLOCKROW block; 00246 unsigned char *st; 00247 int blkn, ci, tbl, sign; 00248 int v, m; 00249 00250 /* Process restart marker if needed */ 00251 if (cinfo->restart_interval) { 00252 if (entropy->restarts_to_go == 0) 00253 process_restart(cinfo); 00254 entropy->restarts_to_go--; 00255 } 00256 00257 if (entropy->ct == -1) return TRUE; /* if error do nothing */ 00258 00259 /* Outer loop handles each block in the MCU */ 00260 00261 for (blkn = 0; blkn < cinfo->blocks_in_MCU; blkn++) { 00262 block = MCU_data[blkn]; 00263 ci = cinfo->MCU_membership[blkn]; 00264 tbl = cinfo->cur_comp_info[ci]->dc_tbl_no; 00265 00266 /* Sections F.2.4.1 & F.1.4.4.1: Decoding of DC coefficients */ 00267 00268 /* Table F.4: Point to statistics bin S0 for DC coefficient coding */ 00269 st = entropy->dc_stats[tbl] + entropy->dc_context[ci]; 00270 00271 /* Figure F.19: Decode_DC_DIFF */ 00272 if (arith_decode(cinfo, st) == 0) 00273 entropy->dc_context[ci] = 0; 00274 else { 00275 /* Figure F.21: Decoding nonzero value v */ 00276 /* Figure F.22: Decoding the sign of v */ 00277 sign = arith_decode(cinfo, st + 1); 00278 st += 2; st += sign; 00279 /* Figure F.23: Decoding the magnitude category of v */ 00280 if ((m = arith_decode(cinfo, st)) != 0) { 00281 st = entropy->dc_stats[tbl] + 20; /* Table F.4: X1 = 20 */ 00282 while (arith_decode(cinfo, st)) { 00283 if ((m <<= 1) == 0x8000) { 00284 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00285 entropy->ct = -1; /* magnitude overflow */ 00286 return TRUE; 00287 } 00288 st += 1; 00289 } 00290 } 00291 /* Section F.1.4.4.1.2: Establish dc_context conditioning category */ 00292 if (m < (int) ((1L << cinfo->arith_dc_L[tbl]) >> 1)) 00293 entropy->dc_context[ci] = 0; /* zero diff category */ 00294 else if (m > (int) ((1L << cinfo->arith_dc_U[tbl]) >> 1)) 00295 entropy->dc_context[ci] = 12 + (sign * 4); /* large diff category */ 00296 else 00297 entropy->dc_context[ci] = 4 + (sign * 4); /* small diff category */ 00298 v = m; 00299 /* Figure F.24: Decoding the magnitude bit pattern of v */ 00300 st += 14; 00301 while (m >>= 1) 00302 if (arith_decode(cinfo, st)) v |= m; 00303 v += 1; if (sign) v = -v; 00304 entropy->last_dc_val[ci] += v; 00305 } 00306 00307 /* Scale and output the DC coefficient (assumes jpeg_natural_order[0]=0) */ 00308 (*block)[0] = (JCOEF) (entropy->last_dc_val[ci] << cinfo->Al); 00309 } 00310 00311 return TRUE; 00312 } 00313 00314 00315 /* 00316 * MCU decoding for AC initial scan (either spectral selection, 00317 * or first pass of successive approximation). 00318 */ 00319 00320 METHODDEF(boolean) 00321 decode_mcu_AC_first (j_decompress_ptr cinfo, JBLOCKROW *MCU_data) 00322 { 00323 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00324 JBLOCKROW block; 00325 unsigned char *st; 00326 int tbl, sign, k; 00327 int v, m; 00328 const int * natural_order; 00329 00330 /* Process restart marker if needed */ 00331 if (cinfo->restart_interval) { 00332 if (entropy->restarts_to_go == 0) 00333 process_restart(cinfo); 