
This is a example application for StarBoard Orange designed by @logic_star. This example can be drive a CHORO Q HYBRID.
WiiNunchuckReader.h
00001 /* 00002 * WiiNunchuckReader. A program allowing the output of one or two 00003 * Wii Nunchucks to be read via I2C and decoded for use, using the mbed 00004 * microcontroller and its associated libraries. 00005 * 00006 * Copyright (C) <2009> Petras Saduikis <petras@petras.co.uk> 00007 * 00008 * This file is part of WiiNunchuckReader. 00009 * 00010 * WiiNunchuckReader is free software: you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation, either version 3 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * WiiNunchuckReader is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License 00021 * along with WiiNunchuckReader. If not, see <http://www.gnu.org/licenses/>. 00022 */ 00023 00024 #ifndef SNATCH59_WIINUNCHUCKREADER_H 00025 #define SNATCH59_WIINUNCHUCKREADER_H 00026 00027 #include <mbed.h> 00028 #include "WiiNunchuckDefs.h" 00029 00030 typedef unsigned char BYTE; 00031 00032 class WiiNunchuckReader { 00033 public: 00034 // constructors 00035 WiiNunchuckReader(PinName sda, PinName scl); 00036 00037 // functions 00038 void RequestRead(); 00039 00040 // accessors 00041 int getJoyX() const { 00042 return joyX; 00043 } 00044 int getJoyY() const { 00045 return joyY; 00046 } 00047 int getAccelX() const { 00048 return accelX; 00049 } 00050 int getAccelY() const { 00051 return accelY; 00052 } 00053 int getAccelZ() const { 00054 return accelZ; 00055 } 00056 int getButtonC() const { 00057 return buttonC; 00058 } 00059 int getButtonZ() const { 00060 return buttonZ; 00061 } 00062 int getBufferSize() const { 00063 return sizeof(readBuf); 00064 } 00065 char* getReadBuf() { 00066 return readBuf; 00067 } 00068 00069 private: 00070 // nunchuck controls states 00071 int joyX; 00072 int joyY; 00073 int accelX; 00074 int accelY; 00075 int accelZ; 00076 int buttonC; 00077 int buttonZ; 00078 00079 // nunchuck init state 00080 bool nunchuckInit; 00081 00082 // nunchuck I2C port 00083 I2C nunchuckPort; 00084 00085 // read data 00086 char readBuf[NUNCHUCK_READLEN]; 00087 00088 // functions 00089 bool NunchuckInit(); 00090 bool NunchuckRead(); 00091 void NunchuckDecode(); 00092 void DecodeAdditional(); 00093 }; 00094 00095 #endif
Generated on Tue Jul 12 2022 22:53:27 by
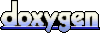