
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Test program. 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 #include "mbed.h" 00009 #include "SG12864A.h" 00010 00011 int main() { 00012 00013 SG12864A lcd(p5, p6, p7, p8, p9, p10, p11, p12, p13, p14, p15, p16, p17, p18); 00014 #if 0 00015 lcd.clear(); 00016 lcd.reset(); 00017 00018 { 00019 static const int pages = 8; 00020 static const int columns = 64; 00021 for (int i = 0; i < pages; i++) { 00022 lcd.setPageAddress(SG12864A::CS1, i); 00023 lcd.setPageAddress(SG12864A::CS2, i); 00024 for (int j = 0; j < columns; j++) { 00025 uint8_t n = 1 << (j % 8); 00026 lcd.setColumnAddress(SG12864A::CS1, j); 00027 lcd.setColumnAddress(SG12864A::CS2, j); 00028 lcd.writeData(SG12864A::CS1, n); 00029 lcd.writeData(SG12864A::CS2, n); 00030 } 00031 } 00032 } 00033 00034 uint8_t n = 0; 00035 while (1) { 00036 lcd.setDisplayStartLine(SG12864A::CS1, n); 00037 lcd.setDisplayStartLine(SG12864A::CS2, n); 00038 n++; 00039 wait_ms(100); 00040 } 00041 #else 00042 lcd.clear(); 00043 lcd.reset(); 00044 bool reverse = false; 00045 while (1) { 00046 // 00047 lcd.bufferClear(reverse); 00048 lcd.bufferDrawLine(0, 0, SG12864A::PIXEL_X - 1, SG12864A::PIXEL_Y - 1, reverse); 00049 lcd.bufferDrawLine(0, SG12864A::PIXEL_Y - 1, SG12864A::PIXEL_X - 1, 0, reverse); 00050 lcd.bufferDrawString(1, 1, "This is a test #1.\b", reverse); 00051 lcd.bufferDrawCheckbox(50, 50, 60, 60, true, reverse); 00052 lcd.bufferPush(); 00053 wait_ms(1000); 00054 00055 // 00056 lcd.bufferClear(reverse); 00057 lcd.bufferDrawBox(0, 0, SG12864A::PIXEL_X - 1, SG12864A::PIXEL_Y - 1, reverse); 00058 lcd.bufferDrawString(1, 2, "This is a test #2.\b", reverse); 00059 lcd.bufferDrawCheckbox(50, 50, 60, 60, false, reverse); 00060 lcd.bufferPush(); 00061 wait_ms(1000); 00062 00063 #if 0 00064 // 00065 lcd.bufferClear(reverse); 00066 lcd.bufferFillBox(0, 0, SG12864A::PIXEL_X - 1, SG12864A::PIXEL_Y - 1, reverse); 00067 lcd.bufferDrawString(1, 3, "This is a test #3.\b", reverse); 00068 lcd.bufferDrawCheckbox(50, 50, 60, 60, true, reverse); 00069 lcd.bufferPush(); 00070 wait_ms(1000); 00071 #endif 00072 00073 // 00074 { 00075 lcd.bufferClear(reverse); 00076 int min = 0; 00077 int max = 100; 00078 for (int i = min; i <= max; i++) { 00079 lcd.bufferDrawProgressbar(10, 10, 118, 20, min, max, i, reverse); 00080 lcd.bufferPush(); 00081 wait_ms(2); 00082 } 00083 } 00084 00085 // 00086 { 00087 lcd.bufferClear(reverse); 00088 int min = -100; 00089 int max = 100; 00090 for (int i = min; i <= max; i++) { 00091 lcd.bufferDrawProgressbar(10, 10, 118, 20, min, max, i, reverse); 00092 lcd.bufferPush(); 00093 wait_ms(2); 00094 } 00095 } 00096 00097 // 00098 { 00099 lcd.bufferClear(reverse); 00100 int min = 100; 00101 int max = 600; 00102 for (int i = min; i <= max; i++) { 00103 lcd.bufferDrawProgressbar(10, 10, 118, 20, min, max, i, reverse); 00104 lcd.bufferPush(); 00105 wait_ms(2); 00106 } 00107 } 00108 00109 // 00110 reverse = !reverse; 00111 } 00112 #endif 00113 }
Generated on Tue Jul 19 2022 05:32:29 by
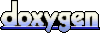