00334 entropy->restarts_to_go--; 00335 } 00336 00337 if (entropy->ct == -1) return TRUE; /* if error do nothing */ 00338 00339 natural_order = cinfo->natural_order; 00340 00341 /* There is always only one block per MCU */ 00342 block = MCU_data[0]; 00343 tbl = cinfo->cur_comp_info[0]->ac_tbl_no; 00344 00345 /* Sections F.2.4.2 & F.1.4.4.2: Decoding of AC coefficients */ 00346 00347 /* Figure F.20: Decode_AC_coefficients */ 00348 k = cinfo->Ss - 1; 00349 do { 00350 st = entropy->ac_stats[tbl] + 3 * k; 00351 if (arith_decode(cinfo, st)) break; /* EOB flag */ 00352 for (;;) { 00353 k++; 00354 if (arith_decode(cinfo, st + 1)) break; 00355 st += 3; 00356 if (k >= cinfo->Se) { 00357 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00358 entropy->ct = -1; /* spectral overflow */ 00359 return TRUE; 00360 } 00361 } 00362 /* Figure F.21: Decoding nonzero value v */ 00363 /* Figure F.22: Decoding the sign of v */ 00364 sign = arith_decode(cinfo, entropy->fixed_bin); 00365 st += 2; 00366 /* Figure F.23: Decoding the magnitude category of v */ 00367 if ((m = arith_decode(cinfo, st)) != 0) { 00368 if (arith_decode(cinfo, st)) { 00369 m <<= 1; 00370 st = entropy->ac_stats[tbl] + 00371 (k <= cinfo->arith_ac_K[tbl] ? 189 : 217); 00372 while (arith_decode(cinfo, st)) { 00373 if ((m <<= 1) == 0x8000) { 00374 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00375 entropy->ct = -1; /* magnitude overflow */ 00376 return TRUE; 00377 } 00378 st += 1; 00379 } 00380 } 00381 } 00382 v = m; 00383 /* Figure F.24: Decoding the magnitude bit pattern of v */ 00384 st += 14; 00385 while (m >>= 1) 00386 if (arith_decode(cinfo, st)) v |= m; 00387 v += 1; if (sign) v = -v; 00388 /* Scale and output coefficient in natural (dezigzagged) order */ 00389 (*block)[natural_order[k]] = (JCOEF) (v << cinfo->Al); 00390 } while (k < cinfo->Se); 00391 00392 return TRUE; 00393 } 00394 00395 00396 /* 00397 * MCU decoding for DC successive approximation refinement scan. 00398 * Note: we assume such scans can be multi-component, 00399 * although the spec is not very clear on the point. 00400 */ 00401 00402 METHODDEF(boolean) 00403 decode_mcu_DC_refine (j_decompress_ptr cinfo, JBLOCKROW *MCU_data) 00404 { 00405 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00406 unsigned char *st; 00407 int p1, blkn; 00408 00409 /* Process restart marker if needed */ 00410 if (cinfo->restart_interval) { 00411 if (entropy->restarts_to_go == 0) 00412 process_restart(cinfo); 00413 entropy->restarts_to_go--; 00414 } 00415 00416 st = entropy->fixed_bin; /* use fixed probability estimation */ 00417 p1 = 1 << cinfo->Al; /* 1 in the bit position being coded */ 00418 00419 /* Outer loop handles each block in the MCU */ 00420 00421 for (blkn = 0; blkn < cinfo->blocks_in_MCU; blkn++) { 00422 /* Encoded data is simply the next bit of the two's-complement DC value */ 00423 if (arith_decode(cinfo, st)) 00424 MCU_data[blkn][0][0] |= p1; 00425 } 00426 00427 return TRUE; 00428 } 00429 00430 00431 /* 00432 * MCU decoding for AC successive approximation refinement scan. 00433 */ 00434 00435 METHODDEF(boolean) 00436 decode_mcu_AC_refine (j_decompress_ptr cinfo, JBLOCKROW *MCU_data) 00437 { 00438 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00439 JBLOCKROW block; 00440 JCOEFPTR thiscoef; 00441 unsigned char *st; 00442 int tbl, k, kex; 00443 int p1, m1; 00444 const int * natural_order; 00445 00446 /* Process restart marker if needed */ 00447 if (cinfo->restart_interval) { 00448 if (entropy->restarts_to_go == 0) 00449 process_restart(cinfo); 00450 entropy->restarts_to_go--; 00451 } 00452 00453 if (entropy->ct == -1) return TRUE; /* if error do nothing */ 00454 00455 natural_order = cinfo->natural_order; 00456 00457 /* There is always only one block per MCU */ 00458 block = MCU_data[0]; 00459 tbl = cinfo->cur_comp_info[0]->ac_tbl_no; 00460 00461 p1 = 1 << cinfo->Al; /* 1 in the bit position being coded */ 00462 m1 = (-1) << cinfo->Al; /* -1 in the bit position being coded */ 00463 00464 /* Establish EOBx (previous stage end-of-block) index */ 00465 kex = cinfo->Se; 00466 do { 00467 if ((*block)[natural_order[kex]]) break; 00468 } while (--kex); 00469 00470 k = cinfo->Ss - 1; 00471 do { 00472 st = entropy->ac_stats[tbl] + 3 * k; 00473 if (k >= kex) 00474 if (arith_decode(cinfo, st)) break; /* EOB flag */ 00475 for (;;) { 00476 thiscoef = *block + natural_order[++k]; 00477 if (*thiscoef) { /* previously nonzero coef */ 00478 if (arith_decode(cinfo, st + 2)) { 00479 if (*thiscoef < 0) 00480 *thiscoef += m1; 00481 else 00482 *thiscoef += p1; 00483 } 00484 break; 00485 } 00486 if (arith_decode(cinfo, st + 1)) { /* newly nonzero coef */ 00487 if (arith_decode(cinfo, entropy->fixed_bin)) 00488 *thiscoef = m1; 00489 else 00490 *thiscoef = p1; 00491 break; 00492 } 00493 st += 3; 00494 if (k >= cinfo->Se) { 00495 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00496 entropy->ct = -1; /* spectral overflow */ 00497 return TRUE; 00498 } 00499 } 00500 } while (k < cinfo->Se); 00501 00502 return TRUE; 00503 } 00504 00505 00506 /* 00507 * Decode one MCU's worth of arithmetic-compressed coefficients. 00508 */ 00509 00510 METHODDEF(boolean) 00511 decode_mcu (j_decompress_ptr cinfo, JBLOCKROW *MCU_data) 00512 { 00513 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00514 jpeg_component_info * compptr; 00515 JBLOCKROW block; 00516 unsigned char *st; 00517 int blkn, ci, tbl, sign, k; 00518 int v, m; 00519 const int * natural_order; 00520 00521 /* Process restart marker if needed */ 00522 if (cinfo->restart_interval) { 00523 if (entropy->restarts_to_go == 0) 00524 process_restart(cinfo); 00525 entropy->restarts_to_go--; 00526 } 00527 00528 if (entropy->ct == -1) return TRUE; /* if error do nothing */ 00529 00530 natural_order = cinfo->natural_order; 00531 00532 /* Outer loop handles each block in the MCU */ 00533 00534 for (blkn = 0; blkn < cinfo->blocks_in_MCU; blkn++) { 00535 block = MCU_data[blkn]; 00536 ci = cinfo->MCU_membership[blkn]; 00537 