
LinkSprite JPEG Color Camera Test Program.
Dependencies: mbed Camera_LS_Y201 SDFileSystem
main.cpp
00001 /** 00002 * ============================================================================= 00003 * LS-Y201 - Test program. (Version 0.0.2) 00004 * ============================================================================= 00005 * Copyright (c) 2010-2011 Shinichiro Nakamura (CuBeatSystems) 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, including without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 * ============================================================================= 00025 */ 00026 #include "mbed.h" 00027 #include "Camera_LS_Y201.h" 00028 #include "SDFileSystem.h" 00029 00030 #define DEBMSG printf 00031 #define NEWLINE() printf("\r\n") 00032 00033 #define USE_SDCARD 0 00034 00035 #if USE_SDCARD 00036 #define FILENAME "/sd/IMG_%04d.jpg" 00037 SDFileSystem fs(p5, p6, p7, p8, "sd"); 00038 #else 00039 #define FILENAME "/local/IMG_%04d.jpg" 00040 LocalFileSystem fs("local"); 00041 #endif 00042 Camera_LS_Y201 cam1(p13, p14); 00043 00044 typedef struct work { 00045 FILE *fp; 00046 } work_t; 00047 00048 work_t work; 00049 00050 /** 00051 * Callback function for readJpegFileContent. 00052 * 00053 * @param buf A pointer to a buffer. 00054 * @param siz A size of the buffer. 00055 */ 00056 void callback_func(int done, int total, uint8_t *buf, size_t siz) { 00057 fwrite(buf, siz, 1, work.fp); 00058 00059 static int n = 0; 00060 int tmp = done * 100 / total; 00061 if (n != tmp) { 00062 n = tmp; 00063 DEBMSG("Writing...: %3d%%", n); 00064 NEWLINE(); 00065 } 00066 } 00067 00068 /** 00069 * Capture. 00070 * 00071 * @param cam A pointer to a camera object. 00072 * @param filename The file name. 00073 * 00074 * @return Return 0 if it succeed. 00075 */ 00076 int capture(Camera_LS_Y201 *cam, char *filename) { 00077 /* 00078 * Take a picture. 00079 */ 00080 if (cam->takePicture() != 0) { 00081 return -1; 00082 } 00083 DEBMSG("Captured."); 00084 NEWLINE(); 00085 00086 /* 00087 * Open file. 00088 */ 00089 work.fp = fopen(filename, "wb"); 00090 if (work.fp == NULL) { 00091 return -2; 00092 } 00093 00094 /* 00095 * Read the content. 00096 */ 00097 DEBMSG("%s", filename); 00098 NEWLINE(); 00099 if (cam->readJpegFileContent(callback_func) != 0) { 00100 fclose(work.fp); 00101 return -3; 00102 } 00103 fclose(work.fp); 00104 00105 /* 00106 * Stop taking pictures. 00107 */ 00108 cam->stopTakingPictures(); 00109 00110 return 0; 00111 } 00112 00113 /** 00114 * Entry point. 00115 */ 00116 int main(void) { 00117 DEBMSG("Camera module"); 00118 NEWLINE(); 00119 DEBMSG("Resetting..."); 00120 NEWLINE(); 00121 wait(1); 00122 00123 if (cam1.reset() == 0) { 00124 DEBMSG("Reset OK."); 00125 NEWLINE(); 00126 } else { 00127 DEBMSG("Reset fail."); 00128 NEWLINE(); 00129 error("Reset fail."); 00130 } 00131 wait(1); 00132 00133 int cnt = 0; 00134 while (1) { 00135 char fname[64]; 00136 snprintf(fname, sizeof(fname) - 1, FILENAME, cnt); 00137 int r = capture(&cam1, fname); 00138 if (r == 0) { 00139 DEBMSG("[%04d]:OK.", cnt); 00140 NEWLINE(); 00141 } else { 00142 DEBMSG("[%04d]:NG. (code=%d)", cnt, r); 00143 NEWLINE(); 00144 error("Failure."); 00145 } 00146 cnt++; 00147 } 00148 }
Generated on Thu Jul 14 2022 12:44:42 by
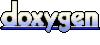