
Modify the file main.cpp for M487
Embed:
(wiki syntax)
Show/hide line numbers
ste_config.h
00001 /* 00002 * Copyright (c) 2017 Nuvoton Tecnology Corp. All rights reserved. 00003 * 00004 * Header for Serial-To-Ethernet configuration. 00005 * 00006 */ 00007 00008 #ifndef _STE_CONFIG_H 00009 #define _STE_CONFIG_H 00010 00011 #include "mbed.h" 00012 #include "EthernetInterface.h" 00013 #include "TCPSocket.h" 00014 #include "TCPServer.h" 00015 #include "BufferedSerial.h" 00016 #include "FATFileSystem.h" 00017 #include "NuSDBlockDevice.h" 00018 00019 00020 //#define ENABLE_WEB_CONFIG // Define this to active a simple web sever for 00021 // UART ports and Ethernet port parameters configuration. 00022 00023 /* Maximum UART ports supported */ 00024 #define MAX_UART_PORTS 2 00025 00026 /* Default UART baud */ 00027 #define DEFAULT_UART_BAUD 115200 00028 00029 /* Network base port number to listen. 00030 So the base port maps to the 1st UART port, 00031 the (base port + 1) maps to the 2nd UART port, etc. */ 00032 #define NET_PORT_BASE 10001 00033 00034 /* Path and Filename of configuration files */ 00035 #define SER_CONFIG_FILE "/fs/STE_SER.TXT" // for serial ports 00036 #define NET_CONFIG_FILE "/fs/STE_NET.TXT" // for network 00037 00038 /* Maximum size of server address */ 00039 #define MAX_SERVER_ADDRESS_SIZE 63 00040 00041 /* Maximum size of IP address */ 00042 #define MAX_IPV4_ADDRESS_SIZE 15 00043 00044 /* Functions and global variables declaration. */ 00045 00046 typedef enum { 00047 NET_SERVER_MODE = 0, 00048 NET_CLIENT_MODE 00049 } E_NetMode; 00050 00051 typedef enum { 00052 IP_STATIC_MODE = 0, 00053 IP_DHCP_MODE 00054 } E_IPMode; 00055 00056 typedef struct { 00057 E_IPMode mode; 00058 char ip[MAX_IPV4_ADDRESS_SIZE+1]; 00059 char mask[MAX_IPV4_ADDRESS_SIZE+1]; 00060 char gateway[MAX_IPV4_ADDRESS_SIZE+1]; 00061 } S_NET_CONFIG; 00062 00063 typedef struct { 00064 E_NetMode mode; // Network server or client mode 00065 int port; // Network port number 00066 BufferedSerial *pserial; // UART number 00067 int baud; // UART baud 00068 int data; // UART data bits 00069 int stop; // UART stop bits 00070 mbed::SerialBase::Parity parity; // UART parity bit 00071 char server_addr[MAX_SERVER_ADDRESS_SIZE+1]; // Server address for TCP client mode 00072 unsigned short server_port; // Server port for TCP client mode 00073 } S_PORT_CONFIG; 00074 00075 extern RawSerial output; // for debug output 00076 extern EthernetInterface eth; 00077 extern S_PORT_CONFIG port_config[MAX_UART_PORTS]; 00078 extern S_NET_CONFIG net_config; 00079 00080 extern bool SD_Card_Mounted; 00081 void start_httpd(void); 00082 00083 #endif
Generated on Sat Jul 23 2022 12:40:30 by
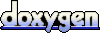