compptr = cinfo->cur_comp_info[ci]; 00538 00539 /* Sections F.2.4.1 & F.1.4.4.1: Decoding of DC coefficients */ 00540 00541 tbl = compptr->dc_tbl_no; 00542 00543 /* Table F.4: Point to statistics bin S0 for DC coefficient coding */ 00544 st = entropy->dc_stats[tbl] + entropy->dc_context[ci]; 00545 00546 /* Figure F.19: Decode_DC_DIFF */ 00547 if (arith_decode(cinfo, st) == 0) 00548 entropy->dc_context[ci] = 0; 00549 else { 00550 /* Figure F.21: Decoding nonzero value v */ 00551 /* Figure F.22: Decoding the sign of v */ 00552 sign = arith_decode(cinfo, st + 1); 00553 st += 2; st += sign; 00554 /* Figure F.23: Decoding the magnitude category of v */ 00555 if ((m = arith_decode(cinfo, st)) != 0) { 00556 st = entropy->dc_stats[tbl] + 20; /* Table F.4: X1 = 20 */ 00557 while (arith_decode(cinfo, st)) { 00558 if ((m <<= 1) == 0x8000) { 00559 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00560 entropy->ct = -1; /* magnitude overflow */ 00561 return TRUE; 00562 } 00563 st += 1; 00564 } 00565 } 00566 /* Section F.1.4.4.1.2: Establish dc_context conditioning category */ 00567 if (m < (int) ((1L << cinfo->arith_dc_L[tbl]) >> 1)) 00568 entropy->dc_context[ci] = 0; /* zero diff category */ 00569 else if (m > (int) ((1L << cinfo->arith_dc_U[tbl]) >> 1)) 00570 entropy->dc_context[ci] = 12 + (sign * 4); /* large diff category */ 00571 else 00572 entropy->dc_context[ci] = 4 + (sign * 4); /* small diff category */ 00573 v = m; 00574 /* Figure F.24: Decoding the magnitude bit pattern of v */ 00575 st += 14; 00576 while (m >>= 1) 00577 if (arith_decode(cinfo, st)) v |= m; 00578 v += 1; if (sign) v = -v; 00579 entropy->last_dc_val[ci] += v; 00580 } 00581 00582 (*block)[0] = (JCOEF) entropy->last_dc_val[ci]; 00583 00584 /* Sections F.2.4.2 & F.1.4.4.2: Decoding of AC coefficients */ 00585 00586 if (cinfo->lim_Se == 0) continue; 00587 tbl = compptr->ac_tbl_no; 00588 k = 0; 00589 00590 /* Figure F.20: Decode_AC_coefficients */ 00591 do { 00592 st = entropy->ac_stats[tbl] + 3 * k; 00593 if (arith_decode(cinfo, st)) break; /* EOB flag */ 00594 for (;;) { 00595 k++; 00596 if (arith_decode(cinfo, st + 1)) break; 00597 st += 3; 00598 if (k >= cinfo->lim_Se) { 00599 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00600 entropy->ct = -1; /* spectral overflow */ 00601 return TRUE; 00602 } 00603 } 00604 /* Figure F.21: Decoding nonzero value v */ 00605 /* Figure F.22: Decoding the sign of v */ 00606 sign = arith_decode(cinfo, entropy->fixed_bin); 00607 st += 2; 00608 /* Figure F.23: Decoding the magnitude category of v */ 00609 if ((m = arith_decode(cinfo, st)) != 0) { 00610 if (arith_decode(cinfo, st)) { 00611 m <<= 1; 00612 st = entropy->ac_stats[tbl] + 00613 (k <= cinfo->arith_ac_K[tbl] ? 