
This is a test program for FirmwareUpdater.
Dependencies: mbed FirmwareUpdater EthernetNetIf
main.cpp
00001 /** 00002 * ============================================================================= 00003 * A test program for firmware updater (Version 0.0.2) 00004 * http://mbed.org/users/shintamainjp/notebook/firmwareupdater_en/ 00005 * ============================================================================= 00006 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * ============================================================================= 00026 */ 00027 00028 #include "mbed.h" 00029 #include "FirmwareUpdater.h" 00030 #include "EthernetNetIf.h" 00031 00032 #define APPLICATION_NAME "firm" 00033 #define SERVER_VERSION 0 00034 00035 EthernetNetIf eth; 00036 FirmwareUpdater fwup("http://mbed.org/media/uploads/shintamainjp/", APPLICATION_NAME, true); 00037 BusOut led(LED4, LED3, LED2, LED1); 00038 Ticker ticker; 00039 00040 // [On a server] 00041 // 1. firm.txt : firmware version file. 00042 // 2. firm.bin : firmware binary file. 00043 // 00044 // [On a mbed] 00045 // 1. firm.txt : firmware version file. 00046 // 2. firm.bin : firmware binary file. 00047 00048 /** 00049 * LED function Type-1. 00050 */ 00051 void tick_func1() { 00052 led = led + 1; 00053 } 00054 00055 /** 00056 * LED function Type-2. 00057 */ 00058 void tick_func2() { 00059 led = led - 1; 00060 } 00061 00062 /** 00063 * Entry point. 00064 */ 00065 int main() { 00066 eth.setup(); 00067 00068 #if SERVER_VERSION 00069 printf("[%s: Server version.]\n", APPLICATION_NAME); 00070 ticker.attach_us(&tick_func1, 200 * 1000); 00071 #else 00072 printf("[%s: Local version.]\n", APPLICATION_NAME); 00073 ticker.attach_us(&tick_func2, 200 * 1000); 00074 #endif 00075 00076 const int a = fwup.exist(); 00077 if (a == 0) { 00078 printf("Found a new firmware.\n"); 00079 const int b = fwup.execute(); 00080 if (b == 0) { 00081 printf("Update succeed.\n"); 00082 printf("Resetting this system...\n\n\n\n\n"); 00083 fwup.reset(); 00084 } else { 00085 printf("Failed execute() [Code=%d]\n", b); 00086 } 00087 } else { 00088 if (a < 0) { 00089 printf("Failed exist() [Code=%d]\n", a); 00090 } else { 00091 printf("Up to date.\n"); 00092 } 00093 } 00094 }
Generated on Mon Jul 18 2022 10:42:43 by
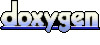