
This is a example application for StarBoard Orange designed by @logic_star. This example can be drive a CHORO Q HYBRID.
main.cpp
00001 /** 00002 * StarBoard Orange - Example application No.3 (Version 0.0.1) 00003 * Drive a CHORO Q HYBRID with wii nunchuk 00004 * 00005 * See also ... http://mbed.org/users/shintamainjp/notebook/starboard_example3_ja/ 00006 * See also ... http://mbed.org/users/shintamainjp/notebook/starboard_example3_en/ 00007 * 00008 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00009 * http://shinta.main.jp/ 00010 */ 00011 00012 /* 00013 * Connection map. 00014 * 00015 * +---+----------------+---------+ 00016 * |Pin|Target |Direction| 00017 * +---+----------------+---------+ 00018 * |p21|IR transmitter |OUT | 00019 * +---+----------------+---------+ 00020 */ 00021 00022 /* 00023 * Include files. 00024 */ 00025 00026 #include <mbed.h> 00027 #include <algorithm> 00028 #include <ChoroQ.h> 00029 #include <I2CConfig.h> 00030 #include <WiiNunchuckReader.h> 00031 #include <TextLCD.h> 00032 #include "appconf.h" 00033 00034 /* 00035 * Objects. 00036 */ 00037 00038 WiiNunchuckReader wn(I2CPort_A::SDA, I2CPort_A::SCL); 00039 ChoroQ cq(p21); 00040 TextLCD lcd(p24, p26, p27, p28, p29, p30); 00041 BusOut led(LED4, LED3, LED2, LED1); 00042 00043 /** 00044 * Display a splash screen. 00045 */ 00046 void splash(void) { 00047 lcd.cls(); 00048 lcd.locate(0, 0); 00049 lcd.printf("StarBoard Orange"); 00050 lcd.locate(0, 1); 00051 lcd.printf("mbed NXP LPC1768"); 00052 wait(3); 00053 00054 lcd.cls(); 00055 lcd.locate(0, 0); 00056 lcd.printf("Example app No.3"); 00057 lcd.locate(0, 1); 00058 lcd.printf(" CHORO Q HYBRID "); 00059 wait(3); 00060 } 00061 00062 /** 00063 * Get an action from a coordinate. 00064 * 00065 * @param x X axis. 00066 * @param y Y axis. 00067 * @param dash State of dash. 00068 * 00069 * @return An action. 00070 */ 00071 ChoroQ::Action getAction(const int x, const int y, bool dash) { 00072 static const int MAX_X = 200; 00073 static const int MIN_X = 20; 00074 static const int MAX_Y = 200; 00075 static const int MIN_Y = 20; 00076 int px = ((x - MIN_X) * 100) / (MAX_X - MIN_X); 00077 int py = ((y - MIN_Y) * 100) / (MAX_Y - MIN_Y); 00078 px = std::max(0, std::min(100, px)) - 50; // Range of a value is -50 to +50 00079 py = std::max(0, std::min(100, py)) - 50; // Range of a value is -50 to +50 00080 00081 if ((std::abs(px) <= 10) && (std::abs(py) <= 10)) { 00082 return ChoroQ::Stop; 00083 } 00084 00085 if ((x == 0) && (y == 0)) { 00086 return ChoroQ::Stop; 00087 } 00088 00089 if (std::abs(px) < 10) { 00090 if (py < 0) { 00091 if (dash) { 00092 return ChoroQ::DownDash; 00093 } else { 00094 return ChoroQ::Down; 00095 } 00096 } else { 00097 if (dash) { 00098 return ChoroQ::UpDash; 00099 } else { 00100 return ChoroQ::Up; 00101 } 00102 } 00103 } 00104 if (std::abs(py) < 10) { 00105 if (px < -20) { 00106 return ChoroQ::Left; 00107 } 00108 if (20 < px) { 00109 return ChoroQ::Right; 00110 } 00111 } 00112 if ((10 < px) && (10 < py)) { 00113 if (dash) { 00114 return ChoroQ::UpRightDash; 00115 } else { 00116 return ChoroQ::UpRight; 00117 } 00118 } 00119 if ((px < -10) && (10 < py)) { 00120 if (dash) { 00121 return ChoroQ::UpLeftDash; 00122 } else { 00123 return ChoroQ::UpLeft; 00124 } 00125 } 00126 if ((px < -10) && (py < -10)) { 00127 if (dash) { 00128 return ChoroQ::DownLeftDash; 00129 } else { 00130 return ChoroQ::DownLeft; 00131 } 00132 } 00133 if ((10 < px) && (py < -10)) { 00134 if (dash) { 00135 return ChoroQ::DownRightDash; 00136 } else { 00137 return ChoroQ::DownRight; 00138 } 00139 } 00140 return ChoroQ::Stop; 00141 } 00142 00143 /** 00144 * Entry point. 00145 */ 00146 int main() { 00147 /* 00148 * Splash. 00149 */ 00150 splash(); 00151 lcd.cls(); 00152 00153 /* 00154 * Setup a configuration. 00155 */ 00156 appconf_t conf; 00157 appconf_init(&conf); 00158 appconf_read(&conf); 00159 00160 /* 00161 * Application loop. 00162 */ 00163 while (true) { 00164 wn.RequestRead(); 00165 00166 ChoroQ::Action ac = getAction(wn.getJoyX(), wn.getJoyY(), (wn.getButtonZ() == 1) ? true : false); 00167 cq.execute(conf.channel, ac); 00168 00169 lcd.locate(0, 0); 00170 lcd.printf("JS :(%3d,%3d) %s", wn.getJoyX(), wn.getJoyY(), ((wn.getButtonZ() == 1) ? "D" : " ")); 00171 00172 char *stattext = ""; 00173 switch (ac) { 00174 case ChoroQ::Undef: 00175 stattext = "Undef"; 00176 break; 00177 case ChoroQ::Up: 00178 stattext = "Up"; 00179 break; 00180 case ChoroQ::Down: 00181 stattext = "Down"; 00182 break; 00183 case ChoroQ::Left: 00184 stattext = "Left"; 00185 break; 00186 case ChoroQ::Right: 00187 stattext = "Right"; 00188 break; 00189 case ChoroQ::UpDash: 00190 stattext = "Up +D"; 00191 break; 00192 case ChoroQ::UpLeft: 00193 stattext = "UpLeft"; 00194 break; 00195 case ChoroQ::UpRight: 00196 stattext = "UpRight"; 00197 break; 00198 case ChoroQ::UpRightDash: 00199 stattext = "UpRight +D"; 00200 break; 00201 case ChoroQ::UpLeftDash: 00202 stattext = "UpLeft +D"; 00203 break; 00204 case ChoroQ::DownLeft: 00205 stattext = "DownLeft"; 00206 break; 00207 case ChoroQ::DownRight: 00208 stattext = "DownRight"; 00209 break; 00210 case ChoroQ::DownDash: 00211 stattext = "Down +D"; 00212 break; 00213 case ChoroQ::DownLeftDash: 00214 stattext = "DownLeft +D"; 00215 break; 00216 case ChoroQ::DownRightDash: 00217 stattext = "DownRight+D"; 00218 break; 00219 case ChoroQ::Stop: 00220 stattext = "Stop"; 00221 break; 00222 } 00223 lcd.locate(0, 1); 00224 lcd.printf("Ch.%c:%-11.11s", 'A' + (int)conf.channel, stattext); 00225 00226 wait_ms(50); 00227 led = led + 1; 00228 } 00229 }
Generated on Tue Jul 12 2022 22:53:27 by
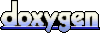