189 : 217); 00614 while (arith_decode(cinfo, st)) { 00615 if ((m <<= 1) == 0x8000) { 00616 WARNMS(cinfo, JWRN_ARITH_BAD_CODE); 00617 entropy->ct = -1; /* magnitude overflow */ 00618 return TRUE; 00619 } 00620 st += 1; 00621 } 00622 } 00623 } 00624 v = m; 00625 /* Figure F.24: Decoding the magnitude bit pattern of v */ 00626 st += 14; 00627 while (m >>= 1) 00628 if (arith_decode(cinfo, st)) v |= m; 00629 v += 1; if (sign) v = -v; 00630 (*block)[natural_order[k]] = (JCOEF) v; 00631 } while (k < cinfo->lim_Se); 00632 } 00633 00634 return TRUE; 00635 } 00636 00637 00638 /* 00639 * Initialize for an arithmetic-compressed scan. 00640 */ 00641 00642 METHODDEF(void) 00643 start_pass (j_decompress_ptr cinfo) 00644 { 00645 arith_entropy_ptr entropy = (arith_entropy_ptr) cinfo->entropy; 00646 int ci, tbl; 00647 jpeg_component_info * compptr; 00648 00649 if (cinfo->progressive_mode) { 00650 /* Validate progressive scan parameters */ 00651 if (cinfo->Ss == 0) { 00652 if (cinfo->Se != 0) 00653 goto bad; 00654 } else { 00655 /* need not check Ss/Se < 0 since they came from unsigned bytes */ 00656 if (cinfo->Se < cinfo->Ss || cinfo->Se > cinfo->lim_Se) 00657 goto bad; 00658 /* AC scans may have only one component */ 00659 if (cinfo->comps_in_scan != 1) 00660 goto bad; 00661 } 00662 if (cinfo->Ah != 0) { 00663 /* Successive approximation refinement scan: must have Al = Ah-1. */ 00664 if (cinfo->Ah-1 != cinfo->Al) 00665 goto bad; 00666 } 00667 if (cinfo->Al > 13) { /* need not check for < 0 */ 00668 bad: 00669 ERREXIT4(cinfo, JERR_BAD_PROGRESSION, 00670 cinfo->Ss, cinfo->Se, cinfo->Ah, cinfo->Al); 00671 } 00672 /* Update progression status, and verify that scan order is legal. 00673 * Note that inter-scan inconsistencies are treated as warnings 00674 * not fatal errors ... not clear if this is right way to behave. 00675 */ 00676 for (ci = 0; ci < cinfo->comps_in_scan; ci++) { 00677 int coefi, cindex = cinfo->cur_comp_info[ci]->component_index; 00678 int *coef_bit_ptr = & cinfo->coef_bits[cindex][0]; 00679 if (cinfo->Ss && coef_bit_ptr[0] < 0) /* AC without prior DC scan */ 00680 WARNMS2(cinfo, JWRN_BOGUS_PROGRESSION, cindex, 0); 00681 for (coefi = cinfo->Ss; coefi <= cinfo->Se; coefi++) { 00682 int expected = (coef_bit_ptr[coefi] < 0) ? 0 : coef_bit_ptr[coefi]; 00683 if (cinfo->Ah != expected) 00684 WARNMS2(cinfo, JWRN_BOGUS_PROGRESSION, cindex, coefi); 00685 coef_bit_ptr[coefi] = cinfo->Al; 00686 } 00687 } 00688 /* Select MCU decoding routine */ 00689 if (cinfo->Ah == 0) { 00690 if (cinfo->Ss == 0) 00691 entropy->pub.decode_mcu = decode_mcu_DC_first; 00692 else 00693 entropy->pub.decode_mcu = decode_mcu_AC_first; 00694 } else { 00695 if (cinfo->Ss == 0) 00696 entropy->pub.decode_mcu = decode_mcu_DC_refine; 00697 else 00698 entropy->pub.decode_mcu = decode_mcu_AC_refine; 00699 } 00700 } else { 00701 /* Check that the scan parameters Ss, Se, Ah/Al are OK for sequential JPEG. 00702 * This ought to be an error condition, but we make it a warning. 00703 */ 00704 if (cinfo->Ss != 0 || cinfo->Ah != 0 || cinfo->Al != 0 || 00705 (cinfo->Se < DCTSIZE2 && cinfo->Se != cinfo->lim_Se)) 00706 WARNMS(cinfo, JWRN_NOT_SEQUENTIAL); 00707 /* Select MCU decoding routine */ 00708 entropy->pub.decode_mcu = decode_mcu; 00709 } 00710 00711 /* Allocate & initialize requested statistics areas */ 00712 for (ci = 0; ci < cinfo->comps_in_scan; ci++) { 00713 compptr = cinfo->cur_comp_info[ci]; 00714 if (! cinfo->progressive_mode || (cinfo->Ss == 0 && cinfo->Ah == 0)) { 00715 tbl = compptr->dc_tbl_no; 00716 if (tbl < 0 || tbl >= NUM_ARITH_TBLS) 00717 ERREXIT1(cinfo, JERR_NO_ARITH_TABLE, tbl); 00718 if (entropy->dc_stats[tbl] == NULL) 00719 entropy->dc_stats[tbl] = (unsigned char *) (*cinfo->mem->alloc_small) 00720 ((j_common_ptr) cinfo, JPOOL_IMAGE, DC_STAT_BINS); 00721 MEMZERO(entropy->dc_stats[tbl], DC_STAT_BINS); 00722 /* Initialize DC predictions to 0 */ 00723 entropy->last_dc_val[ci] = 0; 00724 entropy->dc_context[ci] = 0; 00725 } 00726 if ((! cinfo->progressive_mode && cinfo->lim_Se) || 00727 (cinfo->progressive_mode && cinfo->Ss)) { 00728 tbl = compptr->ac_tbl_no; 00729 if (tbl < 0 || tbl >= NUM_ARITH_TBLS) 00730 ERREXIT1(cinfo, JERR_NO_ARITH_TABLE, tbl); 00731 if (entropy->ac_stats[tbl] == NULL) 00732 entropy->ac_stats[tbl] = (unsigned char *) (*cinfo->mem->alloc_small) 00733 ((j_common_ptr) cinfo, JPOOL_IMAGE, AC_STAT_BINS); 00734 MEMZERO(entropy->ac_stats[tbl], AC_STAT_BINS); 00735 } 00736 } 00737 00738 /* Initialize arithmetic decoding variables */ 00739 entropy->c = 0; 00740 entropy->a = 0; 00741 entropy->ct = -16; /* force reading 2 initial bytes to fill C */ 00742 00743 /* Initialize restart counter */ 00744 entropy->restarts_to_go = cinfo->restart_interval; 00745 } 00746 00747 00748 /* 00749 * Finish up at the end of an arithmetic-compressed scan. 00750 */ 00751 00752 METHODDEF(void) 00753 finish_pass (j_decompress_ptr cinfo) 00754 { 00755 /* no work necessary here */ 00756 } 00757 00758 00759 /* 00760 * Module initialization routine for arithmetic entropy decoding. 00761 */ 00762 00763 GLOBAL(void) 00764 jinit_arith_decoder (j_decompress_ptr cinfo) 00765 { 00766 arith_entropy_ptr entropy; 00767 int i; 00768 00769 entropy = (arith_entropy_ptr) 00770 (*cinfo->mem->alloc_small) ((j_common_ptr) cinfo, JPOOL_IMAGE, 00771 SIZEOF(arith_entropy_decoder)); 00772 cinfo->entropy = &entropy->pub; 00773 entropy->pub.start_pass = start_pass; 00774 entropy->pub.finish_pass = finish_pass; 00775 00776 /* Mark tables unallocated */ 00777 for (i = 0; i < NUM_ARITH_TBLS; i++) { 00778 entropy->dc_stats[i] = NULL; 00779 entropy->ac_stats[i] = NULL; 00780 } 00781 00782 /* Initialize index for fixed probability estimation */ 00783 entropy->fixed_bin[0] = 113; 00784 00785 if (cinfo->progressive_mode) { 00786 /* Create progression status table */ 00787 int *coef_bit_ptr, ci; 00788 cinfo->coef_bits = (int (*)[DCTSIZE2]) 00789 (*cinfo->mem->alloc_small) ((j_common_ptr) cinfo, JPOOL_IMAGE, 00790 cinfo->num_components*DCTSIZE2*SIZEOF(int)); 00791 coef_bit_ptr = & cinfo->coef_bits[0][0]; 00792 for (ci = 0; ci < cinfo->num_components; ci++) 00793 for (i = 0; i < DCTSIZE2; i++) 00794 *coef_bit_ptr++ = -1; 00795 } 00796 }
Generated on Wed Jul 13 2022 18:56:09 by
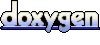